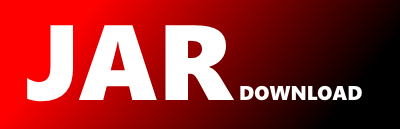
com.jelastic.api.users.response.AuthenticationResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 0f322d863777e8bf660c864b8effb297*/
package com.jelastic.api.users.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import com.jelastic.api.core.persistence.entity.AuthConfig;
import com.jelastic.api.core.persistence.entity.User;
import com.jelastic.api.core.persistence.model.UserStatus;
import com.jelastic.api.data.enumerations.AccessType;
import com.jelastic.api.data.enumerations.AuthType;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class AuthenticationResponse extends Response implements JSONSerializable {
public static final String DATA_LANGUAGE_KEY = "lang";
public static final String NAME = "name";
public static final String EMAIL = "email";
public static final String SESSION = "session";
public static final String UID = "uid";
public static final String ATTEMPTS_LEFT = "attemptsLeft";
public static final String DATA = "data";
private static final String AUTH_TYPE = "type";
private static final String AUTH_CONFIG = "authConfig";
private static final String REQUIRED_AUTH_CHECK = "requiredCheck";
private static final String ACCESS_TYPE = "accessType";
public static final String STATUS = "status";
public static final String IS_TENANT_HOST = "isTenantHost";
public static final String FEDERATION_TYPE = "federationType";
public static final String DEFAULT_TEAM_OWNER_UID = "defaultTeamOwnerUid";
public static final String RESELLER_ID = "resellerId";
public static final String IS_RESELLER_OWNER = "isResellerOwner";
private String session = null, name = null, email = null;
private Map data = null;
private int uid = -1;
private UserStatus status = null;
private int attemptsLeft = -1;
private AuthType authType = AuthType.BASIC;
private AuthConfig requiredAuthCheck;
private AccessType accessType = AccessType.FULL_ACCESS;
private boolean isTenantHost = false;
private String federationType;
private Integer defaultTeamOwnerUid;
private Integer resellerId;
private boolean isResellerOwner;
public AuthenticationResponse() {
super(Response.OK);
}
public AuthenticationResponse(User user) {
super(Response.OK);
setUid(user.getId());
setName(user.getName());
setEmail(user.getEmail());
setData(user.getData());
setStatus(user.getStatus());
setAuthType(user.getAuthType());
setTenantHost(user.isTenantHost());
setFederationType(user.getFederationType());
if (user.getDefaultTeamOwner() != null) {
setDefaultTeamOwnerUid(user.getDefaultTeamOwner().getId());
}
}
public AuthenticationResponse(Response resp) {
this.setResult(resp.getResult());
this.setError(resp.getError());
}
public AuthenticationResponse(int uid) {
super(Response.OK);
this.uid = uid;
}
public AuthenticationResponse(String session) {
super(Response.OK);
this.session = session;
}
public AuthenticationResponse(int result, String error) {
super(result, error);
}
public String getSession() {
return session;
}
public void setSession(Object session) {
setSession(session == null ? null : (String) session);
}
public void setSession(String session) {
this.session = session;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Map getData() {
return data;
}
public void setData(Map data) {
this.data = data;
}
public UserStatus getStatus() {
return status;
}
public void setStatus(UserStatus status) {
this.status = status;
}
public int getAttemptsLeft() {
return attemptsLeft;
}
public void setAttemptsLeft(int attemptsLeft) {
this.attemptsLeft = attemptsLeft;
}
public AuthType getAuthType() {
return authType;
}
public void setAuthType(AuthType authType) {
this.authType = authType;
}
public AuthConfig getRequiredAuthCheck() {
return requiredAuthCheck;
}
public void setRequiredAuthCheck(AuthConfig requiredAuthCheck) {
this.requiredAuthCheck = requiredAuthCheck;
}
public AccessType getAccessType() {
return accessType;
}
public void setAccessType(AccessType accessType) {
this.accessType = accessType;
}
public boolean isTenantHost() {
return isTenantHost;
}
public void setTenantHost(boolean tenantHost) {
isTenantHost = tenantHost;
}
public String getFederationType() {
return federationType;
}
public void setFederationType(String federationType) {
this.federationType = federationType;
}
public Integer getDefaultTeamOwnerUid() {
return defaultTeamOwnerUid;
}
public void setDefaultTeamOwnerUid(Integer defaultTeamOwnerUid) {
this.defaultTeamOwnerUid = defaultTeamOwnerUid;
}
public Integer getResellerId() {
return resellerId;
}
public void setResellerId(Integer resellerId) {
this.resellerId = resellerId;
}
public boolean isResellerOwner() {
return isResellerOwner;
}
public void setResellerOwner(boolean resellerOwner) {
isResellerOwner = resellerOwner;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (getName() != null) {
json.put(NAME, getName());
}
if (getEmail() != null) {
json.put(EMAIL, getEmail());
}
if (getSession() != null) {
json.put(SESSION, getSession());
}
if (getData() != null) {
json.put(DATA, getData());
}
if (getUid() > -1) {
json.put(UID, getUid());
}
if (getStatus() != null) {
json.put(STATUS, getStatus());
}
if (getAttemptsLeft() > -1) {
json.put(ATTEMPTS_LEFT, getAttemptsLeft());
}
if (getFederationType() != null) {
json.put(FEDERATION_TYPE, getFederationType());
}
if (getDefaultTeamOwnerUid() != null) {
json.put(DEFAULT_TEAM_OWNER_UID, getDefaultTeamOwnerUid());
}
if (getResellerId() != null) {
json.put(RESELLER_ID, getResellerId());
}
if (result <= Response.OK) {
JSONObject authConfigJson = new JSONObject();
authConfigJson.put(AUTH_TYPE, authType);
if (requiredAuthCheck != null && !requiredAuthCheck.isTemporary()) {
authConfigJson.put(REQUIRED_AUTH_CHECK, getRequiredAuthCheck()._toJSON());
}
if (authConfigJson.length() > 0) {
json.put(AUTH_CONFIG, authConfigJson);
}
json.put(ACCESS_TYPE, getAccessType());
}
json.put(IS_TENANT_HOST, this.isTenantHost);
json.put(IS_RESELLER_OWNER, this.isResellerOwner);
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public AuthenticationResponse fromJSON(JSONObject json) {
try {
super.fromJSON(json);
if (json.has(NAME)) {
this.name = json.getString(NAME);
}
if (json.has(EMAIL)) {
this.email = json.getString(EMAIL);
}
if (json.has(SESSION)) {
this.session = json.getString(SESSION);
}
if (json.has(UID)) {
this.uid = json.getInt(UID);
}
if (json.has(ATTEMPTS_LEFT)) {
this.attemptsLeft = json.getInt(ATTEMPTS_LEFT);
}
if (json.has(ACCESS_TYPE)) {
this.accessType = AccessType.valueOf(json.getString(ACCESS_TYPE).toUpperCase());
}
if (json.has(STATUS)) {
this.status = UserStatus.valueOf(UserStatus.class, json.getString(STATUS));
}
if (json.has(AUTH_CONFIG)) {
JSONObject authConfigJson = json.getJSONObject(AUTH_CONFIG);
if (authConfigJson.has(AUTH_TYPE)) {
authType = AuthType.get(authConfigJson.getString(AUTH_TYPE));
}
if (authConfigJson.has(REQUIRED_AUTH_CHECK)) {
requiredAuthCheck = new AuthConfig().fromJSON(authConfigJson.getJSONObject(REQUIRED_AUTH_CHECK));
}
}
if (json.has(DATA)) {
JSONObject jsonData = json.getJSONObject(DATA);
data = new HashMap<>();
if (jsonData.has(DATA_LANGUAGE_KEY)) {
data.put(DATA_LANGUAGE_KEY, jsonData.getString(DATA_LANGUAGE_KEY));
}
}
if (json.has(IS_TENANT_HOST)) {
this.isTenantHost = json.getBoolean(IS_TENANT_HOST);
}
if (json.has(FEDERATION_TYPE)) {
this.federationType = json.getString(FEDERATION_TYPE);
}
if (json.has(RESELLER_ID)) {
this.resellerId = json.getInt(RESELLER_ID);
}
if (json.has(IS_RESELLER_OWNER)) {
this.isResellerOwner = json.getBoolean(IS_RESELLER_OWNER);
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy