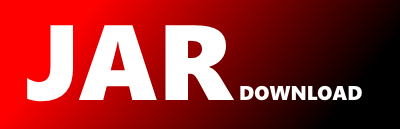
com.jelastic.api.users.response.SSHKeyResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 81ae55ce51a97d15876da1176986ce4f*/
package com.jelastic.api.users.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import com.jelastic.api.core.persistence.entity.SSHKey;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.List;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SSHKeyResponse extends Response implements JSONSerializable {
public static final String RESULT = "result";
public static final String ERROR = "error";
public static final String KEY = "key";
public static final String KEYS = "keys";
public static final String DEPENDENCIES = "dependencies";
private List keys;
private SSHKey key;
private JSONArray dependencies;
public SSHKeyResponse() {
super(Response.OK);
}
public SSHKeyResponse(Response resp) {
this.setResult(resp.getResult());
this.setError(resp.getError());
}
public SSHKeyResponse(int result, String error) {
super(result, error);
}
public SSHKeyResponse(SSHKey key) {
super(Response.OK);
this.key = key;
}
public SSHKeyResponse(List keys) {
super(Response.OK);
this.keys = keys;
}
public List getKeys() {
return keys;
}
public void setKeys(List keys) {
this.keys = keys;
}
public SSHKey getKey() {
return key;
}
public void setKey(SSHKey key) {
this.key = key;
}
public JSONArray getDependencies() {
return dependencies;
}
public void setDependencies(JSONArray dependencies) {
this.dependencies = dependencies;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
try {
if (key != null) {
json.put(KEY, key._toJSON());
}
if (keys != null) {
JSONArray keysJson = new JSONArray();
for (SSHKey key : keys) {
keysJson.put(key._toJSON());
}
json.put(KEYS, keysJson);
}
json.put(DEPENDENCIES, dependencies);
} catch (Exception e) {
e.printStackTrace();
try {
json.put(RESULT, Response.ERROR_UNKNOWN).put(ERROR, e.toString());
} catch (JSONException ex) {
}
}
return json;
}
@Override
public SSHKeyResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(KEY)) {
this.key = new SSHKey()._fromJSON(json.getJSONObject(KEY));
}
if (json.has(KEYS)) {
JSONArray keysJson = json.getJSONArray(KEYS);
keys = new ArrayList();
for (int i = 0; i < keysJson.length(); i++) {
JSONObject key = keysJson.getJSONObject(i);
keys.add(new SSHKey()._fromJSON(key));
}
}
if (json.has(DEPENDENCIES)) {
dependencies = json.getJSONArray(DEPENDENCIES);
}
return this;
}
public SSHKeyResponse fromJSON(JSONObject json) {
try {
return _fromJSON(json);
} catch (JSONException ex) {
error = ex.toString();
result = ERROR_UNKNOWN;
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy