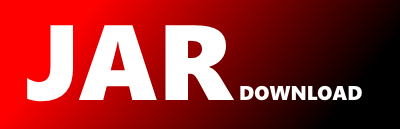
com.jelastic.api.users.response.SessionsResponse Maven / Gradle / Ivy
/*Server class MD5: 136e51587392ca22817668bc11eb1056*/
package com.jelastic.api.users.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import com.jelastic.api.users.session.dto.SessionDTO;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SessionsResponse extends Response implements JSONSerializable {
private static final String SESSIONS = "sessions";
private Set sessions;
public SessionsResponse() {
super(Response.OK);
}
public SessionsResponse(Set sessions) {
super(Response.OK);
this.sessions = sessions;
}
public SessionsResponse(int result, String error) {
super(result, error);
}
public SessionsResponse(Response resp) {
this.setResult(resp.getResult());
this.setError(resp.getError());
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (sessions != null) {
JSONArray jsonArray = new JSONArray();
for (SessionDTO session : sessions) {
jsonArray.put(session.toJSON());
}
json.put(SESSIONS, jsonArray);
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public SessionsResponse fromJSON(JSONObject json) {
try {
if (json.has(SESSIONS) && !json.isNull(SESSIONS)) {
JSONArray jsonArray = json.getJSONArray(SESSIONS);
if (sessions == null) {
sessions = new HashSet<>(jsonArray.length());
}
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
SessionDTO sessionDTO = new SessionDTO();
sessionDTO.fromJSON(jsonObject);
sessions.add(sessionDTO);
}
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy