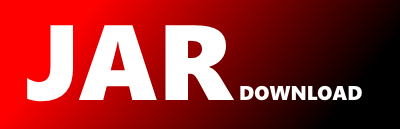
com.jelastic.api.users.response.TwoFAConfigResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.users.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import org.json.JSONException;
import org.json.JSONObject;
/**
*
* @author Maksym Shevchuk
*/
public class TwoFAConfigResponse extends Response implements JSONSerializable {
private static final String SECRET_KEY = "secretKey";
private static final String ACCOUNT = "account";
private static final String SECRET_KEY_URI = "secretKeyUri";
private static final String ISSUER = "issuer";
private String secretKey;
private String account;
private String secretKeyUri;
private String issuer;
public TwoFAConfigResponse() {
super(Response.OK);
}
public TwoFAConfigResponse(String secretKey, String account, String secretKeyUri, String issuer) {
super(Response.OK);
this.secretKey = secretKey;
this.account = account;
this.secretKeyUri = secretKeyUri;
this.issuer = issuer;
}
public TwoFAConfigResponse(int result, String error) {
super(result, error);
}
public String getSecretKey() {
return secretKey;
}
public void setSecretKey(String secretKey) {
this.secretKey = secretKey;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getSecretKeyUri() {
return secretKeyUri;
}
public void setSecretKeyUri(String secretKeyUri) {
this.secretKeyUri = secretKeyUri;
}
public String getIssuer() {
return issuer;
}
public void setIssuer(String issuer) {
this.issuer = issuer;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
json.put(SECRET_KEY, getSecretKey());
json.put(ACCOUNT, getAccount());
json.put(SECRET_KEY_URI, getSecretKeyUri());
json.put(ISSUER, getIssuer());
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy