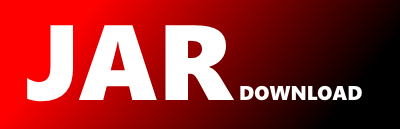
com.jelastic.api.users.session.dto.SessionDTO Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 2b7ad15e1122252e2e821536a6279ebd*/
package com.jelastic.api.users.session.dto;
import com.jelastic.api.json.JSONDeserializable;
import com.jelastic.api.json.JSONSerializable;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @author Maksym Shevchuk
*/
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class SessionDTO implements JSONDeserializable, JSONSerializable {
private static final String ID = "id";
private static final String USER_AGENT = "userAgent";
private static final String HOST = "host";
private static final String UID = "uid";
private static final String CURRENT = "current";
private static final String CREATED_ON = "createdOn";
private static final String LAST_ACCESS = "lastAccess";
private int id;
private String userAgent;
private String host;
private int uid;
private boolean current;
private String createdOn;
private String lastAccess;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUserAgent() {
return userAgent;
}
public void setUserAgent(String userAgent) {
this.userAgent = userAgent;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public boolean isCurrent() {
return current;
}
public void setCurrent(boolean current) {
this.current = current;
}
public String getCreatedOn() {
return createdOn;
}
public void setCreatedOn(String createdOn) {
this.createdOn = createdOn;
}
public String getLastAccess() {
return lastAccess;
}
public void setLastAccess(String lastAccess) {
this.lastAccess = lastAccess;
}
@Override
public Object fromJSON(JSONObject json) {
try {
if (json.has(ID)) {
this.id = json.getInt(ID);
}
if (json.has(USER_AGENT)) {
this.userAgent = json.getString(USER_AGENT);
}
if (json.has(HOST)) {
this.host = json.getString(HOST);
}
if (json.has(UID)) {
this.uid = json.getInt(UID);
}
if (json.has(CURRENT)) {
this.current = json.getBoolean(CURRENT);
}
if (json.has(CREATED_ON)) {
this.createdOn = json.getString(CREATED_ON);
}
if (json.has(LAST_ACCESS)) {
this.lastAccess = json.getString(LAST_ACCESS);
}
} catch (JSONException e) {
e.printStackTrace();
}
return this;
}
@Override
public Object fromString(String str) {
try {
return fromJSON(new JSONObject(str));
} catch (JSONException e) {
e.printStackTrace();
}
return null;
}
@Override
public JSONObject toJSON() {
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put(ID, id);
jsonObject.put(USER_AGENT, userAgent);
jsonObject.put(HOST, host);
jsonObject.put(UID, uid);
jsonObject.put(CURRENT, current);
jsonObject.put(CREATED_ON, createdOn);
jsonObject.put(LAST_ACCESS, lastAccess);
} catch (JSONException e) {
e.printStackTrace();
}
return jsonObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy