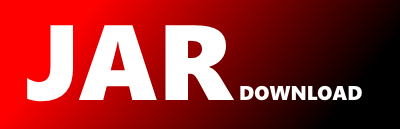
com.jelastic.api.utils.IpV4 Maven / Gradle / Ivy
/*Server class MD5: f1d304adb6a8833931ecb51ab0b92b03*/
package com.jelastic.api.utils;
import java.util.StringTokenizer;
import java.util.regex.Pattern;
import com.jelastic.api.core.utils.StringUtils;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class IpV4 implements Comparable {
public static String SEPARATOR = ".";
public static String MASK_16 = "16";
public static String SUBNET_16_SUFFIX = "0.0/";
static public final String IPV4_REGEX = "(([0-1]?[0-9]{1,2}\\.)|(2[0-4][0-9]\\.)|(25[0-5]\\.)){3}(([0-1]?[0-9]{1,2})|(2[0-4][0-9])|(25[0-5]))";
private static final String IPV4_SUBNET_REGEX = "(([0-1]?[0-9]{1,2}\\.)|(2[0-4][0-9]\\.)|(25[0-5]\\.)){3}(([0-1]?[0-9]{1,2})|(2[0-4][0-9])|(25[0-5]))/([1-9]|[1-2][0-9]|3[0-2])";
private static final Pattern IPV4_SUBNET_PATTERN = Pattern.compile(IPV4_SUBNET_REGEX);
private static final Pattern IPV4_PATTERN = Pattern.compile(IPV4_REGEX);
private int[] octets;
private String ipaddr = "";
public static boolean isIpV4Address(String address) {
if (StringUtils.isEmpty(address)) {
return false;
}
try {
new IpV4(address);
return true;
} catch (Exception e) {
return false;
}
}
public IpV4(String ipaddr) {
parse(ipaddr);
}
public IpV4(int[] octets) {
this.octets = octets;
octetsToIpaddr();
}
public static boolean isValidIPV4(final String s) {
return IPV4_PATTERN.matcher(s).matches();
}
public static boolean isValidIPV4Subnet(final String s) {
return IPV4_SUBNET_PATTERN.matcher(s).matches();
}
public static boolean isInRange(String startIp, String endIp, String checkIp) {
return isInRange(new IpV4(startIp), new IpV4(endIp), new IpV4(checkIp));
}
public static boolean isInRange(IpV4 startIp, IpV4 endIp, IpV4 checkIp) {
if (checkIp.compareTo(startIp) < 0 || checkIp.compareTo(endIp) > 0) {
return false;
}
return true;
}
private void octetsToIpaddr() {
for (int i : octets) {
this.ipaddr += SEPARATOR + i;
}
this.ipaddr = this.ipaddr.substring(1);
}
public final void parse(String ipaddr) {
this.ipaddr = ipaddr;
octets = new int[4];
StringTokenizer token = new StringTokenizer(ipaddr, SEPARATOR);
int index = 0;
while (token.hasMoreTokens()) {
String ipNum = token.nextToken();
octets[index++] = Integer.valueOf(ipNum).intValue();
}
}
public int compareTo(IpV4 ip) {
return compareTo(this, ip);
}
public static int compareTo(IpV4 ip1, IpV4 ip2) {
int compareResult = 0;
for (int i = 0; i < ip1.octets.length; i++) {
compareResult = ip1.compareOctet(ip1.octets[i], ip2.octets[i]);
if (compareResult != 0) {
return compareResult;
}
}
return compareResult;
}
private int compareOctet(int firstOctet, int secondOctet) {
if (firstOctet < secondOctet) {
return -1;
} else if (firstOctet > secondOctet) {
return 1;
} else {
return 0;
}
}
public String getIpaddr() {
return ipaddr;
}
public void setIpaddr(String ipaddr) {
this.ipaddr = ipaddr;
}
public int[] getOctets() {
return octets;
}
public void setOctets(int[] octets) {
this.octets = octets;
}
public IpV4 nextIp() {
int[] clonedOctets = cloneOctets();
for (int i = clonedOctets.length - 1; i > 0; i--) {
if (clonedOctets[i] < 255) {
clonedOctets[i]++;
break;
} else if (clonedOctets[i] == 255) {
clonedOctets[i] = 1;
}
}
return new IpV4(clonedOctets);
}
private int[] cloneOctets() {
int[] clonedOctets = new int[octets.length];
System.arraycopy(octets, 0, clonedOctets, 0, octets.length);
return clonedOctets;
}
public String get16Subnet() {
return octets[0] + SEPARATOR + octets[1] + SEPARATOR + SUBNET_16_SUFFIX + MASK_16;
}
public boolean isLANIP() {
if (octets[0] == 10) {
return true;
} else if (octets[0] == 100 && (octets[1] >= 64 && octets[1] <= 127)) {
return true;
} else if (octets[0] == 172 && (octets[1] >= 16 && octets[1] <= 31)) {
return true;
} else if (octets[0] == 192 && octets[1] == 168) {
return true;
} else {
return false;
}
}
public boolean isExternal() {
return !isLANIP() && !isLoopback();
}
public boolean isLoopback() {
return octets[0] == 127 && octets[1] == 0 && octets[2] == 0 && octets[3] == 1;
}
public static void main(String[] args) {
IpV4 ipV4 = new IpV4("127.0.0.1");
System.out.println(ipV4.isLoopback());
System.out.println(ipV4.isLANIP());
System.out.println(ipV4.isExternal());
}
@Override
public String toString() {
return this.ipaddr;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy