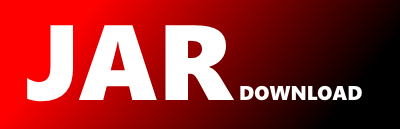
com.jelastic.api.utils.JavaNetUtils Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 54f28d1bbcaf782e3f02d6d15fd43b60*/
package com.jelastic.api.utils;
import java.io.IOException;
import java.net.IDN;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Socket;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class JavaNetUtils {
public static boolean isReachableHost(String ipAddress, int timeout) {
try {
InetAddress address = InetAddress.getByAddress(getStringIpAsBytes(ipAddress));
if (address.isReachable(timeout)) {
return true;
} else {
return false;
}
} catch (Exception ex) {
ex.printStackTrace();
return false;
}
}
static boolean canConvertToPunycode(String domain) {
try {
IDN.toASCII(domain);
return true;
} catch (IllegalArgumentException e) {
return false;
}
}
public static String convertToPunycode(String value) {
if (value == null) {
return null;
}
return IDN.toASCII(value);
}
public static byte[] getStringIpAsBytes(String ipAddress) {
String[] ints = ipAddress.split("\\.");
byte[] b = new byte[4];
b[0] = new Integer(ints[0]).byteValue();
b[1] = new Integer(ints[1]).byteValue();
b[2] = new Integer(ints[2]).byteValue();
b[3] = new Integer(ints[3]).byteValue();
return b;
}
public static boolean isTCPPortReachable(String host, int port, int timeout) {
try (Socket socket = new Socket()) {
socket.connect(new InetSocketAddress(host, port), timeout);
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy