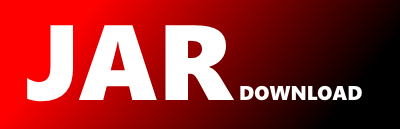
com.jfinal.plugin.activerecord.Page Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfinal Show documentation
Show all versions of jfinal Show documentation
JFinal is a simple, light, rapid,independent, extensible Java WEB + ORM framework. The feature of JFinal looks like ruby on rails especially ActiveRecord.
/**
* Copyright (c) 2011-2021, James Zhan 詹波 ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jfinal.plugin.activerecord;
import java.io.Serializable;
import java.util.List;
/**
* Page is the result of Model.paginate(......) or Db.paginate(......)
*/
public class Page implements Serializable {
private static final long serialVersionUID = -7102129155309986923L;
private List list; // list result of this page
private int pageNumber; // page number
private int pageSize; // result amount of this page
private int totalPage; // total page
private int totalRow; // total row
/**
* Constructor.
* @param list the list of paginate result
* @param pageNumber the page number
* @param pageSize the page size
* @param totalPage the total page of paginate
* @param totalRow the total row of paginate
*/
public Page(List list, int pageNumber, int pageSize, int totalPage, int totalRow) {
this.list = list;
this.pageNumber = pageNumber;
this.pageSize = pageSize;
this.totalPage = totalPage;
this.totalRow = totalRow;
}
public Page() {
}
/**
* Return list of this page.
*/
public List getList() {
return list;
}
public void setList(List list) {
this.list = list;
}
/**
* Return page number.
*/
public int getPageNumber() {
return pageNumber;
}
public void setPageNumber(int pageNumber) {
this.pageNumber = pageNumber;
}
/**
* Return page size.
*/
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
/**
* Return total page.
*/
public int getTotalPage() {
return totalPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
/**
* Return total row.
*/
public int getTotalRow() {
return totalRow;
}
public void setTotalRow(int totalRow) {
this.totalRow = totalRow;
}
public boolean isFirstPage() {
return pageNumber == 1;
}
public boolean isLastPage() {
return pageNumber >= totalPage;
}
public String toString() {
StringBuilder msg = new StringBuilder();
msg.append("pageNumber : ").append(pageNumber);
msg.append("\npageSize : ").append(pageSize);
msg.append("\ntotalPage : ").append(totalPage);
msg.append("\ntotalRow : ").append(totalRow);
return msg.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy