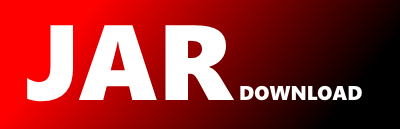
com.jfoenix.controls.JFXListView Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.jfoenix.controls;
import com.jfoenix.skins.JFXListViewSkin;
import javafx.beans.property.*;
import javafx.collections.FXCollections;
import javafx.collections.ListChangeListener;
import javafx.collections.ObservableList;
import javafx.css.*;
import javafx.css.converter.BooleanConverter;
import javafx.css.converter.SizeConverter;
import javafx.event.Event;
import javafx.scene.Node;
import javafx.scene.control.Control;
import javafx.scene.control.Label;
import javafx.scene.control.ListView;
import javafx.scene.control.Skin;
import javafx.scene.input.ContextMenuEvent;
import javafx.scene.input.MouseEvent;
import java.util.*;
/**
* Material design implementation of List View
*
* @author Shadi Shaheen
* @version 1.0
* @since 2016-03-09
*/
public class JFXListView extends ListView {
/**
* {@inheritDoc}
*/
public JFXListView() {
this.setCellFactory(listView -> new JFXListCell<>());
initialize();
}
/**
* {@inheritDoc}
*/
@Override
protected Skin> createDefaultSkin() {
return new JFXListViewSkin<>(this);
}
private ObjectProperty depthProperty = new SimpleObjectProperty<>(0);
public ObjectProperty depthProperty() {
return depthProperty;
}
public int getDepth() {
return depthProperty.get();
}
public void setDepth(int depth) {
depthProperty.set(depth);
}
private ReadOnlyDoubleWrapper currentVerticalGapProperty = new ReadOnlyDoubleWrapper();
ReadOnlyDoubleProperty currentVerticalGapProperty() {
return currentVerticalGapProperty.getReadOnlyProperty();
}
private void expand() {
currentVerticalGapProperty.set(verticalGap.get());
}
private void collapse() {
currentVerticalGapProperty.set(0);
}
/*
* this only works if the items were labels / strings
*/
private BooleanProperty showTooltip = new SimpleBooleanProperty(false);
public final BooleanProperty showTooltipProperty() {
return this.showTooltip;
}
public final boolean isShowTooltip() {
return this.showTooltipProperty().get();
}
public final void setShowTooltip(final boolean showTooltip) {
this.showTooltipProperty().set(showTooltip);
}
/***************************************************************************
* *
* SubList Properties *
* *
**************************************************************************/
@Deprecated
private ObjectProperty groupnode = new SimpleObjectProperty<>(new Label("GROUP"));
@Deprecated
public Node getGroupnode() {
return groupnode.get();
}
@Deprecated
public void setGroupnode(Node node) {
this.groupnode.set(node);
}
/*
* selected index property that includes the sublists
*/
@Deprecated
private ReadOnlyObjectWrapper overAllIndexProperty = new ReadOnlyObjectWrapper<>(-1);
@Deprecated
public ReadOnlyObjectProperty overAllIndexProperty() {
return overAllIndexProperty.getReadOnlyProperty();
}
// private sublists property
@Deprecated
private ObjectProperty>> sublistsProperty = new SimpleObjectProperty<>(
FXCollections.observableArrayList());
@Deprecated
private LinkedHashMap> sublistsIndices = new LinkedHashMap<>();
// this method shouldn't be called from user
@Deprecated
void addSublist(JFXListView> subList, int index) {
if (!sublistsProperty.get().contains(subList)) {
sublistsProperty.get().add(subList);
sublistsIndices.put(index, subList);
subList.getSelectionModel().selectedIndexProperty().addListener((o, oldVal, newVal) -> {
if (newVal.intValue() != -1) {
updateOverAllSelectedIndex();
}
});
}
}
private void updateOverAllSelectedIndex() {
// if item from the list is selected
if (this.getSelectionModel().getSelectedIndex() != -1) {
int selectedIndex = this.getSelectionModel().getSelectedIndex();
Iterator>> itr = sublistsIndices.entrySet().iterator();
int preItemsSize = 0;
while (itr.hasNext()) {
Map.Entry> entry = itr.next();
if (entry.getKey() < selectedIndex) {
preItemsSize += entry.getValue().getItems().size() - 1;
}
}
overAllIndexProperty.set(selectedIndex + preItemsSize);
} else {
Iterator>> itr = sublistsIndices.entrySet().iterator();
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy