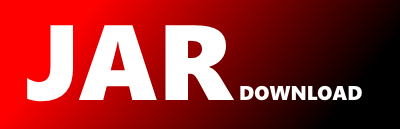
com.jgoodies.binding.formatter.EmptyDateFormatter Maven / Gradle / Ivy
/*
* Copyright (c) 2003-2008 JGoodies Karsten Lentzsch. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* o Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* o Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* o Neither the name of JGoodies Karsten Lentzsch nor the names of
* its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.jgoodies.binding.formatter;
import java.text.DateFormat;
import java.text.ParseException;
import java.util.Date;
import javax.swing.text.DateFormatter;
import com.jgoodies.binding.BindingUtils;
/**
* In addition to its superclass DateFormatter, this class converts
* to/from the empty string. Therefore it holds an empty value
* that is the counterpart of the empty string.
* The Method #valueToString
converts the empty value to the
* empty string. And #stringToValue
converts blank strings
* to the empty value. In all other cases the conversion is delegated
* to its superclass.
*
* Often the empty value is {@code null}. As an alternative you can map
* the empty string to a given date, for example epoch (January 1, 1970).
*
* Examples:
* new EmptyDateFormatter();
* new EmptyDateFormatter(new Date(0));
*
*
* @author Karsten Lentzsch
* @version $Revision: 1.6 $
*
* @see java.text.Format
*/
public class EmptyDateFormatter extends DateFormatter {
/**
* Holds the date that is converted to an empty string and
* that is the result of converting blank strings to a value.
*
* @see #stringToValue(String)
* @see #valueToString(Object)
*/
private final Date emptyValue;
// Instance Creation ****************************************************
/**
* Constructs an EmptyDateFormatter that converts {@code null}
* to the empty string and vice versa.
*/
public EmptyDateFormatter() {
this((Date) null);
}
/**
* Constructs an EmptyDateFormatter configured with the specified
* Format; maps {@code null} to the empty string and vice versa.
*
* @param format Format used to dictate legal values
*/
public EmptyDateFormatter(DateFormat format) {
this(format, null);
}
/**
* Constructs an EmptyDateFormatter that converts the given
* emptyValue
to the empty string and vice versa.
*
* @param emptyValue the representation of the empty string
*/
public EmptyDateFormatter(Date emptyValue) {
this.emptyValue = emptyValue == null
? null
: new Date(emptyValue.getTime());
}
/**
* Constructs an EmptyDateFormatter configured with the specified
* Format; maps {@code null} to the given emptyValue
* and vice versa.
*
* @param format Format used to dictate legal values
* @param emptyValue the representation of the empty string
*/
public EmptyDateFormatter(DateFormat format, Date emptyValue) {
super(format);
this.emptyValue = emptyValue == null
? null
: new Date(emptyValue.getTime());
}
// Overriding Superclass Behavior *****************************************
/**
* Returns the Object
representation of the
* String
text
.
*
* Unlike its superclass, this class converts blank strings
* to the empty value.
*
* @param text String
to convert
* @return Object
representation of text
* @throws ParseException if there is an error in the conversion
*/
@Override
public Object stringToValue(String text) throws ParseException {
return BindingUtils.isBlank(text)
? emptyValue
: super.stringToValue(text);
}
/**
* Returns a String representation of the Object value
.
* This invokes format
on the current Format
.
*
* Unlike its superclass, this class converts the empty value
* to the empty string.
*
* @param value the value to convert
* @return a String representation of value
* @throws ParseException if there is an error in the conversion
*/
@Override
public String valueToString(Object value) throws ParseException {
return BindingUtils.equals(value, emptyValue)
? ""
: super.valueToString(value);
}
}