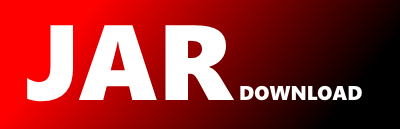
com.jianggujin.http.core.JRequest Maven / Gradle / Ivy
package com.jianggujin.http.core;
import java.io.IOException;
import java.io.InputStream;
import java.net.InetSocketAddress;
import java.net.MalformedURLException;
import java.net.Proxy;
import java.net.URL;
import java.nio.charset.Charset;
import java.nio.charset.IllegalCharsetNameException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.jianggujin.http.response.JNoBodyResponse;
import com.jianggujin.http.util.JDataUtils;
import com.jianggujin.http.util.JKeyVal;
/**
* 请求
*
* @author jianggujin
*
*/
public class JRequest {
/**
* 代理
*/
private Proxy proxy; // nullable
/**
* 超时时间
*/
private int timeoutMilliseconds;
/**
* 请求参数
*/
private List data;
/**
* 请求体
*/
private Object body = null;
/**
* 数据编码
*/
private String charset = JDataUtils.defaultCharset;
/**
* 请求地址
*/
protected URL url;
/**
* 请求方法
*/
protected JMethod method;
/**
* 头信息
*/
protected Map headers;
/**
* cookie信息
*/
Map cookies;
/**
* SSL
*/
private JSSLContextFactory sslContextFactory = null;
/**
* 响应对象
*/
private JResponse response;
/**
* 忽略HTTP响应式状态码错误
*/
private boolean ignoreError = false;
/**
* 请求解析器
*/
private JRequestBodyResolver requestBodyResolver;
public JRequest() {
}
public JRequest(URL url) {
this(url, null, null);
}
public JRequest(URL url, JResponse response) {
this(url, null, response);
}
public JRequest(URL url, JMethod method, JResponse response) {
this.url = url;
this.headers = new LinkedHashMap();
this.cookies = new LinkedHashMap();
this.data = new ArrayList();
this.headers.put("Accept-Encoding", "gzip");
this.method = method == null ? JMethod.GET : method;
this.response = response == null ? new JNoBodyResponse() : response;
this.timeoutMilliseconds = 5000;
}
/**
* 获得请求地址
*
* @return url
*/
public URL url() {
return url;
}
/**
* 设置请求地址
*
* @param url
* 请求地址
* @return {@link JRequest}
*/
public JRequest url(URL url) {
if (url == null) {
throw new IllegalArgumentException("url must not be null");
}
this.url = url;
return this;
}
/**
* 设置请求地址
*
* @param url
* 请求地址
* @return {@link JRequest}
*/
public JRequest url(String url) {
if (url == null) {
throw new IllegalArgumentException("url must not be null");
}
try {
this.url = new URL(url);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Malformed URL: " + url, e);
}
return this;
}
/**
* 获得请求方法
*
* @return {@link JMethod}
*/
public JMethod method() {
return method;
}
/**
* 设置请求方法
*
* @param method
* 请求方法
* @return {@link JRequest}
*/
public JRequest method(JMethod method) {
if (method == null) {
throw new IllegalArgumentException("method must not be null");
}
this.method = method;
return this;
}
/**
* 获得头信息
*
* @param name
* 头信息的名称
* @return 头信息的值
*/
public String header(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
return getHeaderCaseInsensitive(name);
}
/**
* 设置头信息
*
* @param name
* 头信息的名称
* @param value
* 头信息的值
* @return {@link JRequest}
*/
public JRequest header(String name, String value) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
if (value == null) {
throw new IllegalArgumentException("Header value must not be empty.");
}
removeHeader(name); // ensures we don't get an "accept-encoding" and a
// "Accept-Encoding"
headers.put(name, value);
return this;
}
/**
* 设置头信息
*
* @param headers
* @return
*/
public JRequest header(Map headers) {
if (headers == null) {
return this;
}
Iterator> iterator = headers.entrySet().iterator();
while (iterator.hasNext()) {
Entry entry = iterator.next();
header(entry.getKey(), entry.getValue());
}
return this;
}
/**
* 判断是否存在头
*
* @param name
* @return
*/
public boolean hasHeader(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
return getHeaderCaseInsensitive(name) != null;
}
/**
* 判断是否存在指定值的头
*/
public boolean hasHeaderWithValue(String name, String value) {
return hasHeader(name) && header(name).equalsIgnoreCase(value);
}
/**
* 移除头
*
* @param name
* @return
*/
public JRequest removeHeader(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
Map.Entry entry = scanHeaders(name); // remove is case
// insensitive too
if (entry != null) {
headers.remove(entry.getKey()); // ensures correct case
}
return this;
}
/**
* 头
*
* @return
*/
public Map headers() {
return headers;
}
/**
* 获得头信息
*
* @param name
* @return
*/
private String getHeaderCaseInsensitive(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
// quick evals for common case of title case, lower case, then scan for
// mixed
String value = headers.get(name);
if (value == null) {
value = headers.get(name.toLowerCase());
}
if (value == null) {
Map.Entry entry = scanHeaders(name);
if (entry != null) {
value = entry.getValue();
}
}
return value;
}
/**
* 扫描头
*
* @param name
* @return
*/
private Entry scanHeaders(String name) {
String lc = name.toLowerCase();
for (Map.Entry entry : headers.entrySet()) {
if (entry.getKey().toLowerCase().equals(lc)) {
return entry;
}
}
return null;
}
/**
* 获得cookie
*
* @param name
* @return
*/
public String cookie(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Cookie name must not be empty");
}
return cookies.get(name);
}
/**
* 设置cookie
*
* @param name
* @param value
* @return
*/
public JRequest cookie(String name, String value) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Cookie name must not be empty.");
}
if (value == null) {
throw new IllegalArgumentException("Cookie value must not be empty.");
}
cookies.put(name, value);
return this;
}
/**
* 设置cookie
*
* @param cookies
* @return
*/
public JRequest cookie(Map cookies) {
if (cookies == null) {
return this;
}
Iterator> iterator = cookies.entrySet().iterator();
while (iterator.hasNext()) {
Entry entry = iterator.next();
cookie(entry.getKey(), entry.getValue());
}
return this;
}
/**
* 判断是否存在cookie
*
* @param name
* @return
*/
public boolean hasCookie(String name) {
return cookies.containsKey(name);
}
/**
* 移除cookie
*
* @param name
* @return
*/
public JRequest removeCookie(String name) {
cookies.remove(name);
return this;
}
/**
* 获得所有cookie
*
* @return
*/
public Map cookies() {
return cookies;
}
/**
* 获得代理
*
* @return
*/
public Proxy proxy() {
return proxy;
}
/**
* 设置代理
*
* @param proxy
* @return
*/
public JRequest proxy(Proxy proxy) {
this.proxy = proxy;
return this;
}
/**
* 设置代理
*
* @param host
* @param port
* @return
*/
public JRequest proxy(String host, int port) {
this.proxy = new Proxy(Proxy.Type.HTTP, InetSocketAddress.createUnresolved(host, port));
return this;
}
/**
* 获得超时时间
*
* @return
*/
public int timeout() {
return timeoutMilliseconds;
}
/**
* 设置超时时间
*
* @param millis
* @return
*/
public JRequest timeout(int millis) {
if (millis < 0) {
throw new IllegalArgumentException("Timeout milliseconds must be 0 (infinite) or greater");
}
timeoutMilliseconds = millis;
return this;
}
public JSSLContextFactory sslContextFactory() {
return sslContextFactory;
}
public JRequest sslContextFactory(JSSLContextFactory sslContextFactory) {
this.sslContextFactory = sslContextFactory;
return this;
}
/**
* 添加请求参数
*
* @param keyval
* @return
*/
public JRequest data(JKeyVal keyval) {
if (keyval == null) {
throw new IllegalArgumentException("Key val must not be null");
}
data.add(keyval);
return this;
}
/**
* 添加请求参数
*
* @param key
* @param value
* @return
*/
public JRequest data(String key, String value) {
data(JKeyVal.create(key, value));
return this;
}
/**
* 添加请求参数
*
* @param key
* @param filename
* @param inputStream
* @return
*/
public JRequest data(String key, String filename, InputStream inputStream) {
data(JKeyVal.create(key, filename, inputStream));
return this;
}
/**
* 添加请求参数
*
* @param data
* @return
*/
public JRequest data(Map data) {
if (data == null) {
return this;
}
for (Map.Entry entry : data.entrySet()) {
data(JKeyVal.create(entry.getKey(), entry.getValue()));
}
return this;
}
/**
* 获得请求参数
*
* @return
*/
public List data() {
return data;
}
/**
* 设置请求体
*
* @param body
* @return
*/
public JRequest requestBody(Object body) {
this.body = body;
return this;
}
/**
* 获得请求体
*
* @return
*/
public Object requestBody() {
return body;
}
/**
* 设置请求编码
*
* @param charset
* @return
*/
public JRequest charset(String charset) {
if (charset == null) {
throw new IllegalArgumentException("Charset must not be null");
}
if (!Charset.isSupported(charset)) {
throw new IllegalCharsetNameException(charset);
}
this.charset = charset;
return this;
}
/**
* 获得请求编码
*
* @return
*/
public String charset() {
return charset;
}
/**
* 忽略HTTP响应式状态码错误
*
* @return
*/
public boolean ignoreError() {
return ignoreError;
}
/**
* 忽略HTTP响应式状态码错误
*
* @param ignoreError
*/
public JRequest ignoreError(boolean ignoreError) {
this.ignoreError = ignoreError;
return this;
}
/**
* 执行请求
*
* @throws IOException
*/
public JRequest execute() throws IOException {
if (this.url == null) {
throw new IllegalStateException("url must not be null");
}
JRequestExecuter.execute(this);
return this;
}
/**
* 获得响应处理器
*
* @return
*/
public JResponse response() {
return response;
}
/**
* 设置响应处理器
*
* @param response
* @return
*/
public JRequest response(JResponse response) {
this.response = response;
return this;
}
/**
* 获得请求体解析器
*
* @return
*/
public JRequestBodyResolver requestBodyResolver() {
return requestBodyResolver;
}
/**
* 设置请求体解析器
*
* @param requestBodyResolver
* @return
*/
public JRequest requestBodyResolver(JRequestBodyResolver requestBodyResolver) {
this.requestBodyResolver = requestBodyResolver;
return this;
}
/**
* 创建请求对象
*
* @param url
* @return
*/
public static JRequest create(String url) {
if (JDataUtils.isEmpty(url)) {
throw new IllegalArgumentException("Must supply a valid URL");
}
try {
return new JRequest(new URL(url));
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Malformed URL: " + url, e);
}
}
/**
* 创建请求对象
*
* @param url
* @return
*/
public static JRequest create(URL url) {
return new JRequest(url);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy