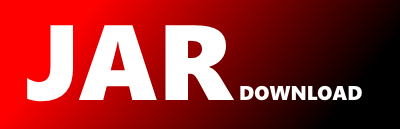
com.jianggujin.http.ssl.JAbstractSSLContextFactory Maven / Gradle / Ivy
package com.jianggujin.http.ssl;
import java.security.SecureRandom;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.KeyManager;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.X509TrustManager;
import com.jianggujin.http.core.JSSLContextFactory;
public abstract class JAbstractSSLContextFactory implements JSSLContextFactory {
/**
* 获得协议
*
* @return
*/
protected abstract String getProtocol();
/**
* 获得密钥管理器
*
* @return
*/
protected KeyManager[] getKeyManagers() {
return null;
}
public SSLContext getSSLContext() {
try {
// 实例化SSL上下文
SSLContext ctx = SSLContext.getInstance(getProtocol());
// 初始化SSL上下文
ctx.init(getKeyManagers(), new X509TrustManager[] { new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType)
throws java.security.cert.CertificateException {
}
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
} }, new SecureRandom());
return ctx;
} catch (Exception e) {
throw new IllegalArgumentException(e);
}
}
public HostnameVerifier getHostnameVerifier() {
HostnameVerifier verifier = new HostnameVerifier() {
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
return verifier;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy