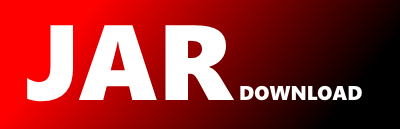
com.jianggujin.http.core.JRequest Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.http.core;
import java.io.InputStream;
import java.net.InetSocketAddress;
import java.net.MalformedURLException;
import java.net.Proxy;
import java.net.URL;
import java.nio.charset.Charset;
import java.nio.charset.IllegalCharsetNameException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.jianggujin.http.util.JDataUtils;
import com.jianggujin.http.util.JKeyVal;
/**
* 请求
*
* @author jianggujin
*
*/
public class JRequest {
/**
* 代理
*/
private Proxy proxy;
/**
* 超时时间
*/
private int timeoutMilliseconds;
/**
* 请求参数
*/
private List data;
/**
* 请求体
*/
private Object body = null;
/**
* 数据编码
*/
private String charset = JDataUtils.DEFAULT_CHARSET;
/**
* 请求地址
*/
protected URL url;
/**
* 请求方法
*/
protected JMethod method;
/**
* 请求头信息
*/
protected Map headers;
/**
* cookie信息
*/
Map cookies;
/**
* SSL
*/
private JSSLContextFactory sslContextFactory = null;
/**
* 响应对象
*/
private JResponse response;
/**
* 忽略HTTP响应式状态码错误
*/
private boolean ignoreError = false;
/**
* 强制是否含有请求体
*/
private boolean forceHasBody = false;
/**
* 请求解析器
*/
private JRequestBodyResolver requestBodyResolver;
public JRequest() {
this(null, null, null);
}
public JRequest(URL url) {
this(url, null, null);
}
public JRequest(URL url, JResponse response) {
this(url, null, response);
}
public JRequest(URL url, JMethod method, JResponse response) {
this.url = url;
// this.headers = new LinkedHashMap();
// this.cookies = new LinkedHashMap();
// this.data = new ArrayList();
// this.headers.put("Accept-Encoding", "gzip");
this.method = method == null ? JMethod.GET : method;
this.response = response;
this.timeoutMilliseconds = JDataUtils.DEFAULT_TIME_OUT;
}
/**
* 获得请求地址
*
* @return url
*/
public URL url() {
return url;
}
/**
* 设置请求地址
*
* @param url 请求地址
* @return {@link JRequest}
*/
public JRequest url(URL url) {
if (url == null) {
throw new IllegalArgumentException("url must not be null");
}
this.url = url;
return this;
}
/**
* 设置请求地址
*
* @param url 请求地址
* @return {@link JRequest}
*/
public JRequest url(String url) {
if (url == null) {
throw new IllegalArgumentException("url must not be null");
}
try {
this.url = new URL(url);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Malformed URL: " + url, e);
}
return this;
}
/**
* 获得请求方法
*
* @return {@link JMethod}
*/
public JMethod method() {
return method;
}
/**
* 设置请求方法
*
* @param method 请求方法
* @return {@link JRequest}
*/
public JRequest method(JMethod method) {
if (method == null) {
throw new IllegalArgumentException("method must not be null");
}
this.method = method;
return this;
}
/**
* 获得请求头信息
*
* @param name 请求头信息的名称
* @return 请求头信息的值
*/
public String header(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
return this.headers != null ? this.headers.get(name) : null;
}
/**
* 设置请求头信息
*
* @param name 请求头信息的名称
* @param value 请求头信息的值
* @return {@link JRequest}
*/
public JRequest header(String name, String value) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
if (this.headers == null) {
this.headers = new HashMap();
}
headers.put(name, value);
return this;
}
/**
* 设置请求头信息
*
* @param headers
* @return
*/
public JRequest header(Map headers) {
if (headers == null || headers.size() == 0) {
return this;
}
if (headers.containsKey("") || headers.containsKey(null)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
if (this.headers != null) {
this.headers.putAll(headers);
} else {
this.headers = new HashMap(headers);
}
return this;
}
/**
* 判断是否存在头
*
* @param name
* @return
*/
public boolean hasHeader(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
return this.headers != null ? this.headers.containsKey(name) : false;
}
/**
* 判断是否存在指定值的头
*/
public boolean hasHeaderWithValue(String name, String value) {
return hasHeader(name) && header(name).equals(value);
}
/**
* 移除头
*
* @param name
* @return
*/
public JRequest removeHeader(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Header name must not be empty.");
}
if (this.headers != null) {
headers.remove(name);
}
return this;
}
/**
* 请求头
*
* @return
*/
public Map headers() {
return headers == null ? null : Collections.unmodifiableMap(headers);
}
/**
* 获得cookie
*
* @param name
* @return
*/
public String cookie(String name) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Cookie name must not be empty");
}
return this.cookies != null ? cookies.get(name) : null;
}
/**
* 设置cookie
*
* @param name
* @param value
* @return
*/
public JRequest cookie(String name, String value) {
if (JDataUtils.isEmpty(name)) {
throw new IllegalArgumentException("Cookie name must not be empty.");
}
if (this.cookies == null) {
this.cookies = new HashMap();
}
cookies.put(name, value);
return this;
}
/**
* 设置cookie
*
* @param cookies
* @return
*/
public JRequest cookie(Map cookies) {
if (cookies == null) {
return this;
}
if (cookies.containsKey("") || cookies.containsKey(null)) {
throw new IllegalArgumentException("Cookie name must not be empty.");
}
if (this.cookies != null) {
this.cookies.putAll(cookies);
} else {
this.cookies = new HashMap(cookies);
}
return this;
}
/**
* 判断是否存在cookie
*
* @param name
* @return
*/
public boolean hasCookie(String name) {
return this.cookies != null ? cookies.containsKey(name) : false;
}
/**
* 移除cookie
*
* @param name
* @return
*/
public JRequest removeCookie(String name) {
if (this.cookies != null) {
cookies.remove(name);
}
return this;
}
/**
* 获得所有cookie
*
* @return
*/
public Map cookies() {
return cookies == null ? null : Collections.unmodifiableMap(cookies);
}
/**
* 获得代理
*
* @return
*/
public Proxy proxy() {
return proxy;
}
/**
* 设置代理
*
* @param proxy
* @return
*/
public JRequest proxy(Proxy proxy) {
this.proxy = proxy;
return this;
}
/**
* 设置代理
*
* @param host
* @param port
* @return
*/
public JRequest proxy(String host, int port) {
this.proxy = new Proxy(Proxy.Type.HTTP, InetSocketAddress.createUnresolved(host, port));
return this;
}
/**
* 获得超时时间
*
* @return
*/
public int timeout() {
return timeoutMilliseconds;
}
/**
* 设置超时时间
*
* @param millis
* @return
*/
public JRequest timeout(int millis) {
if (millis < 0) {
throw new IllegalArgumentException("Timeout milliseconds must be 0 (infinite) or greater");
}
timeoutMilliseconds = millis;
return this;
}
public JSSLContextFactory sslContextFactory() {
return sslContextFactory;
}
public JRequest sslContextFactory(JSSLContextFactory sslContextFactory) {
this.sslContextFactory = sslContextFactory;
return this;
}
/**
* 添加请求参数
*
* @param keyval
* @return
*/
public JRequest data(JKeyVal keyval) {
if (keyval == null) {
return this;
}
if (this.data == null) {
this.data = new ArrayList();
}
data.add(keyval);
return this;
}
/**
* 添加请求参数
*
* @param key
* @param value
* @return
*/
public JRequest data(String key, String value) {
data(JKeyVal.create(key, value));
return this;
}
/**
* 添加请求参数
*
* @param key
* @param filename
* @param inputStream
* @return
*/
public JRequest data(String key, String filename, InputStream inputStream) {
data(JKeyVal.create(key, filename, inputStream));
return this;
}
/**
* 添加请求参数
*
* @param data
* @return
*/
public JRequest data(Map data) {
if (data == null || data.isEmpty()) {
return this;
}
for (Map.Entry entry : data.entrySet()) {
data(JKeyVal.create(entry.getKey(), entry.getValue()));
}
return this;
}
/**
* 获得请求参数
*
* @return
*/
public List data() {
return data;
}
/**
* 设置请求体
*
* @param body
* @return
*/
public JRequest requestBody(Object body) {
this.body = body;
return this;
}
/**
* 获得请求体
*
* @return
*/
public Object requestBody() {
return body;
}
/**
* 设置请求编码
*
* @param charset
* @return
*/
public JRequest charset(String charset) {
if (charset == null) {
throw new IllegalArgumentException("Charset must not be null");
}
if (!Charset.isSupported(charset)) {
throw new IllegalCharsetNameException(charset);
}
this.charset = charset;
return this;
}
/**
* 获得请求编码
*
* @return
*/
public String charset() {
return charset;
}
/**
* 忽略HTTP响应式状态码错误
*
* @return
*/
public boolean ignoreError() {
return ignoreError;
}
/**
* 忽略HTTP响应式状态码错误
*
* @param ignoreError
*/
public JRequest ignoreError(boolean ignoreError) {
this.ignoreError = ignoreError;
return this;
}
/**
* 强制是否含有请求体的判断
*
* @return
*/
public boolean forceHasBody() {
return forceHasBody;
}
/**
* 强制是否含有请求体的判断
*
* @param forceHasBody
*/
public JRequest forceHasBody(boolean forceHasBody) {
this.forceHasBody = forceHasBody;
return this;
}
/**
* 执行请求
*
* @throws JHttpException
*/
public JRequest execute() throws JHttpException {
if (this.url == null) {
throw new IllegalStateException("url must not be null");
}
JRequestExecuter.execute(this);
return this;
}
/**
* POST请求
*
* @return
* @throws JHttpException
*/
public JRequest post() throws JHttpException {
method(JMethod.POST);
return execute();
}
/**
* GET请求
*
* @return
* @throws JHttpException
*/
public JRequest get() throws JHttpException {
method(JMethod.GET);
return execute();
}
/**
* PUT请求
*
* @return
* @throws JHttpException
*/
public JRequest put() throws JHttpException {
method(JMethod.PUT);
return execute();
}
/**
* DELETE请求
*
* @return
* @throws JHttpException
*/
public JRequest delete() throws JHttpException {
method(JMethod.DELETE);
return execute();
}
/**
* 获得响应处理器
*
* @return
*/
public JResponse response() {
return response;
}
/**
* 设置响应处理器
*
* @param response
* @return
*/
public JRequest response(JResponse response) {
this.response = response;
return this;
}
/**
* 获得请求体解析器
*
* @return
*/
public JRequestBodyResolver requestBodyResolver() {
return requestBodyResolver;
}
/**
* 设置请求体解析器
*
* @param requestBodyResolver
* @return
*/
public JRequest requestBodyResolver(JRequestBodyResolver requestBodyResolver) {
this.requestBodyResolver = requestBodyResolver;
return this;
}
/**
* 创建请求对象
*
* @param url
* @return
*/
public static JRequest create(String url) {
if (JDataUtils.isEmpty(url)) {
throw new IllegalArgumentException("Must supply a valid URL");
}
try {
return new JRequest(new URL(url));
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Malformed URL: " + url, e);
}
}
/**
* 创建请求对象
*
* @param url
* @return
*/
public static JRequest create(URL url) {
return new JRequest(url);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy