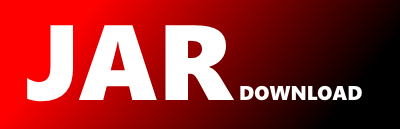
com.jianggujin.http.util.JOutputStream Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.http.util;
import java.io.BufferedOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
/**
* 输出流,支持字节字符输出
*
* @author jianggujin
*
*/
public class JOutputStream extends BufferedOutputStream {
private String charset = JDataUtils.DEFAULT_CHARSET;
public JOutputStream(OutputStream out) {
super(out);
}
public JOutputStream(OutputStream out, String charset) {
super(out);
if (!Charset.isSupported(charset)) {
throw new IllegalArgumentException("not support charset [" + charset + "]");
}
this.charset = charset;
}
public JOutputStream(OutputStream out, int size) {
super(out, size);
}
/**
* 获得字符编码
*
* @return
*/
public String getCharset() {
return charset;
}
/**
* 设置字符编码
*
* @param charset
*/
public void setCharset(String charset) {
this.charset = charset;
}
/**
* 写入字符
*
* @param c
* @throws IOException
*/
public void write(char c) throws IOException {
this.write(new char[] { c });
}
/**
* 写入字符数组
*
* @param c
* @throws IOException
*/
public void write(char[] c) throws IOException {
this.write(c, 0, c.length);
}
/**
* 写入字符数组
*
* @param cbuf
* @param off
* @param len
* @throws IOException
*/
public void write(char[] cbuf, int off, int len) throws IOException {
// this.write(Charset.forName(charset).encode(CharBuffer.wrap(cbuf, off,
// len)));
this.write(new String(cbuf, off, len));
}
/**
* 写入字符序列
*
* @param cs
* @throws IOException
*/
public void write(CharSequence cs) throws IOException {
this.write(cs.toString().getBytes(charset));
// this.write(cs, 0, cs.length());
}
/**
* 写入字符序列
*
* @param cs
* @param off
* @param len
* @throws IOException
*/
public void write(CharSequence cs, int off, int len) throws IOException {
// this.write(Charset.forName(charset).encode(CharBuffer.wrap(cs, off,
// len)));
this.write(cs.subSequence(off, len));
}
/**
* 写入字节缓冲区
*
* @param buffer
* @throws IOException
*/
public void write(ByteBuffer buffer) throws IOException {
this.write(buffer.array());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy