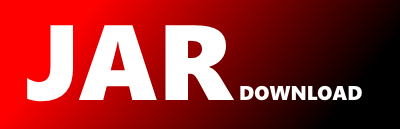
com.jianggujin.logging.slf4j.JSlf4jImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JLogging Show documentation
Show all versions of JLogging Show documentation
Java日志工具,自动加载已有的日志包,相当于日志工具的适配器
The newest version!
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.logging.slf4j;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.Marker;
import org.slf4j.spi.LocationAwareLogger;
import com.jianggujin.logging.JLog;
/**
* slf4j实现
*
* @author jianggujin
*
*/
public class JSlf4jImpl implements JLog {
private JLog log;
public JSlf4jImpl(String clazz) {
Logger logger = LoggerFactory.getLogger(clazz);
if (logger instanceof LocationAwareLogger) {
try {
// check for slf4j >= 1.6 method signature
logger.getClass().getMethod("log", Marker.class, String.class, int.class, String.class, Object[].class,
Throwable.class);
log = new JSlf4jLocationAwareLoggerImpl((LocationAwareLogger) logger);
return;
} catch (SecurityException e) {
// fail-back to Slf4jLoggerImpl
} catch (NoSuchMethodException e) {
// fail-back to Slf4jLoggerImpl
}
}
// Logger is not LocationAwareLogger or slf4j version < 1.6
log = new JSlf4jLoggerImpl(logger);
}
public boolean isDebugEnabled() {
return log.isDebugEnabled();
}
public boolean isTraceEnabled() {
return log.isTraceEnabled();
}
public void error(String s, Throwable e) {
log.error(s, e);
}
public void error(String s) {
log.error(s);
}
public void debug(String s) {
log.debug(s);
}
public void trace(String s) {
log.trace(s);
}
public void warn(String s) {
log.warn(s);
}
public void info(String s) {
log.info(s);
}
public void debug(String s, Throwable e) {
log.debug(s, e);
}
public void trace(String s, Throwable e) {
log.trace(s, e);
}
public void warn(String s, Throwable e) {
log.warn(s, e);
}
public void info(String s, Throwable e) {
log.info(s, e);
}
public boolean isErrorEnabled() {
return log.isErrorEnabled();
}
public boolean isInfoEnabled() {
return log.isInfoEnabled();
}
public boolean isWarnEnabled() {
return log.isWarnEnabled();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy