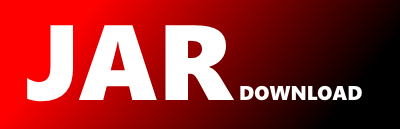
com.jianggujin.modulelink.JModuleConfig Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink;
import java.net.URL;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import com.jianggujin.modulelink.util.JAssert;
/**
* 模块配置信息
*
* @author jianggujin
*
*/
public class JModuleConfig implements Comparable {
/**
* 模块名,建议用英文命名
*/
private final String name;
/**
* 模块描述
*/
private String desc;
/**
* 模块的版本
*/
private final String version;
/**
* 模块指定需要覆盖的Class的包名,不遵循双亲委派, 模块的类加载器加载这些包
*
* 如果子模块中加载不到那么仍然会到父容器中加载
*/
private Set overridePackages = new HashSet();
/**
* JAR 包资源地址,模块存放的地方
*/
private Set moduleUrls = new HashSet();
/**
* 指定使用的Action
*/
private Set actions = new HashSet();
/**
* 需要排除的Action
*/
private Set exclusionActions = new HashSet();
/**
* 扫描包, 当该set不为空时启动包扫描
* 1、默认实现会扫描包下面的{@link JAction}实现类
* 2、spring扫描注解的包,将自动扫描注解形式的bean
*
* 当xml中和注解同时定义了一个相同名字的bean将会以xml中的为主,也就是注解定义的bean会被xml定义的bean
* 覆盖
*
* xml中的bean不能依赖注解bean,注解bean可以依赖xml定义的bean
*/
private Set scanPackages = new HashSet();
/**
* 是否激活,仅当模块有多个版本的时候有效
*/
private boolean active;
public JModuleConfig(String name, String version) {
JAssert.checkNotNull(name, "name must not be null");
JAssert.checkNotNull(version, "version must not be null");
this.name = name;
this.version = version;
}
/**
* 获得模块名称
*
* @return
*/
public String getName() {
return name;
}
/**
* 获得模块描述
*
* @return
*/
public String getDesc() {
return desc;
}
/**
* 设置模块描述
*
* @param desc
*/
public void setDesc(String desc) {
this.desc = desc;
}
public JModuleConfig withDesc(String desc) {
this.setDesc(desc);
return this;
}
/**
* 获得模块版本
*
* @return
*/
public String getVersion() {
return version;
}
/**
* 获得需要打破双亲委托的包名
*
* @return
*/
public Set getOverridePackages() {
return overridePackages;
}
/**
* 设置需要打破双亲委托的包名
*
* @param overridePackages
*/
public void setOverridePackages(Set overridePackages) {
JAssert.checkNotNull(overridePackages, "overridePackages must not be null");
this.overridePackages = overridePackages;
}
public JModuleConfig withOverridePackages(Set overridePackages) {
this.setOverridePackages(overridePackages);
return this;
}
/**
* 添加需要打破双亲委托的包名
*
* @param overridePackages
* @return
*/
public JModuleConfig addOverridePackages(Collection overridePackages) {
if (overridePackages != null) {
this.overridePackages.addAll(overridePackages);
}
return this;
}
/**
* 添加需要打破双亲委托的包名
*
* @param packageName
* @return
*/
public JModuleConfig addOverridePackage(String packageName) {
JAssert.checkNotNull(packageName, "packageName must not be null");
this.overridePackages.add(packageName);
return this;
}
/**
* 移除需要打破双亲委托的包名
*
* @param packageName
* @return
*/
public JModuleConfig removeOverridePackage(String packageName) {
this.overridePackages.remove(packageName);
return this;
}
/**
* 获得模块地址
*
* @return
*/
public Set getModuleUrls() {
return moduleUrls;
}
/**
* 设置模块地址
*
* @param moduleUrls
*/
public void setModuleUrls(Set moduleUrls) {
JAssert.checkNotNull(moduleUrls, "moduleUrls must not be null");
this.moduleUrls = moduleUrls;
}
public JModuleConfig withModuleUrls(Set moduleUrls) {
this.setModuleUrls(moduleUrls);
return this;
}
/**
* 添加模块地址
*
* @param moduleUrls
* @return
*/
public JModuleConfig addModuleUrls(Collection moduleUrls) {
if (moduleUrls != null) {
this.moduleUrls.addAll(moduleUrls);
}
return this;
}
/**
* 添加模块地址
*
* @param moduleUrl
* @return
*/
public JModuleConfig addModuleUrl(URL moduleUrl) {
JAssert.checkNotNull(moduleUrl, "moduleUrl must not be null");
this.moduleUrls.add(moduleUrl);
return this;
}
/**
* 移除模块地址
*
* @param moduleUrl
* @return
*/
public JModuleConfig removeModuleUrl(URL moduleUrl) {
this.moduleUrls.remove(moduleUrl);
return this;
}
/**
* 获得Action
*
* @return
*/
public Set getActions() {
return actions;
}
/**
* 设置Action
*
* @param actions
*/
public void setActions(Set actions) {
JAssert.checkNotNull(actions, "actions must not be null");
this.actions = actions;
}
public JModuleConfig withActions(Set actions) {
this.setActions(actions);
return this;
}
/**
* 添加Action
*
* @param actions
* @return
*/
public JModuleConfig addActions(Collection actions) {
if (actions != null) {
this.actions.addAll(actions);
}
return this;
}
/**
* 添加Action
*
* @param action
* @return
*/
public JModuleConfig addAction(String action) {
JAssert.checkNotNull(action, "action must not be null");
this.actions.add(action);
return this;
}
/**
* 移除Action
*
* @param action
* @return
*/
public JModuleConfig removeAction(String action) {
this.actions.remove(action);
return this;
}
/**
* 获得移除的Action
*
* @return
*/
public Set getExclusionActions() {
return exclusionActions;
}
/**
* 设置移除的Action
*
* @param exclusionActions
*/
public void setExclusionActions(Set exclusionActions) {
JAssert.checkNotNull(exclusionActions, "exclusionActions must not be null");
this.exclusionActions = exclusionActions;
}
public JModuleConfig withExclusionActions(Set exclusionActions) {
this.setExclusionActions(exclusionActions);
return this;
}
/**
* 添加移除的Action
*
* @param exclusionActions
* @return
*/
public JModuleConfig addExclusionActions(Collection exclusionActions) {
if (exclusionActions != null) {
this.exclusionActions.addAll(exclusionActions);
}
return this;
}
/**
* 添加移除的Action
*
* @param exclusionAction
* @return
*/
public JModuleConfig addExclusionAction(String exclusionAction) {
JAssert.checkNotNull(exclusionAction, "exclusionAction must not be null");
this.exclusionActions.add(exclusionAction);
return this;
}
/**
* 移除移除的Action
*
* @param exclusionAction
* @return
*/
public JModuleConfig removeExclusionAction(String exclusionAction) {
this.exclusionActions.remove(exclusionAction);
return this;
}
/**
* 获得扫描包的包名
*
* @return
*/
public Set getScanPackages() {
return scanPackages;
}
/**
* 设置扫描包的包名
*
* @param scanPackages
*/
public void setScanPackages(Set scanPackages) {
JAssert.checkNotNull(scanPackages, "scanPackages must not be null");
this.scanPackages = scanPackages;
}
public JModuleConfig withScanPackages(Set scanPackages) {
this.setScanPackages(scanPackages);
return this;
}
/**
* 添加扫描包的包名
*
* @param scanPackages
* @return
*/
public JModuleConfig addScanPackages(Collection scanPackages) {
if (scanPackages != null) {
this.scanPackages.addAll(scanPackages);
}
return this;
}
/**
* 添加扫描包的包名
*
* @param packageName
* @return
*/
public JModuleConfig addScanPackage(String packageName) {
JAssert.checkNotNull(packageName, "packageName must not be null");
this.scanPackages.add(packageName);
return this;
}
/**
* 移除扫描包的包名
*
* @param packageName
* @return
*/
public JModuleConfig removeScanPackage(String packageName) {
this.scanPackages.remove(packageName);
return this;
}
public boolean isActive() {
return active;
}
public void setActive(boolean active) {
this.active = active;
}
public JModuleConfig withActive(boolean active) {
this.setActive(active);
return this;
}
@Override
public int compareTo(JModuleConfig other) {
String name = other.name;
String version = other.version;
int value = this.name.compareTo(name);
if (value == 0) {
return this.version.compareTo(version);
}
return value;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((name == null) ? 0 : name.hashCode());
result = prime * result + ((version == null) ? 0 : version.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
JModuleConfig other = (JModuleConfig) obj;
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
if (version == null) {
if (other.version != null) {
return false;
}
} else if (!version.equals(other.version)) {
return false;
}
return true;
}
@Override
public String toString() {
return "JModuleConfig [name=" + name + ", desc=" + desc + ", version=" + version + ", overridePackages="
+ overridePackages + ", moduleUrls=" + moduleUrls + ", actions=" + actions + ", exclusionActions="
+ exclusionActions + ", scanPackages=" + scanPackages + ", active=" + active + "]";
}
}