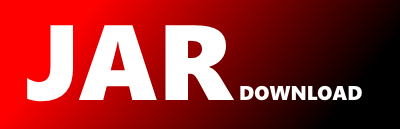
com.jianggujin.modulelink.impl.JAbstractModule Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.impl;
import java.beans.Introspector;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.ResourceBundle;
import java.util.Set;
import com.jianggujin.modulelink.JAction;
import com.jianggujin.modulelink.JModule;
import com.jianggujin.modulelink.JModuleConfig;
import com.jianggujin.modulelink.util.JAssert;
import com.jianggujin.modulelink.util.JLogFactory;
import com.jianggujin.modulelink.util.JLogFactory.JLog;
import com.jianggujin.modulelink.util.JModuleClassLoader;
import com.jianggujin.modulelink.util.JModuleUtils;
/**
* 模块抽象实现
*
* @author jianggujin
*
*/
public abstract class JAbstractModule implements JModule {
private static final JLog logger = JLogFactory.getLog(JAbstractModule.class);
/** 模块的配置信息 */
protected final JModuleConfig moduleConfig;
protected JAction defaultAction;
/** 模块中的Action */
protected Map actions;
public JAbstractModule(JModuleConfig moduleConfig) {
JAssert.checkNotNull(moduleConfig, "moduleConfig must not be null");
this.moduleConfig = moduleConfig;
this.actions = new HashMap();
}
@Override
public Set getActionNames() {
return this.actions.keySet();
}
@Override
public JAction findAction(String name) {
return this.actions.get(name);
}
@Override
public JAction getDefaultAction() {
return defaultAction;
}
@Override
public Object doAction(String actionName, Object in) {
JAssert.checkNotNull(actionName, "actionName must not be null");
return JModuleUtils.doActionWithinModuleClassLoader(this.actions.get(actionName), in);
}
@Override
public boolean hasAction(String actionName) {
return this.actions.containsKey(actionName);
}
@Override
public Object doDefaultAction(Object in) {
return JModuleUtils.doActionWithinModuleClassLoader(this.defaultAction, in);
}
@Override
public boolean hasDefaultAction() {
return this.defaultAction != null;
}
@Override
public void destroy() {
if (!actions.isEmpty()) {
actions.clear();
}
this.defaultAction = null;
Introspector.flushCaches();
ClassLoader classLoader = getModuleClassLoader();
if (classLoader == null) {
throw new NullPointerException("classLoader is null");
}
// 从已经使用给定类加载器加载的缓存中移除所有资源包
ResourceBundle.clearCache(classLoader);
if (classLoader instanceof JModuleClassLoader) {
try {
((JModuleClassLoader) classLoader).close();
} catch (IOException e) {
logger.error("close classLoader error.", e);
}
}
}
@Override
public JModuleConfig getModuleConfig() {
return moduleConfig;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy