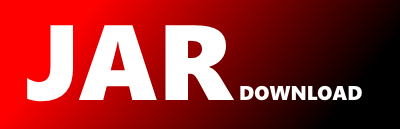
com.jianggujin.modulelink.impl.JRuntimeModule Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.impl;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.jianggujin.modulelink.JModule;
import com.jianggujin.modulelink.JModuleConfig;
import com.jianggujin.modulelink.JModuleLinkException;
import com.jianggujin.modulelink.util.JAssert;
import com.jianggujin.modulelink.util.JStringUtils;
/**
* 运行的模块
*
* @author jianggujin
*
*/
public class JRuntimeModule {
private final String name;
private String defaultVersion;
private Object moduleObj;
public JRuntimeModule(String name) {
this.name = name;
}
/**
* 查找指定版本模块
*
* @param version
* @return
*/
public JModule findModule(String version) {
return findModule0(version);
}
/**
* 查找模块
*
* @param version
* @return
*/
private JModule findModule0(String version) {
if (this.moduleObj != null) {
if (this.moduleObj instanceof JModule) {
JModule module = (JModule) this.moduleObj;
return module.getModuleConfig().getVersion().equals(version) ? module : null;
} else {
@SuppressWarnings("unchecked")
Map modules = (Map) moduleObj;
return modules.get(version);
}
}
return null;
}
/**
* 判断是否有指定版本
*
* @param version
* @return
*/
public boolean hasVersion(String version) {
if (version == null)
return false;
return findModule0(version) != null;
}
/**
* 获得默认模块
*
* @return
*/
public JModule getDefaultModule() {
return findModule0(defaultVersion);
}
/**
* 添加模块
*
* @param module
*/
public void add(JModule module) {
JModuleConfig moduleConfig = module.getModuleConfig();
String name = moduleConfig.getName(), version = moduleConfig.getVersion();
// 同样的模块名称与版本不允许注册
JAssert.checkState(name.equals(this.name) && !hasVersion(moduleConfig.getVersion()),
"duplicated module, name:%s, version %s", name, version);
if (this.moduleObj == null) {
this.moduleObj = module;
this.defaultVersion = version;
} else if (this.moduleObj instanceof JModule) {
JModule old = (JModule) this.moduleObj;
Map modules = new HashMap();
modules.put(old.getModuleConfig().getVersion(), old);
modules.put(version, module);
this.moduleObj = modules;
} else {
@SuppressWarnings("unchecked")
Map modules = (Map) moduleObj;
modules.put(version, module);
}
if (moduleConfig.isActive()) {
this.defaultVersion = version;
}
}
public JModule remove(String version) {
// 只有一个模块
if (this.moduleObj instanceof JModule) {
if (this.defaultVersion.equals(version)) {
this.defaultVersion = null;
JModule module = (JModule) this.moduleObj;
this.moduleObj = null;
return module;
}
return null;
}
if (this.moduleObj instanceof Map, ?>) {
@SuppressWarnings("unchecked")
Map modules = (Map) moduleObj;
if (modules.size() > 2 && JStringUtils.equals(version, defaultVersion))
throw new JModuleLinkException("module has version more than 2, default version could not allow remove.");
JModule module = modules.remove(version);
// 如果还剩一个默认的版本,则更换moduleObj的值
if (modules.size() == 1) {
this.moduleObj = modules.remove(defaultVersion);
}
return module;
}
return null;
}
public String getName() {
return name;
}
public String getDefaultVersion() {
return defaultVersion;
}
public void setDefaultVersion(String defaultVersion) {
JAssert.checkState(hasVersion(defaultVersion), "could not found version:" + defaultVersion);
this.defaultVersion = defaultVersion;
}
public JRuntimeModule withDefaultVersion(String defaultVersion) {
setDefaultVersion(defaultVersion);
return this;
}
private Collection list0() {
if (this.moduleObj == null) {
return null;
} else if (this.moduleObj instanceof JModule) {
List list = new ArrayList();
list.add((JModule) this.moduleObj);
return list;
} else {
@SuppressWarnings("unchecked")
Map modules = (Map) moduleObj;
return modules.values();
}
}
/**
* 获得所有模块
*
* @return
*/
public Collection list() {
return list0();
}
/**
* 获得所有模块
*
* @return
*/
public int count() {
return this.moduleObj == null ? 0 : (this.moduleObj instanceof JModule ? 1 : ((Map, ?>) this.moduleObj).size());
}
/**
* 清空
*
* @return
*/
public Collection clear() {
this.defaultVersion = null;
Collection list = list0();
if (this.moduleObj instanceof Map, ?>) {
@SuppressWarnings("unchecked")
Map modules = (Map) moduleObj;
modules.clear();
}
this.moduleObj = null;
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy