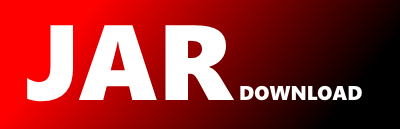
com.jianggujin.modulelink.impl.spring.JSpringModuleConfig Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.impl.spring;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.jianggujin.modulelink.JModuleConfig;
import com.jianggujin.modulelink.util.JAssert;
/**
* Spring模块配置
*
* @author jianggujin
*
*/
public class JSpringModuleConfig extends JModuleConfig {
/**
* 模块里的bean需要的配置信息,集成了spring properties
*/
private Map properties = new HashMap();
/**
* XML配置
*/
private Set xmlPatterns = new HashSet();
/**
* 需要排除的配置文件
*/
private Set exclusions = new HashSet();
public JSpringModuleConfig(String name, String version) {
super(name, version);
}
public JSpringModuleConfig(JModuleConfig moduleConfig) {
super(moduleConfig.getName(), moduleConfig.getName());
this.setDesc(moduleConfig.getDesc());
this.setOverridePackages(moduleConfig.getOverridePackages());
this.setModuleUrls(moduleConfig.getModuleUrls());
this.setScanPackages(moduleConfig.getScanPackages());
this.setActions(moduleConfig.getActions());
this.setExclusionActions(moduleConfig.getExclusionActions());
this.setActive(moduleConfig.isActive());
}
public Map getProperties() {
return properties;
}
public void setProperties(Map properties) {
JAssert.checkNotNull(properties, "properties must not be null");
this.properties = properties;
}
public JSpringModuleConfig withProperties(Map properties) {
this.setProperties(properties);
return this;
}
public JSpringModuleConfig addProperties(Map extends String, ? extends Object> properties) {
if (properties != null) {
this.properties.putAll(properties);
}
return this;
}
public JSpringModuleConfig addProperty(String name, Object value) {
JAssert.checkNotNull(name, "name must not be null");
JAssert.checkNotNull(value, "value must not be null");
this.properties.put(name, value);
return this;
}
public JSpringModuleConfig removeProperty(String name) {
this.properties.remove(name);
return this;
}
public Set getXmlPatterns() {
return xmlPatterns;
}
public void setXmlPatterns(Set xmlPatterns) {
JAssert.checkNotNull(xmlPatterns, "xmlPatterns must not be null");
this.xmlPatterns = xmlPatterns;
}
public JSpringModuleConfig withXmlPatterns(Set xmlPatterns) {
this.setXmlPatterns(xmlPatterns);
return this;
}
public JSpringModuleConfig addXmlPatterns(Collection extends String> xmlPatterns) {
if (xmlPatterns != null) {
this.xmlPatterns.addAll(xmlPatterns);
}
return this;
}
public JSpringModuleConfig addXmlPattern(String xmlPattern) {
JAssert.checkNotNull(xmlPattern, "xmlPattern must not be null");
this.xmlPatterns.add(xmlPattern);
return this;
}
public JSpringModuleConfig removeXmlPattern(String xmlPattern) {
this.xmlPatterns.remove(xmlPattern);
return this;
}
public Set getExclusions() {
return exclusions;
}
public void setExclusions(Set exclusions) {
JAssert.checkNotNull(exclusions, "exclusions must not be null");
this.exclusions = exclusions;
}
public JSpringModuleConfig withExclusions(Set exclusions) {
this.setExclusions(exclusions);
return this;
}
public JSpringModuleConfig addExclusions(Collection extends String> exclusions) {
if (exclusions != null) {
this.exclusions.addAll(exclusions);
}
return this;
}
public JSpringModuleConfig addExclusion(String exclusion) {
JAssert.checkNotNull(exclusion, "exclusion must not be null");
this.exclusions.add(exclusion);
return this;
}
public JSpringModuleConfig removeExclusion(String exclusion) {
this.exclusions.remove(exclusion);
return this;
}
/**
* 获得模块地址路径
*
* @return
*/
public List getModuleUrlPath() {
List moduleUrls = new ArrayList();
for (URL url : this.getModuleUrls()) {
moduleUrls.add(url.toString());
}
return moduleUrls;
}
@Override
public String toString() {
return "JSpringModuleConfig [properties=" + properties + ", xmlPatterns=" + xmlPatterns + ", exclusions="
+ exclusions + ", toString()=" + super.toString() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy