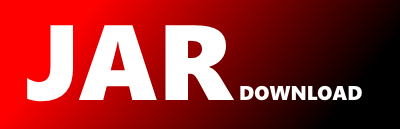
com.jianggujin.modulelink.util.JDefaultMultiValueMap Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.util;
import java.io.Serializable;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* 默认使用{@link LinkedHashMap}实现的多值集合
*
* @author jianggujin
*
* @param
* @param
*/
@SuppressWarnings("serial")
public class JDefaultMultiValueMap implements JMultiValueMap, Serializable {
private final Map> targetMap;
public JDefaultMultiValueMap() {
this.targetMap = new LinkedHashMap>();
}
public JDefaultMultiValueMap(int initialCapacity) {
this.targetMap = new LinkedHashMap>(initialCapacity);
}
public JDefaultMultiValueMap(Map> otherMap) {
this(otherMap, false);
}
/**
* 可以覆盖默认的{@link LinkedHashMap}实现
*
* @param otherMap
* @param overrideDefault
*/
public JDefaultMultiValueMap(Map> otherMap, boolean overrideDefault) {
JAssert.checkNotNull(otherMap, "otherMap must not be null.");
this.targetMap = overrideDefault ? otherMap : new LinkedHashMap>(otherMap);
}
@Override
public void add(K key, V value) {
List values = this.targetMap.get(key);
if (values == null) {
values = new LinkedList();
this.targetMap.put(key, values);
}
values.add(value);
}
@Override
public V getFirst(K key) {
List values = this.targetMap.get(key);
return (values != null ? values.get(0) : null);
}
@Override
public void set(K key, V value) {
List values = new LinkedList();
values.add(value);
this.targetMap.put(key, values);
}
@Override
public void setAll(Map values) {
for (Entry entry : values.entrySet()) {
set(entry.getKey(), entry.getValue());
}
}
@Override
public Map toSingleValueMap() {
LinkedHashMap singleValueMap = new LinkedHashMap(this.targetMap.size());
for (Entry> entry : targetMap.entrySet()) {
singleValueMap.put(entry.getKey(), entry.getValue().get(0));
}
return singleValueMap;
}
@Override
public int size() {
return this.targetMap.size();
}
@Override
public boolean isEmpty() {
return this.targetMap.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return this.targetMap.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return this.targetMap.containsValue(value);
}
@Override
public List get(Object key) {
return this.targetMap.get(key);
}
@Override
public List put(K key, List value) {
return this.targetMap.put(key, value);
}
@Override
public List remove(Object key) {
return this.targetMap.remove(key);
}
@Override
public void putAll(Map extends K, ? extends List> m) {
this.targetMap.putAll(m);
}
@Override
public void clear() {
this.targetMap.clear();
}
@Override
public Set keySet() {
return this.targetMap.keySet();
}
@Override
public Collection> values() {
return this.targetMap.values();
}
@Override
public Set>> entrySet() {
return this.targetMap.entrySet();
}
@Override
public boolean equals(Object obj) {
return this.targetMap.equals(obj);
}
@Override
public int hashCode() {
return this.targetMap.hashCode();
}
@Override
public String toString() {
return this.targetMap.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy