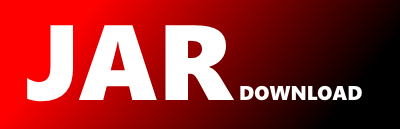
com.jianggujin.modulelink.util.JLogFactory Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.util;
import java.lang.reflect.Constructor;
/**
* 日志
*
* @author jianggujin
*
*/
public final class JLogFactory {
private static Constructor extends JLog> logConstructor;
static {
tryImplementation(new Runnable() {
public void run() {
setImplementation(JLoggingAdapter.class);
}
});
tryImplementation(new Runnable() {
public void run() {
setImplementation(JStdOutImpl.class);
}
});
}
private JLogFactory() {
}
public static JLog getLog(Class> aClass) {
return getLog(aClass.getName());
}
public static JLog getLog(String logger) {
try {
return logConstructor.newInstance(logger);
} catch (Throwable t) {
throw new JLogException("Error creating logger for logger " + logger + ". Cause: " + t, t);
}
}
private static void tryImplementation(Runnable runnable) {
if (logConstructor == null) {
try {
runnable.run();
} catch (Throwable t) {
// ignore
}
}
}
public static void setImplementation(Class extends JLog> implClass) {
try {
Constructor extends JLog> candidate = implClass.getConstructor(String.class);
JLog log = candidate.newInstance(JLogFactory.class.getName());
if (log.isDebugEnabled()) {
log.debug("Logging initialized using '" + implClass + "' adapter.");
}
logConstructor = candidate;
} catch (Throwable t) {
throw new JLogException("Error setting Log implementation. Cause: " + t, t);
}
}
/**
* 日志接口
*
* @author jianggujin
*
*/
public static interface JLog {
/**
* 错误开启
*
* @return
*/
public boolean isErrorEnabled();
/**
* 信息开启
*
* @return
*/
public boolean isInfoEnabled();
/**
* 警告开启
*
* @return
*/
public boolean isWarnEnabled();
/**
* 调试开启
*
* @return
*/
public boolean isDebugEnabled();
/**
* 跟踪开启
*
* @return
*/
public boolean isTraceEnabled();
/**
* 错误
*
* @param s
*/
public void error(String s);
/**
* 错误
*
* @param s
* @param e
*/
public void error(String s, Throwable e);
/**
* 调试
*
* @param s
*/
public void debug(String s);
/**
* 调试
*
* @param s
* @param e
*/
public void debug(String s, Throwable e);
/**
* 跟踪
*
* @param s
*/
public void trace(String s);
/**
* 跟踪
*
* @param s
* @param e
*/
public void trace(String s, Throwable e);
/**
* 警告
*
* @param s
*/
public void warn(String s);
/**
* 警告
*
* @param s
* @param e
*/
public void warn(String s, Throwable e);
/**
* 信息
*
* @param s
*/
public void info(String s);
/**
* 信息
*
* @param s
* @param e
*/
public void info(String s, Throwable e);
}
public static class JStdOutImpl implements JLog {
public JStdOutImpl(String clazz) {
// Do Nothing
}
public boolean isDebugEnabled() {
return true;
}
public boolean isTraceEnabled() {
return true;
}
public void error(String s, Throwable e) {
System.err.println(s);
e.printStackTrace(System.err);
}
public void error(String s) {
System.err.println(s);
}
public void debug(String s) {
System.out.println(s);
}
public void trace(String s) {
System.out.println(s);
}
public void warn(String s) {
System.out.println(s);
}
public void info(String s) {
System.out.println(s);
}
public void debug(String s, Throwable e) {
System.out.println(s);
e.printStackTrace(System.out);
}
public void trace(String s, Throwable e) {
System.out.println(s);
e.printStackTrace(System.out);
}
public void warn(String s, Throwable e) {
System.out.println(s);
e.printStackTrace(System.out);
}
public void info(String s, Throwable e) {
System.out.println(s);
e.printStackTrace(System.out);
}
public boolean isErrorEnabled() {
return true;
}
public boolean isInfoEnabled() {
return true;
}
public boolean isWarnEnabled() {
return true;
}
}
public static class JLogException extends RuntimeException {
private static final long serialVersionUID = 1L;
public JLogException() {
super();
}
public JLogException(String message) {
super(message);
}
public JLogException(String message, Throwable cause) {
super(message, cause);
}
public JLogException(Throwable cause) {
super(cause);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy