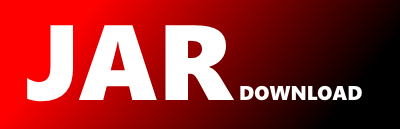
com.jianggujin.modulelink.util.JStringUtils Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.util;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.Map;
/**
* 字符串工具
*
* @author jianggujin
*
*/
public class JStringUtils {
public static final int INDEX_NOT_FOUND = -1;
public static final String[] EMPTY_STRING_ARRAY = new String[0];
public static boolean isBlank(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; i++) {
if ((Character.isWhitespace(str.charAt(i)) == false)) {
return false;
}
}
return true;
}
public static boolean equals(String str1, String str2) {
return str1 == null ? str2 == null : str1.equals(str2);
}
public static String reflectionToString(Throwable t) {
StringWriter sw = new StringWriter();
PrintWriter writer = new PrintWriter(sw);
t.printStackTrace(writer);
writer.close();
return sw.toString();
}
/**
* 首字母变小写
*/
public static String firstCharToLowerCase(String str) {
char firstChar = str.charAt(0);
if (firstChar >= 'A' && firstChar <= 'Z') {
char[] arr = str.toCharArray();
arr[0] += ('a' - 'A');
return new String(arr);
}
return str;
}
/**
* 首字母变大写
*/
public static String firstCharToUpperCase(String str) {
char firstChar = str.charAt(0);
if (firstChar >= 'a' && firstChar <= 'z') {
char[] arr = str.toCharArray();
arr[0] -= ('a' - 'A');
return new String(arr);
}
return str;
}
public static boolean notBlank(String str) {
return !isBlank(str);
}
public static boolean notBlank(String... strings) {
if (strings == null || strings.length == 0) {
return false;
}
for (String str : strings) {
if (isBlank(str)) {
return false;
}
}
return true;
}
public static boolean notNull(Object... paras) {
if (paras == null) {
return false;
}
for (Object obj : paras) {
if (obj == null) {
return false;
}
}
return true;
}
public static String toCamelCase(String stringWithUnderline) {
if (stringWithUnderline.indexOf('_') == -1) {
return stringWithUnderline;
}
stringWithUnderline = stringWithUnderline.toLowerCase();
char[] fromArray = stringWithUnderline.toCharArray();
char[] toArray = new char[fromArray.length];
int j = 0;
for (int i = 0; i < fromArray.length; i++) {
if (fromArray[i] == '_') {
// 当前字符为下划线时,将指针后移一位,将紧随下划线后面一个字符转成大写并存放
i++;
if (i < fromArray.length) {
toArray[j++] = Character.toUpperCase(fromArray[i]);
}
} else {
toArray[j++] = fromArray[i];
}
}
return new String(toArray, 0, j);
}
public static String join(String[] stringArray) {
StringBuilder sb = new StringBuilder();
for (String s : stringArray) {
sb.append(s);
}
return sb.toString();
}
public static String join(String[] stringArray, String separator) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < stringArray.length; i++) {
if (i > 0) {
sb.append(separator);
}
sb.append(stringArray[i]);
}
return sb.toString();
}
public static String getRandomUUID() {
return java.util.UUID.randomUUID().toString().replace("-", "");
}
public static String toString(Object obj) {
return obj == null ? null : obj.toString();
}
/**
* 用提供的数据替换掉字符串中内容
*
* @param text
* @param bindRes
* @return
*/
public static String resolveDynamicPropnames(String text, Map bindRes) {
return resolveDynamicPropnames(text, bindRes, "${", "}");
}
/**
* 用提供的数据替换掉字符串中内容
*
* @param text
* @param bindRes
* @param prefix
* 插值前缀
* @param suffix
* 插值后缀
* @return
*/
public static String resolveDynamicPropnames(String text, Map bindRes, String prefix,
String suffix) {
if (text == null) {
return text;
}
int startIndex = text.indexOf(prefix);
if (startIndex == -1) {
return text;
}
String tempStr = text;
StringBuilder result = new StringBuilder(text.length() + 32);
int prefixLength = prefix.length();
while (startIndex != -1) {
result.append(tempStr.substring(0, startIndex));
int endIndex = tempStr.indexOf(suffix, startIndex + prefixLength);
if (endIndex != -1) {
String dName = tempStr.substring(startIndex + prefixLength, endIndex);
try {
String pValue = null;
if (bindRes != null) {
Object obj = bindRes.get(dName);
if (obj != null) {
pValue = String.valueOf(obj);
}
}
if (pValue != null) {
result.append(resolveDynamicPropnames(pValue, bindRes));
} else {
result.append(tempStr.substring(startIndex, endIndex + 1));
}
} catch (Throwable ex) {
}
tempStr = tempStr.substring(endIndex + suffix.length());
startIndex = tempStr.indexOf(prefix);
} else {
tempStr = tempStr.substring(startIndex);
startIndex = -1;
}
}
result.append(tempStr);
return result.toString();
}
public static String[] addStringToArray(String[] array, String str) {
if (array == null || array.length == 0) {
return new String[] { str };
}
String[] newArr = new String[array.length + 1];
System.arraycopy(array, 0, newArr, 0, array.length);
newArr[array.length] = str;
return newArr;
}
public static boolean isEmpty(String str) {
return str == null || str.length() == 0;
}
public static int indexOf(String str, char searchChar, int startPos) {
if (isEmpty(str)) {
return INDEX_NOT_FOUND;
}
return str.indexOf(searchChar, startPos);
}
public static String[] separateString(String str, String delimiter) {
return separateString(str, delimiter, false);
}
public static String[] separateString(String str, String delimiter, boolean trim) {
if (str == null) {
return null;
} else {
int count = str.length();
if (count == 0) {
return EMPTY_STRING_ARRAY;
} else {
if (isEmpty(delimiter)) {
delimiter = " \t\n\r\f";
}
ArrayList list = new ArrayList();
int i = 0;
int begin = 0;
boolean notMatch = false;
if (delimiter.length() == 1) {
char c = delimiter.charAt(0);
while (i < count) {
if (str.charAt(i) == c) {
if (notMatch) {
list.add(trim ? str.substring(begin, i).trim() : str.substring(begin, i));
notMatch = false;
}
++i;
begin = i;
} else {
notMatch = true;
++i;
}
}
} else {
while (i < count) {
if (delimiter.indexOf(str.charAt(i)) >= 0) {
if (notMatch) {
list.add(trim ? str.substring(begin, i).trim() : str.substring(begin, i));
notMatch = false;
}
++i;
begin = i;
} else {
notMatch = true;
++i;
}
}
}
if (notMatch) {
list.add(trim ? str.substring(begin, i).trim() : str.substring(begin, i));
}
return (String[]) list.toArray(new String[list.size()]);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy