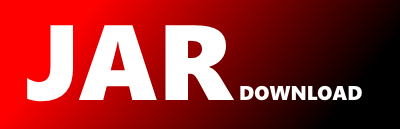
com.jianggujin.modulelink.util.JThreadFactory Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.util;
import java.io.Serializable;
import java.util.concurrent.ThreadFactory;
/**
* 线程工厂
*
* @author jianggujin
*
*/
@SuppressWarnings("serial")
public class JThreadFactory implements ThreadFactory, Serializable {
/**
* 线程名称前缀
*/
private String threadNamePrefix;
/**
* 线程优先级
*/
private int threadPriority = Thread.NORM_PRIORITY;
/**
* 守护线程
*/
private boolean daemon = false;
/**
* 线程组
*/
private ThreadGroup threadGroup;
/**
* 线程个数
*/
private int threadCount = 0;
/**
* 线程个数监视器
*/
private final Object threadCountMonitor = new Object();
public JThreadFactory() {
this.threadNamePrefix = getDefaultThreadNamePrefix();
}
public JThreadFactory(String threadNamePrefix) {
this.threadNamePrefix = (threadNamePrefix != null ? threadNamePrefix : getDefaultThreadNamePrefix());
}
public Thread newThread(Runnable runnable) {
return createThread(runnable);
}
/**
* 设置线程名称前缀
*
* @param threadNamePrefix
*/
public void setThreadNamePrefix(String threadNamePrefix) {
this.threadNamePrefix = (threadNamePrefix != null ? threadNamePrefix : getDefaultThreadNamePrefix());
}
/**
* 获得线程名称前缀
*
* @return
*/
public String getThreadNamePrefix() {
return this.threadNamePrefix;
}
/**
* 设置线程优先级,默认为{@link Thread#NORM_PRIORITY}
*
* @param threadPriority
*/
public void setThreadPriority(int threadPriority) {
this.threadPriority = threadPriority;
}
/**
* 获得线程优先级
*
* @return
*/
public int getThreadPriority() {
return this.threadPriority;
}
/**
* 设置是否应该创建守护线程
*
* @param daemon
*/
public void setDaemon(boolean daemon) {
this.daemon = daemon;
}
/**
* 获得是否应该创建守护线程
*
* @return
*/
public boolean isDaemon() {
return this.daemon;
}
/**
* 指定要创建的线程的线程组名称
*
* @param name
*/
public void setThreadGroupName(String name) {
this.threadGroup = new ThreadGroup(name);
}
/**
* 指定要创建的线程的线程组
*
* @param threadGroup
*/
public void setThreadGroup(ThreadGroup threadGroup) {
this.threadGroup = threadGroup;
}
/**
* 获得线程组
*
* @return
*/
public ThreadGroup getThreadGroup() {
return this.threadGroup;
}
/**
* 创建线程
*
* @param runnable
* @return
*/
public Thread createThread(Runnable runnable) {
Thread thread = new Thread(getThreadGroup(), runnable, nextThreadName());
thread.setPriority(getThreadPriority());
thread.setDaemon(isDaemon());
return thread;
}
/**
* 获得下一个线程名称
*
* @return
*/
protected String nextThreadName() {
int threadNumber = 0;
synchronized (this.threadCountMonitor) {
this.threadCount++;
threadNumber = this.threadCount;
}
return getThreadNamePrefix() + threadNumber;
}
/**
* 获得默认线程名称前缀
*
* @return
*/
protected String getDefaultThreadNamePrefix() {
return "JMuduleLink-";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy