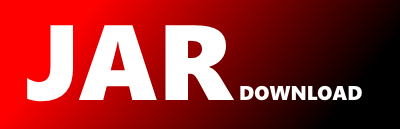
com.jianggujin.modulelink.util.vfs.JJBoss6VFS Maven / Gradle / Ivy
/**
* Copyright 2018 jianggujin (www.jianggujin.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jianggujin.modulelink.util.vfs;
import java.io.IOException;
import java.lang.reflect.Method;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* 一个用于JBoss6的{@link JVFS }实现
*
* @author jianggujin
*
*/
public class JJBoss6VFS extends JVFS {
/**
* 模仿JBoss VirtualFile类的精简实现
*
* @author jianggujin
*
*/
static class VirtualFile {
static Class> VirtualFile;
static Method getPathNameRelativeTo, getChildrenRecursively;
Object virtualFile;
VirtualFile(Object virtualFile) {
this.virtualFile = virtualFile;
}
String getPathNameRelativeTo(VirtualFile parent) {
try {
return invoke(getPathNameRelativeTo, virtualFile, parent.virtualFile);
} catch (IOException e) {
return null;
}
}
List getChildren() throws IOException {
List> objects = invoke(getChildrenRecursively, virtualFile);
List children = new ArrayList(objects.size());
for (Object object : objects) {
children.add(new VirtualFile(object));
}
return children;
}
}
/**
* 模仿JBoss VFS类的精简实现
*
* @author jianggujin
*
*/
static class VFS {
static Class> VFS;
static Method getChild;
private VFS() {
}
static VirtualFile getChild(URL url) throws IOException {
Object o = invoke(getChild, VFS, url);
return o == null ? null : new VirtualFile(o);
}
}
/**
* 表示VFS当前的环境是否有效
*/
private static Boolean valid;
/**
* 找到所有需要访问JBoss 6 VFS的类和方法
*/
protected static synchronized void initialize() {
if (valid == null) {
// 假设有效。如果出错,它会被翻转
valid = Boolean.TRUE;
// 查找和验证所需的类
VFS.VFS = checkNotNull(getClass("org.jboss.vfs.VFS"));
VirtualFile.VirtualFile = checkNotNull(getClass("org.jboss.vfs.VirtualFile"));
// 查找和验证所需的方法
VFS.getChild = checkNotNull(getMethod(VFS.VFS, "getChild", URL.class));
VirtualFile.getChildrenRecursively = checkNotNull(
getMethod(VirtualFile.VirtualFile, "getChildrenRecursively"));
VirtualFile.getPathNameRelativeTo = checkNotNull(
getMethod(VirtualFile.VirtualFile, "getPathNameRelativeTo", VirtualFile.VirtualFile));
// 验证API没有改变
checkReturnType(VFS.getChild, VirtualFile.VirtualFile);
checkReturnType(VirtualFile.getChildrenRecursively, List.class);
checkReturnType(VirtualFile.getPathNameRelativeTo, String.class);
}
}
/**
* 验证所提供的对象引用是否为null。如果它是空的,那么VFS在当前环境终将被标记为无效
*
* @param object
* @return
*/
protected static T checkNotNull(T object) {
if (object == null) {
setInvalid();
}
return object;
}
/**
* 验证方法的返回类型是期望的类型。如果不是,那么VFS在当前环境终将被标记为无效
*
* @param method
* @param expected
*/
protected static void checkReturnType(Method method, Class> expected) {
if (method != null && !expected.isAssignableFrom(method.getReturnType())) {
setInvalid();
}
}
/**
* 标记VFS在当前环境是无效的
*/
protected static void setInvalid() {
if (JJBoss6VFS.valid == Boolean.TRUE) {
JJBoss6VFS.valid = Boolean.FALSE;
}
}
static {
initialize();
}
@Override
public boolean isValid() {
return valid;
}
@Override
public List list(URL url, String path) throws IOException {
VirtualFile directory;
directory = VFS.getChild(url);
if (directory == null) {
return Collections.emptyList();
}
if (!path.endsWith("/")) {
path += "/";
}
List children = directory.getChildren();
List names = new ArrayList(children.size());
for (VirtualFile vf : children) {
names.add(path + vf.getPathNameRelativeTo(directory));
}
return names;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy