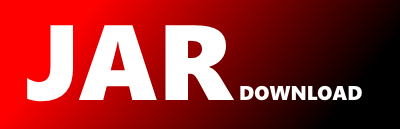
com.jidesoft.swing.JToolTipFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jide-oss Show documentation
Show all versions of jide-oss Show documentation
JIDE Common Layer (Professional Swing Components)
/*
* @(#)JiToolTipFactory.java 4/25/2008
*
* Copyright 2002 - 2005 JIDE Software Inc. All rights reserved.
*/
package com.jidesoft.swing;
import javax.swing.*;
import java.awt.*;
/**
* This class creates instances of JTooltip components. It provides a consistent means for creating custom tooltips
* without the need for overriding JIDE components that create tooltips
*/
public class JToolTipFactory {
private static JToolTipFactory _tooltipFactory;
/**
* Creates a new tooltip.
*
* @param c the component the tooltip describes
* @return the new tooltip object
*/
public JToolTip createToolTip(JComponent c) {
return createToolTip(c, false);
}
/**
* Creates a new tooltip. If overlapping is true then the tooltip will take on the foreground/background color and
* font of the specified component (if the component isspecifiedd)
*
* @param c the component the tooltip describes
* @param overlapping whether the tooltip is for a normal or overlapping tooltip
* @return the new tooltip object
*/
public JToolTip createToolTip(JComponent c, boolean overlapping) {
JToolTip tt = new JToolTip();
if (c != null) {
tt.setComponent(c);
if (overlapping) {
if (c.getBackground() != null) {
Color bg = c.getBackground();
if (bg.getAlpha() != 255) {
bg = new Color(bg.getRed(), bg.getGreen(), bg.getBlue());
}
tt.setBackground(bg);
}
if (c.getForeground() != null) {
tt.setForeground(c.getForeground());
}
if (c.getFont() != null) {
tt.setFont(c.getFont());
}
}
}
return tt;
}
/**
* Sets the JToolTipFactory
that will be used to obtain JToolTip
s. This will throw an
* IllegalArgumentException
if factory
is null.
*
* @param factory the shared factory
* @throws IllegalArgumentException if factory
is null
*/
public static void setSharedInstance(JToolTipFactory factory) {
if (factory == null) {
throw new IllegalArgumentException("JToolTipFactory can not be null");
}
_tooltipFactory = factory;
}
/**
* Returns the shared JToolTipFactory
which can be used to obtain JToolTip
s.
*
* @return the shared factory
*/
public static JToolTipFactory getSharedInstance() {
if (_tooltipFactory == null) {
_tooltipFactory = new JToolTipFactory();
}
return _tooltipFactory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy