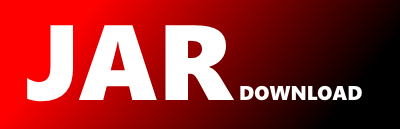
com.jidesoft.swing.ShadowFactory Maven / Gradle / Ivy
Show all versions of jide-oss Show documentation
/*
* @(#)ShadowFactory.java 9/14/2011
*
* Copyright 2002 - 2011 JIDE Software Inc. All rights reserved.
*/
package com.jidesoft.swing;
import java.awt.*;
import java.awt.image.*;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeSupport;
import java.util.HashMap;
/**
* A shadow factory generates a drop shadow for any given picture, respecting the transparency channel if present.
* The resulting picture contains the shadow only and to create a drop shadow effect you will need to stack the original
* picture and the shadow generated by the factory.
Shadow Properties
A shadow is defined by three
* properties:
- size: The size, in pixels, of the shadow. This property also defines the fuzzyness.
* - opacity: The opacity, between 0.0 and 1.0, of the shadow.
- color: The color of the shadow.
* Shadows are not meant to be black only.
You can set these properties using the provided mutaters or the
* appropriate constructor. Here are two ways of creating a green shadow of size 10 and with an opacity of 50%:
*
* ShadowFactory factory = new ShadowFactory(10, 0.5f, Color.GREEN);
* // ..
* factory = new ShadowFactory();
* factory.setSize(10);
* factory.setOpacity(0.5f);
* factory.setColor(Color.GREEN);
*
* The default constructor provides the following default values: - size: 5 pixels
* - opacity: 50%
- color: Black
Shadow Quality
The factory provides
* two shadow generation algorithms: fast quality blur and high quality blur. You can select your
* preferred algorithm by setting the appropriate rendering hint:
*
* ShadowFactory factory = new ShadowFactory();
* factory.setRenderingHint(ShadowFactory.KEY_BLUR_QUALITY,
* ShadowFactory.VALUE_BLUR_QUALITY_HIGH);
*
* The default rendering algorithm is VALUE_BLUR_QUALITY_FAST
. The current implementation should
* provide the same quality with both algorithms but performances are guaranteed to be better (about 30 times faster)
* with the fast quality blur.
Generating a Shadow
A shadow is generated as a
* BufferedImage
from another BufferedImage
. Once the factory is set up, you must call {@link
* #createShadow} to actually generate the shadow:
*
* ShadowFactory factory = new ShadowFactory();
* // factory setup
* BufferedImage shadow = factory.createShadow(bufferedImage);
*
* The resulting image is of type BufferedImage.TYPE_INT_ARGB
. Both dimensions of this image are larger
* than original image's: - new width = original width + 2 * shadow size
- new height = original height +
* 2 * shadow size
This must be taken into account when you need to create a drop shadow effect.
* Properties Changes
This factory allows to register property change listeners with {@link
* #addPropertyChangeListener}. Listening to properties changes is very useful when you embed the factory in a graphical
* component and give the API user the ability to access the factory. By listening to properties changes, you can easily
* repaint the component when needed.
Threading Issues
ShadowFactory
is not guaranteed to
* be thread-safe.
*
* @author Romain Guy
* @author Sebastien Petrucci
*/
public class ShadowFactory {
/**
* Key for the blur quality rendering hint.
*/
public static final String KEY_BLUR_QUALITY = "blur_quality";
/**
* Selects the fast rendering algorithm. This is the default rendering hint for
* KEY_BLUR_QUALITY
.
*/
public static final String VALUE_BLUR_QUALITY_FAST = "fast";
/**
* Selects the high quality rendering algorithm. With current implementation, This algorithm does not guarantee a
* better rendering quality and should not be used.
*/
public static final String VALUE_BLUR_QUALITY_HIGH = "high";
/**
* Identifies a change to the size used to render the shadow.
When the property change event is fired, the
* old value and the new value are provided as Integer
instances.
*/
public static final String SIZE_CHANGED_PROPERTY = "shadow_size";
/**
* Identifies a change to the opacity used to render the shadow.
When the property change event is fired,
* the old value and the new value are provided as Float
instances.
*/
public static final String OPACITY_CHANGED_PROPERTY = "shadow_opacity";
/**
* Identifies a change to the color used to render the shadow.
*/
public static final String COLOR_CHANGED_PROPERTY = "shadow_color";
// size of the shadow in pixels (defines the fuzziness)
private int size = 5;
// opacity of the shadow
private float opacity = 0.5f;
// color of the shadow
private Color color = Color.BLACK;
// rendering hints map
private HashMap hints;
// notifies listeners of properties changes
private PropertyChangeSupport changeSupport;
/**
* Creates a default good looking shadow generator. The default shadow factory provides the following default
* values:
- size: 5 pixels
- opacity: 50%
- color: Black
* - rendering quality: VALUE_BLUR_QUALITY_FAST
These properties provide a regular, good
* looking shadow.
*/
public ShadowFactory() {
this(5, 0.5f, Color.BLACK);
}
/**
* A shadow factory needs three properties to generate shadows. These properties are:
- size:
* The size, in pixels, of the shadow. This property also defines the fuzzyness.
- opacity: The
* opacity, between 0.0 and 1.0, of the shadow.
- color: The color of the shadow. Shadows are not
* meant to be black only.
Besides these properties you can set rendering hints to control the
* rendering process. The default rendering hints let the factory use the fastest shadow generation algorithm.
*
* @param size The size of the shadow in pixels. Defines the fuzziness.
* @param opacity The opacity of the shadow.
* @param color The color of the shadow.
* @see #setRenderingHint(Object, Object)
*/
public ShadowFactory(final int size, final float opacity, final Color color) {
hints = new HashMap();
hints.put(KEY_BLUR_QUALITY, VALUE_BLUR_QUALITY_FAST);
changeSupport = new PropertyChangeSupport(this);
setSize(size);
setOpacity(opacity);
setColor(color);
}
/**
* Add a PropertyChangeListener to the listener list. The listener is registered for all properties. The same
* listener object may be added more than once, and will be called as many times as it is added. If
* listener
is null, no exception is thrown and no action is taken.
*
* @param listener the PropertyChangeListener to be added
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
changeSupport.addPropertyChangeListener(listener);
}
/**
* Remove a PropertyChangeListener from the listener list. This removes a PropertyChangeListener that was
* registered for all properties. If listener
was added more than once to the same event source, it
* will be notified one less time after being removed. If listener
is null, or was never added, no
* exception is thrown and no action is taken.
*
* @param listener
*/
public void removePropertyChangeListener(PropertyChangeListener listener) {
changeSupport.removePropertyChangeListener(listener);
}
/**
* Maps the specified rendering hint key
to the specified value
in this
* SahdowFactory
object.
*
* @param key The rendering hint key
* @param value The rendering hint value
*/
public void setRenderingHint(final Object key, final Object value) {
hints.put(key, value);
}
/**
* Gets the color used by the factory to generate shadows.
*
* @return this factory's shadow color
*/
public Color getColor() {
return color;
}
/**
* Sets the color used by the factory to generate shadows.
Consecutive calls to {@link #createShadow} will
* all use this color until it is set again.
If the color provided is null, the previous color will be
* retained.
*
* @param shadowColor the generated shadows color
*/
public void setColor(final Color shadowColor) {
if (shadowColor != null) {
Color oldColor = this.color;
this.color = shadowColor;
changeSupport.firePropertyChange(COLOR_CHANGED_PROPERTY,
oldColor,
this.color);
}
}
/**
* Gets the opacity used by the factory to generate shadows.
The opacity is comprised between 0.0f and
* 1.0f; 0.0f being fully transparent and 1.0f fully opaque.
*
* @return this factory's shadow opacity
*/
public float getOpacity() {
return opacity;
}
/**
* Sets the opacity used by the factory to generate shadows.
Consecutive calls to {@link #createShadow}
* will all use this color until it is set again.
The opacity is comprised between 0.0f and 1.0f; 0.0f being
* fully transparent and 1.0f fully opaque. If you provide a value out of these boundaries, it will be restrained to
* the closest boundary.
*
* @param shadowOpacity the generated shadows opacity
*/
public void setOpacity(final float shadowOpacity) {
float oldOpacity = this.opacity;
if (shadowOpacity < 0.0) {
this.opacity = 0.0f;
}
else if (shadowOpacity > 1.0f) {
this.opacity = 1.0f;
}
else {
this.opacity = shadowOpacity;
}
changeSupport.firePropertyChange(OPACITY_CHANGED_PROPERTY,
oldOpacity,
this.opacity);
}
/**
* Gets the size in pixel used by the factory to generate shadows.
*
* @return this factory's shadow size
*/
public int getSize() {
return size;
}
/**
* Sets the size, in pixels, used by the factory to generate shadows.
The size defines the blur radius
* applied to the shadow to create the fuzziness.
There is virtually no limit to the size but it has an
* impact on shadow generation performances. The greater this value, the longer it will take to generate the shadow.
* Remember the generated shadow image dimensions are computed as follow:
- new width = original width + 2 *
* shadow size
- new height = original height + 2 * shadow size
The size cannot be negative. If
* you provide a negative value, the size will be 0 instead.
*
* @param shadowSize the generated shadows size in pixels (fuzziness)
*/
public void setSize(final int shadowSize) {
int oldSize = this.size;
if (shadowSize < 0) {
this.size = 0;
}
else {
this.size = shadowSize;
}
changeSupport.firePropertyChange(SIZE_CHANGED_PROPERTY,
new Integer(oldSize),
new Integer(this.size));
}
/**
* Generates the shadow for a given picture and the current properties of the factory.
The generated
* shadow image dimensions are computed as follow:
- new width = original width + 2 * shadow size
* - new height = original height + 2 * shadow size
The time taken by a call to this method
* depends on the size of the shadow, the larger the longer it takes, and on the selected rendering algorithm.
*
* @param image the picture from which the shadow must be cast
* @return the picture containing the shadow of image
*/
public BufferedImage createShadow(final BufferedImage image) {
if (hints.get(KEY_BLUR_QUALITY) == VALUE_BLUR_QUALITY_HIGH) {
// the high quality algorithm is a 3-pass algorithm
// it goes through all the pixels of the original picture at least
// three times to generate the shadow
// it is easy to understand but very slow
BufferedImage subject = prepareImage(image);
BufferedImage shadow = new BufferedImage(subject.getWidth(),
subject.getHeight(),
BufferedImage.TYPE_INT_ARGB);
BufferedImage shadowMask = createShadowMask(subject);
getLinearBlurOp(size).filter(shadowMask, shadow);
return shadow;
}
// call the fast rendering algorithm
return createShadowFast(image);
}
// prepares the picture for the high quality rendering algorithm
private BufferedImage prepareImage(final BufferedImage image) {
BufferedImage subject = new BufferedImage(image.getWidth() + size * 2,
image.getHeight() + size * 2,
BufferedImage.TYPE_INT_ARGB);
Graphics2D g2 = subject.createGraphics();
g2.drawImage(image, null, size, size);
g2.dispose();
return subject;
}
// fast rendering algorithm
// basically applies duplicates the picture and applies a size*size kernel
// in only one pass.
// the kernel is simulated by an horizontal and a vertical pass
// implemented by Sebastien Petrucci
private BufferedImage createShadowFast(final BufferedImage src) {
int shadowSize = this.size;
int srcWidth = src.getWidth();
int srcHeight = src.getHeight();
int dstWidth = srcWidth + size;
int dstHeight = srcHeight + size;
int left = (shadowSize - 1) >> 1;
int right = shadowSize - left;
int yStop = dstHeight - right;
BufferedImage dst = new BufferedImage(dstWidth, dstHeight,
BufferedImage.TYPE_INT_ARGB);
int shadowRgb = color.getRGB() & 0x00FFFFFF;
int[] aHistory = new int[shadowSize];
int historyIdx;
int aSum;
ColorModel srcColorModel = src.getColorModel();
WritableRaster srcRaster = src.getRaster();
int[] dstBuffer = ((DataBufferInt) dst.getRaster().getDataBuffer()).getData();
int lastPixelOffset = right * dstWidth;
float hSumDivider = 1.0f / size;
float vSumDivider = opacity / size;
// horizontal pass : extract the alpha mask from the source picture and
// blur it into the destination picture
for (int srcY = 0, dstOffset = left * dstWidth; srcY < srcHeight; srcY++) {
// first pixels are empty
for (historyIdx = 0; historyIdx < shadowSize; ) {
aHistory[historyIdx++] = 0;
}
aSum = 0;
historyIdx = 0;
// compute the blur average with pixels from the source image
for (int srcX = 0; srcX < srcWidth; srcX++) {
int a = (int) (aSum * hSumDivider); // calculate alpha value
dstBuffer[dstOffset++] = a << 24; // store the alpha value only
// the shadow color will be added in the next pass
aSum -= aHistory[historyIdx]; // subtract the oldest pixel from the sum
// extract the new pixel ...
a = srcColorModel.getAlpha(srcRaster.getDataElements(srcX, srcY, null));
aHistory[historyIdx] = a; // ... and store its value into history
aSum += a; // ... and add its value to the sum
if (++historyIdx >= shadowSize) {
historyIdx -= shadowSize;
}
}
// blur the end of the row - no new pixels to grab
for (int i = 0; i < shadowSize; i++) {
int a = (int) (aSum * hSumDivider);
dstBuffer[dstOffset++] = a << 24;
// subtract the oldest pixel from the sum ... and nothing new to add !
aSum -= aHistory[historyIdx];
if (++historyIdx >= shadowSize) {
historyIdx -= shadowSize;
}
}
}
// vertical pass
for (int x = 0, bufferOffset = 0; x < dstWidth; x++, bufferOffset = x) {
aSum = 0;
// first pixels are empty
for (historyIdx = 0; historyIdx < left; ) {
aHistory[historyIdx++] = 0;
}
// and then they come from the dstBuffer
for (int y = 0; y < right; y++, bufferOffset += dstWidth) {
int a = dstBuffer[bufferOffset] >>> 24; // extract alpha
aHistory[historyIdx++] = a; // store into history
aSum += a; // and add to sum
}
bufferOffset = x;
historyIdx = 0;
// compute the blur average with pixels from the previous pass
for (int y = 0; y < yStop; y++, bufferOffset += dstWidth) {
int a = (int) (aSum * vSumDivider); // calculate alpha value
dstBuffer[bufferOffset] = a << 24 | shadowRgb; // store alpha value + shadow color
aSum -= aHistory[historyIdx]; // subtract the oldest pixel from the sum
a = dstBuffer[bufferOffset + lastPixelOffset] >>> 24; // extract the new pixel ...
aHistory[historyIdx] = a; // ... and store its value into history
aSum += a; // ... and add its value to the sum
if (++historyIdx >= shadowSize) {
historyIdx -= shadowSize;
}
}
// blur the end of the column - no pixels to grab anymore
for (int y = yStop; y < dstHeight; y++, bufferOffset += dstWidth) {
int a = (int) (aSum * vSumDivider);
dstBuffer[bufferOffset] = a << 24 | shadowRgb;
aSum -= aHistory[historyIdx]; // subtract the oldest pixel from the sum
if (++historyIdx >= shadowSize) {
historyIdx -= shadowSize;
}
}
}
return dst;
}
// creates the shadow mask for the original picture
// it colorize all the pixels with the shadow color according to their
// original transparency
private BufferedImage createShadowMask(final BufferedImage image) {
BufferedImage mask = new BufferedImage(image.getWidth(),
image.getHeight(),
BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = mask.createGraphics();
g2d.drawImage(image, 0, 0, null);
g2d.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_IN,
opacity));
g2d.setColor(color);
g2d.fillRect(0, 0, image.getWidth(), image.getHeight());
g2d.dispose();
return mask;
}
// creates a blur convolve operation by generating a kernel of
// dimensions (size, size).
private ConvolveOp getLinearBlurOp(final int size) {
float[] data = new float[size * size];
float value = 1.0f / (float) (size * size);
for (int i = 0; i < data.length; i++) {
data[i] = value;
}
return new ConvolveOp(new Kernel(size, size, data));
}
}