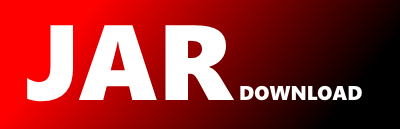
com.jidesoft.comparator.DefaultComparator Maven / Gradle / Ivy
package com.jidesoft.comparator;
import java.util.Comparator;
/**
* Badly named, this class compares objects by first converting them to Strings using the
* toString method.
*/
public class DefaultComparator implements Comparator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy