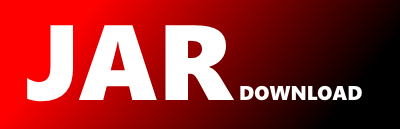
com.jidesoft.dialog.JideOptionPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jide-oss Show documentation
Show all versions of jide-oss Show documentation
JIDE Common Layer (Professional Swing Components)
/*
* @(#)JideOptionPane.java 3/27/2006
*
* Copyright 2002 - 2006 JIDE Software Inc. All rights reserved.
*/
package com.jidesoft.dialog;
import com.jidesoft.plaf.basic.BasicJideOptionPaneUI;
import com.jidesoft.swing.JideSwingUtilities;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Locale;
/**
* JideOptionPane
is an enhanced version of JOptionPane.
*
* This component is still in beta, thus we didn't include the UIDefault needed by this component into
* LookAndFeelFactory by default. If you want to use it, please refer to JideOptionPaneDemo's getDemoPanel method where
* we add all necessary UIDefaults using UIDefaultCustomizer.
*/
public class JideOptionPane extends JOptionPane {
private static final long serialVersionUID = 1916857052448620771L;
private Object _title;
private Object _details;
// private boolean _bannerVisible = true;
/**
* Bound property name for details
.
*/
public static final String DETAILS_PROPERTY = "details";
/**
* Bound property name for title
.
*/
public static final String TITLE_PROPERTY = "title";
public JideOptionPane() {
initComponents();
}
public JideOptionPane(Object message) {
super(message);
initComponents();
}
public JideOptionPane(Object message, int messageType) {
super(message, messageType);
initComponents();
}
public JideOptionPane(Object message, int messageType, int optionType) {
super(message, messageType, optionType);
initComponents();
}
public JideOptionPane(Object message, int messageType, int optionType, Icon icon) {
super(message, messageType, optionType, icon);
initComponents();
}
public JideOptionPane(Object message, int messageType, int optionType, Icon icon, Object[] options) {
super(message, messageType, optionType, icon, options);
initComponents();
}
public JideOptionPane(Object message, int messageType, int optionType, Icon icon, Object[] options, Object initialValue) {
super(message, messageType, optionType, icon, options, initialValue);
initComponents();
}
protected void initComponents() {
}
/**
* A new type for the option pane to have only the close button.
*/
public static final int CLOSE_OPTION = 3;
/**
* Overrides the method in JOptionPane to allow a new option - CLOSE_OPTION.
*
* @param newType the type of the option pane.
*/
@Override
public void setOptionType(int newType) {
if (newType != DEFAULT_OPTION && newType != YES_NO_OPTION &&
newType != YES_NO_CANCEL_OPTION && newType != OK_CANCEL_OPTION
&& newType != CLOSE_OPTION)
throw new RuntimeException("JOptionPane: option type must be one of JOptionPane.DEFAULT_OPTION, JOptionPane.YES_NO_OPTION, JOptionPane.YES_NO_CANCEL_OPTION or JOptionPane.OK_CANCEL_OPTION");
int oldType = optionType;
optionType = newType;
firePropertyChange(OPTION_TYPE_PROPERTY, oldType, optionType);
}
/**
* Sets the details object. The object can be a string or a component. If it is a string, it will be put into a
* JTextArea. If it is a component, it will be used directly. As long as the value is not null, a "Details" button
* will be added to button panel allowing you to show or hide the details panel.
*
* @param details the details.
*/
public void setDetails(Object details) {
Object oldDetails = _details;
_details = details;
firePropertyChange(DETAILS_PROPERTY, oldDetails, _details);
}
/**
* Gets the details object. The object can be a string or a component. If it is a string, it will be put into a
* JTextArea. If it is a component, it will be used directly. As long as the value is not null, a "Details" button
* will be added to button panel allowing you to show or hide the details panel.
*
* @return the details object.
*/
public Object getDetails() {
return _details;
}
/**
* Gets the title of the option pane.
*
* @return the title of the option pane.
*/
public Object getTitle() {
return _title;
}
/**
* Sets the title of the option pane.
*
* @param title the new title of the option pane.
*/
public void setTitle(Object title) {
Object old = _title;
_title = title;
firePropertyChange(TITLE_PROPERTY, old, _title);
}
/**
* Sets the details component visible. Please note that you need to call this method before the option pane is
* shown. The visible flag is actually stored on a static field so if you set one option pane visible, all option
* panes' details component will be visible.
*
* @param visible true or false.
*/
public void setDetailsVisible(boolean visible) {
((BasicJideOptionPaneUI) getUI()).setDetailsVisible(visible);
}
/**
* Gets the localized string from resource bundle. Subclass can override it to provide its own string. Available
* keys are defined in buttons.properties that begin with "Button.".
*
* @param key the resource string key
*
* @return the localized string.
*/
public String getResourceString(String key) {
return ButtonResources.getResourceBundle(getLocale()).getString(key);
}
/**
* Checks if the details component is visible.
*
* @return true if visible. Otherwise false.
*/
public boolean isDetailsVisible() {
return ((BasicJideOptionPaneUI) getUI()).isDetailsVisible();
}
@Override
public void setLocale(Locale l) {
if (!JideSwingUtilities.equals(l, getLocale())) {
super.setLocale(l);
updateUI();
}
}
// public boolean isBannerVisible() {
// return _bannerVisible;
// }
//
// public void setBannerVisible(boolean bannerVisible) {
// _bannerVisible = bannerVisible;
// }
/**
* Shows a question-message dialog requesting input from the user. The dialog uses the default frame, which usually
* means it is centered on the screen.
*
* @param message the Object
to display
*
* @throws java.awt.HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static String showInputDialog(Object message)
throws HeadlessException {
return showInputDialog(null, message);
}
/**
* Shows a question-message dialog requesting input from the user, with the input value initialized to
* initialSelectionValue
. The dialog uses the default frame, which usually means it is centered on the
* screen.
*
* @param message the Object
to display
* @param initialSelectionValue the value used to initialize the input field
*
* @since 1.4
*/
public static String showInputDialog(Object message, Object initialSelectionValue) {
return showInputDialog(null, message, initialSelectionValue);
}
/**
* Shows a question-message dialog requesting input from the user parented to parentComponent
. The
* dialog is displayed on top of the Component
's frame, and is usually positioned below the
* Component
.
*
* @param parentComponent the parent Component
for the dialog
* @param message the Object
to display
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static String showInputDialog(Component parentComponent,
Object message) throws HeadlessException {
return showInputDialog(parentComponent, message, UIManager.getString("OptionPane.inputDialogTitle"), QUESTION_MESSAGE);
}
/**
* Shows a question-message dialog requesting input from the user and parented to parentComponent
. The
* input value will be initialized to initialSelectionValue
. The dialog is displayed on top of the
* Component
's frame, and is usually positioned below the Component
.
*
* @param parentComponent the parent Component
for the dialog
* @param message the Object
to display
* @param initialSelectionValue the value used to initialize the input field
*
* @since 1.4
*/
public static String showInputDialog(Component parentComponent, Object message,
Object initialSelectionValue) {
return (String) showInputDialog(parentComponent, message,
UIManager.getString("OptionPane.inputDialogTitle"), QUESTION_MESSAGE, null, null,
initialSelectionValue);
}
/**
* Shows a dialog requesting input from the user parented to parentComponent
with the dialog having the
* title title
and message type messageType
.
*
* @param parentComponent the parent Component
for the dialog
* @param message the Object
to display
* @param title the String
to display in the dialog title bar
* @param messageType the type of message that is to be displayed: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static String showInputDialog(Component parentComponent,
Object message, String title, int messageType)
throws HeadlessException {
return (String) showInputDialog(parentComponent, message, title,
messageType, null, null, null);
}
/**
* Prompts the user for input in a blocking dialog where the initial selection, possible selections, and all other
* options can be specified. The user will able to choose from selectionValues
, where null
* implies the user can input whatever they wish, usually by means of a JTextField
.
* initialSelectionValue
is the initial value to prompt the user with. It is up to the UI to decide how
* best to represent the selectionValues
, but usually a JComboBox
, JList
, or
* JTextField
will be used.
*
* @param parentComponent the parent Component
for the dialog
* @param message the Object
to display
* @param title the String
to display in the dialog title bar
* @param messageType the type of message to be displayed: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
* @param icon the Icon
image to display
* @param selectionValues an array of Object
s that gives the possible selections
* @param initialSelectionValue the value used to initialize the input field
*
* @return user's input, or null
meaning the user canceled the input
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static Object showInputDialog(Component parentComponent,
Object message, String title, int messageType, Icon icon,
Object[] selectionValues, Object initialSelectionValue)
throws HeadlessException {
JideOptionPane pane = new JideOptionPane(message, messageType,
OK_CANCEL_OPTION, icon,
null, null);
if (parentComponent != null) {
pane.setLocale(parentComponent.getLocale());
}
pane.setWantsInput(true);
pane.setSelectionValues(selectionValues);
pane.setInitialSelectionValue(initialSelectionValue);
pane.setComponentOrientation(((parentComponent == null) ?
getRootFrame() : parentComponent).getComponentOrientation());
int style = styleFromMessageType(messageType);
JDialog dialog = pane.createDialog(parentComponent, title, style);
pane.selectInitialValue();
dialog.setVisible(true);
dialog.dispose();
Object value = pane.getInputValue();
if (value == UNINITIALIZED_VALUE) {
return null;
}
return value;
}
private static int styleFromMessageType(int messageType) {
switch (messageType) {
case ERROR_MESSAGE:
return JRootPane.ERROR_DIALOG;
case QUESTION_MESSAGE:
return JRootPane.QUESTION_DIALOG;
case WARNING_MESSAGE:
return JRootPane.WARNING_DIALOG;
case INFORMATION_MESSAGE:
return JRootPane.INFORMATION_DIALOG;
case PLAIN_MESSAGE:
default:
return JRootPane.PLAIN_DIALOG;
}
}
/**
* Brings up an information-message dialog titled "Message".
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static void showMessageDialog(Component parentComponent,
Object message) throws HeadlessException {
showMessageDialog(parentComponent, message, UIManager.getString("OptionPane.messageDialogTitle"),
INFORMATION_MESSAGE);
}
/**
* Brings up a dialog that displays a message using a default icon determined by the messageType
* parameter.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
* @param title the title string for the dialog
* @param messageType the type of message to be displayed: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static void showMessageDialog(Component parentComponent,
Object message, String title, int messageType)
throws HeadlessException {
showMessageDialog(parentComponent, message, title, messageType, null);
}
/**
* Brings up a dialog displaying a message, specifying all parameters.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
* @param title the title string for the dialog
* @param messageType the type of message to be displayed: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
* @param icon an icon to display in the dialog that helps the user identify the kind of message that is
* being displayed
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static void showMessageDialog(Component parentComponent,
Object message, String title, int messageType, Icon icon)
throws HeadlessException {
showOptionDialog(parentComponent, message, title, DEFAULT_OPTION,
messageType, icon, null, null);
}
/**
* Brings up a dialog with the options Yes, No and Cancel; with the title, Select an
* Option.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
*
* @return an integer indicating the option selected by the user
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static int showConfirmDialog(Component parentComponent,
Object message) throws HeadlessException {
return showConfirmDialog(parentComponent, message,
UIManager.getString("OptionPane.titleText"),
YES_NO_CANCEL_OPTION);
}
/**
* Brings up a dialog where the number of choices is determined by the optionType
parameter.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
* @param title the title string for the dialog
* @param optionType an int designating the options available on the dialog: YES_NO_OPTION
,
* YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
*
* @return an int indicating the option selected by the user
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static int showConfirmDialog(Component parentComponent,
Object message, String title, int optionType)
throws HeadlessException {
return showConfirmDialog(parentComponent, message, title, optionType,
QUESTION_MESSAGE);
}
/**
* Brings up a dialog where the number of choices is determined by the optionType
parameter, where the
* messageType
parameter determines the icon to display. The messageType
parameter is
* primarily used to supply a default icon from the Look and Feel.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used.
* @param message the Object
to display
* @param title the title string for the dialog
* @param optionType an integer designating the options available on the dialog: YES_NO_OPTION
,
* YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
* @param messageType an integer designating the kind of message this is; primarily used to determine the icon
* from the pluggable Look and Feel: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
*
* @return an integer indicating the option selected by the user
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static int showConfirmDialog(Component parentComponent,
Object message, String title, int optionType, int messageType)
throws HeadlessException {
return showConfirmDialog(parentComponent, message, title, optionType,
messageType, null);
}
/**
* Brings up a dialog with a specified icon, where the number of choices is determined by the
* optionType
parameter. The messageType
parameter is primarily used to supply a default
* icon from the look and feel.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object to display
* @param title the title string for the dialog
* @param optionType an int designating the options available on the dialog: YES_NO_OPTION
,
* YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
* @param messageType an int designating the kind of message this is, primarily used to determine the icon from
* the pluggable Look and Feel: ERROR_MESSAGE
, INFORMATION_MESSAGE
,
* WARNING_MESSAGE
, QUESTION_MESSAGE
, or
* PLAIN_MESSAGE
* @param icon the icon to display in the dialog
*
* @return an int indicating the option selected by the user
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static int showConfirmDialog(Component parentComponent,
Object message, String title, int optionType,
int messageType, Icon icon) throws HeadlessException {
return showOptionDialog(parentComponent, message, title, optionType,
messageType, icon, null, null);
}
/**
* Brings up a dialog with a specified icon, where the initial choice is determined by the initialValue
* parameter and the number of choices is determined by the optionType
parameter.
*
* If optionType
is YES_NO_OPTION
, or YES_NO_CANCEL_OPTION
and the
* options
parameter is null
, then the options are supplied by the look and feel.
*
* The messageType
parameter is primarily used to supply a default icon from the look and feel.
*
* @param parentComponent determines the Frame
in which the dialog is displayed; if null
,
* or if the parentComponent
has no Frame
, a default
* Frame
is used
* @param message the Object
to display
* @param title the title string for the dialog
* @param optionType an integer designating the options available on the dialog: DEFAULT_OPTION
,
* YES_NO_OPTION
, YES_NO_CANCEL_OPTION
, or
* OK_CANCEL_OPTION
* @param messageType an integer designating the kind of message this is, primarily used to determine the icon
* from the pluggable Look and Feel: ERROR_MESSAGE
,
* INFORMATION_MESSAGE
, WARNING_MESSAGE
,
* QUESTION_MESSAGE
, or PLAIN_MESSAGE
* @param icon the icon to display in the dialog
* @param options an array of objects indicating the possible choices the user can make; if the objects are
* components, they are rendered properly; non-String
objects are rendered using
* their toString
methods; if this parameter is null
, the options
* are determined by the Look and Feel
* @param initialValue the object that represents the default selection for the dialog; only meaningful if
* options
is used; can be null
*
* @return an integer indicating the option chosen by the user, or CLOSED_OPTION
if the user closed the
* dialog
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static int showOptionDialog(Component parentComponent,
Object message, String title, int optionType, int messageType,
Icon icon, Object[] options, Object initialValue)
throws HeadlessException {
JideOptionPane pane = new JideOptionPane(message, messageType,
optionType, icon,
options, initialValue);
if (parentComponent != null) {
pane.setLocale(parentComponent.getLocale());
}
pane.setInitialValue(initialValue);
pane.setComponentOrientation(((parentComponent == null) ?
getRootFrame() : parentComponent).getComponentOrientation());
int style = styleFromMessageType(messageType);
JDialog dialog = pane.createDialog(parentComponent, title, style);
pane.selectInitialValue();
dialog.setVisible(true);
dialog.dispose();
Object selectedValue = pane.getValue();
if (selectedValue == null)
return CLOSED_OPTION;
if (options == null) {
if (selectedValue instanceof Integer)
return ((Integer) selectedValue).intValue();
return CLOSED_OPTION;
}
for (int counter = 0, maxCounter = options.length;
counter < maxCounter; counter++) {
if (options[counter].equals(selectedValue))
return counter;
}
return CLOSED_OPTION;
}
/**
* Creates and returns a new JDialog
wrapping this
centered on the
* parentComponent
in the parentComponent
's frame. title
is the title of the
* returned dialog. The returned JDialog
will not be resizable by the user, however programs can invoke
* setResizable
on the JDialog
instance to change this property. The returned
* JDialog
will be set up such that once it is closed, or the user clicks on one of the buttons, the
* optionpane's value property will be set accordingly and the dialog will be closed. Each time the dialog is made
* visible, it will reset the option pane's value property to JOptionPane.UNINITIALIZED_VALUE
to ensure
* the user's subsequent action closes the dialog properly.
*
* @param parentComponent determines the frame in which the dialog is displayed; if the parentComponent
* has no Frame
, a default Frame
is used
* @param title the title string for the dialog
*
* @return a new JDialog
containing this instance
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public JDialog createDialog(Component parentComponent, String title)
throws HeadlessException {
int style = styleFromMessageType(getMessageType());
return createDialog(parentComponent, title, style);
}
/**
* Creates and returns a new parentless JDialog
with the specified title. The returned
* JDialog
will not be resizable by the user, however programs can invoke setResizable
on
* the JDialog
instance to change this property. The returned JDialog
will be set up such
* that once it is closed, or the user clicks on one of the buttons, the optionpane's value property will be set
* accordingly and the dialog will be closed. Each time the dialog is made visible, it will reset the option pane's
* value property to JOptionPane.UNINITIALIZED_VALUE
to ensure the user's subsequent action closes the
* dialog properly.
*
* @param title the title string for the dialog
*
* @return a new JDialog
containing this instance
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
* @since 1.6
*/
public JDialog createDialog(String title) throws HeadlessException {
int style = styleFromMessageType(getMessageType());
JDialog dialog = new JDialog((Dialog) null, title, true);
initDialog(dialog, style, null);
return dialog;
}
private JDialog createDialog(Component parentComponent, String title,
int style)
throws HeadlessException {
final JDialog dialog;
Window window = JideOptionPane.getWindowForComponent(parentComponent);
if (window instanceof Frame) {
dialog = new JDialog((Frame) window, title, true);
}
else {
dialog = new JDialog((Dialog) window, title, true);
}
// if (window instanceof SwingUtilities.SharedOwnerFrame) {
// WindowListener ownerShutdownListener =
// (WindowListener) SwingUtilities.getSharedOwnerFrameShutdownListener();
// dialog.addWindowListener(ownerShutdownListener);
// }
initDialog(dialog, style, parentComponent);
return dialog;
}
private void initDialog(final JDialog dialog, int style, Component parentComponent) {
dialog.setComponentOrientation(this.getComponentOrientation());
Container contentPane = dialog.getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(this, BorderLayout.CENTER);
dialog.setResizable(false);
if (JDialog.isDefaultLookAndFeelDecorated()) {
boolean supportsWindowDecorations =
UIManager.getLookAndFeel().getSupportsWindowDecorations();
if (supportsWindowDecorations) {
dialog.setUndecorated(true);
getRootPane().setWindowDecorationStyle(style);
}
}
dialog.pack();
dialog.setLocationRelativeTo(parentComponent);
WindowAdapter adapter = new WindowAdapter() {
private boolean gotFocus = false;
public void windowClosing(WindowEvent we) {
setValue(null);
}
public void windowGainedFocus(WindowEvent we) {
// Once window gets focus, set initial focus
if (!gotFocus) {
selectInitialValue();
gotFocus = true;
}
}
};
dialog.addWindowListener(adapter);
dialog.addWindowFocusListener(adapter);
dialog.addComponentListener(new ComponentAdapter() {
public void componentShown(ComponentEvent ce) {
// reset value to ensure closing works properly
setValue(JOptionPane.UNINITIALIZED_VALUE);
}
});
addPropertyChangeListener(new PropertyChangeListener() {
public void propertyChange(PropertyChangeEvent event) {
// Let the defaultCloseOperation handle the closing
// if the user closed the window without selecting a button
// (newValue = null in that case). Otherwise, close the dialog.
if (dialog.isVisible() && event.getSource() == JideOptionPane.this &&
(event.getPropertyName().equals(VALUE_PROPERTY)) &&
event.getNewValue() != null &&
event.getNewValue() != JOptionPane.UNINITIALIZED_VALUE) {
dialog.setVisible(false);
}
}
});
}
/**
* Returns the specified component's Frame
.
*
* @param parentComponent the Component
to check for a Frame
*
* @return the Frame
that contains the component, or getRootFrame
if the component is
* null
, or does not have a valid Frame
parent
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see #getRootFrame
* @see java.awt.GraphicsEnvironment#isHeadless
*/
public static Frame getFrameForComponent(Component parentComponent)
throws HeadlessException {
if (parentComponent == null)
return getRootFrame();
if (parentComponent instanceof Frame)
return (Frame) parentComponent;
return JOptionPane.getFrameForComponent(parentComponent.getParent());
}
/**
* Returns the specified component's toplevel Frame
or Dialog
.
*
* @param parentComponent the Component
to check for a Frame
or Dialog
*
* @return the Frame
or Dialog
that contains the component, or the default frame if the
* component is null
, or does not have a valid Frame
or Dialog
* parent
*
* @throws HeadlessException if GraphicsEnvironment.isHeadless
returns true
* @see java.awt.GraphicsEnvironment#isHeadless
*/
static Window getWindowForComponent(Component parentComponent)
throws HeadlessException {
if (parentComponent == null)
return getRootFrame();
if (parentComponent instanceof Frame || parentComponent instanceof Dialog)
return (Window) parentComponent;
return JideOptionPane.getWindowForComponent(parentComponent.getParent());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy