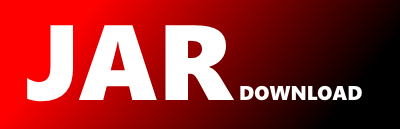
com.jidesoft.utils.MathUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jide-oss Show documentation
Show all versions of jide-oss Show documentation
JIDE Common Layer (Professional Swing Components)
package com.jidesoft.utils;
import com.jidesoft.range.IntegerRange;
import com.jidesoft.range.LongRange;
import com.jidesoft.range.NumericRange;
import com.jidesoft.range.Range;
import java.util.ArrayList;
import java.util.List;
import static java.lang.Double.*;
/**
* A collection of several util methods related to Math. We only used it in DefaultSummaryCalculator in JIDE Pivot Grid
* to calculate statistics but this class will be reserved as a place holder for methods related to Math.
*/
public final class MathUtils {
protected MathUtils() {
}
/**
* Returns the sum number in the numbers list.
*
* @param numbers the numbers to calculate the sum.
* @return the sum of the numbers.
*/
public static double sum(List numbers) {
double sum = 0;
for (Number value : numbers) {
sum += value.doubleValue();
}
return sum;
}
/**
* Returns the mean number in the numbers list.
*
* @param numbers the numbers to calculate the mean.
* @return the mean of the numbers.
*/
public static double mean(List numbers) {
double sum = sum(numbers);
return sum / numbers.size();
}
/**
* Returns the min number in the numbers list.
*
* @param numbers the numbers to calculate the min.
* @return the min number in the numbers list.
*/
public static double min(List numbers) {
double min = MAX_VALUE;
for (Number value : numbers) {
double v = value.doubleValue();
if (v < min) {
min = v;
}
}
return min;
}
/**
* Returns the max number in the numbers list.
*
* @param numbers the numbers to calculate the max.
* @return the max number in the numbers list.
*/
public static double max(List numbers) {
double max = MIN_VALUE;
for (Number value : numbers) {
double v = value.doubleValue();
if (v > max) {
max = v;
}
}
return max;
}
/**
* Returns the standard deviation of the numbers.
*
* Double.NaN is returned if the numbers list is empty.
*
* @param numbers the numbers to calculate the standard deviation.
* @param biasCorrected true if variance is calculated by dividing by n - 1. False if by n. stddev is a sqrt of the
* variance.
* @return the standard deviation
*/
public static double stddev(List numbers, boolean biasCorrected) {
double stddev = NaN;
int n = numbers.size();
if (n > 0) {
if (n > 1) {
stddev = Math.sqrt(var(numbers, biasCorrected));
}
else {
stddev = 0.0;
}
}
return stddev;
}
/**
* Computes the variance of the available values. By default, the unbiased "sample variance" definitional formula is
* used: variance = sum((x_i - mean)^2) / (n - 1)
*
* The "population variance" ( sum((x_i - mean)^2) / n ) can also be computed using this statistic. The
* biasCorrected
property determines whether the "population" or "sample" value is returned by the
* evaluate
and getResult
methods. To compute population variances, set this property to
* false
.
*
* @param numbers the numbers to calculate the variance.
* @param biasCorrected true if variance is calculated by dividing by n - 1. False if by n.
* @return the variance of the numbers.
*/
public static double var(List numbers, boolean biasCorrected) {
int n = numbers.size();
if (n == 0) {
return NaN;
}
else if (n == 1) {
return 0d;
}
double mean = mean(numbers);
List squares = new ArrayList();
for (Number number : numbers) {
double XminMean = number.doubleValue() - mean;
squares.add(Math.pow(XminMean, 2));
}
double sum = sum(squares);
return sum / (biasCorrected ? (n - 1) : n);
}
/**
* Returns the range of numbers.
*
* @param numbers the numbers to calculate the range.
* @return the range of the numbers.
*/
public static Range range(List numbers) {
double min = MAX_VALUE;
double max = MIN_VALUE;
for (Number value : numbers) {
double v = value.doubleValue();
if (v < min) {
min = v;
}
if (v > max) {
max = v;
}
}
return new NumericRange(min, max);
}
/**
* Returns the range of numbers.
*
* @param numbers the numbers to calculate the range.
* @return the range of the numbers.
*/
public static Range rangeInteger(List numbers) {
double min = Integer.MAX_VALUE;
double max = Integer.MIN_VALUE;
for (Number value : numbers) {
double v = value.doubleValue();
if (v < min) {
min = v;
}
if (v > max) {
max = v;
}
}
return new IntegerRange((int) min, (int) max);
}
/**
* Returns the range of numbers.
*
* @param numbers the numbers to calculate the range.
* @return the range of the numbers.
*/
public static Range rangeLong(List numbers) {
double min = Long.MAX_VALUE;
double max = Long.MIN_VALUE;
for (Number value : numbers) {
double v = value.doubleValue();
if (v < min) {
min = v;
}
if (v > max) {
max = v;
}
}
return new LongRange((long) min, (long) max);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy