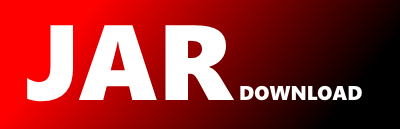
com.jnape.palatable.lambda.functions.specialized.Predicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.functions.specialized;
import com.jnape.palatable.lambda.functions.Fn1;
import java.util.function.Function;
/**
* A specialized {@link Fn1} that returns a Boolean
.
*
* @param The argument type
*/
public interface Predicate extends Fn1, java.util.function.Predicate {
/**
* {@inheritDoc}
*/
@Override
default boolean test(A a) {
return apply(a);
}
/**
* Override of {@link Function#compose(Function)}, returning an instance of Predicate
for
* compatibility. Right-to-left composition.
*
* @param before the function who's return value is this predicate's argument
* @param the new argument type
* @return a new predicate of Z (the new argument type)
*/
@Override
@SuppressWarnings("unchecked")
default Predicate compose(Function super Z, ? extends A> before) {
return Fn1.super.compose(before)::apply;
}
/**
* {@inheritDoc}
*/
@Override
@SuppressWarnings("unchecked")
default Predicate diMapL(Fn1 fn) {
return Fn1.super.diMapL(fn)::apply;
}
/**
* Override of {@link java.util.function.Predicate#and(java.util.function.Predicate)}, returning an instance of
* Predicate
for compatibility. Left-to-right composition.
*
* @param other the predicate to test if this one succeeds
* @return a predicate representing the conjunction of this predicate and other
*/
@Override
default Predicate and(java.util.function.Predicate super A> other) {
return a -> apply(a) && other.test(a);
}
/**
* Override of {@link java.util.function.Predicate#or(java.util.function.Predicate)}, returning an instance of
* Predicate
for compatibility. Left-to-right composition.
*
* @param other the predicate to test if this one fails
* @return a predicate representing the disjunction of this predicate and other
*/
@Override
default Predicate or(java.util.function.Predicate super A> other) {
return a -> apply(a) || other.test(a);
}
/**
* Override of {@link java.util.function.Predicate#negate()}, returning an instance of Predicate
for
* compatibility.
*
* @return the negation of this predicate
*/
@Override
default Predicate negate() {
return a -> !apply(a);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy