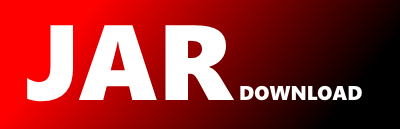
com.jnape.palatable.lambda.functions.Fn2 Maven / Gradle / Ivy
package com.jnape.palatable.lambda.functions;
import com.jnape.palatable.lambda.adt.hlist.Tuple2;
import java.util.function.BiFunction;
/**
* A function taking two arguments. Note that defining Fn2
in terms of Fn1
provides a
* reasonable approximation of currying in the form of multiple apply
overloads that take different numbers
* of arguments.
*
* @param The first argument type
* @param The second argument type
* @param The return type
* @see Fn1
* @see com.jnape.palatable.lambda.functions.builtin.fn2.Partial2
*/
@FunctionalInterface
public interface Fn2 extends Fn1> {
/**
* Invoke this function with the given arguments.
*
* @param a the first argument
* @param b the second argument
* @return the result of the function application
*/
C apply(A a, B b);
/**
* Partially apply this function by passing its first argument.
*
* @param a the first argument
* @return an Fn1 that takes the second argument and returns the result
*/
@Override
default Fn1 apply(A a) {
return (b) -> apply(a, b);
}
/**
* Flip the order of the arguments.
*
* @return an Fn2 that takes the first and second arguments in reversed order
*/
default Fn2 flip() {
return (b, a) -> apply(a, b);
}
/**
* Returns an Fn1
that takes the arguments as a Tuple2<A, B>
.
*
* @return an Fn1 taking a Tuple2
*/
default Fn1, C> uncurry() {
return (ab) -> apply(ab._1(), ab._2());
}
/**
* View this Fn2
as a j.u.f.BiFunction
.
*
* @return the same logic as a BiFunction
* @see BiFunction
*/
default BiFunction toBiFunction() {
return this::apply;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy