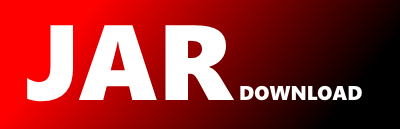
com.jnape.palatable.lambda.lens.lenses.MapLens Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.lens.lenses;
import com.jnape.palatable.lambda.lens.Lens;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import static com.jnape.palatable.lambda.lens.Lens.lens;
import static com.jnape.palatable.lambda.lens.Lens.simpleLens;
import static com.jnape.palatable.lambda.lens.functions.View.view;
import static com.jnape.palatable.lambda.lens.lenses.OptionalLens.unLiftA;
import static java.util.stream.Collectors.toSet;
/**
* Lenses that operate on {@link Map}s.
*/
public final class MapLens {
private MapLens() {
}
/**
* Convenience static factory method for creating a lens that focuses on a copy of a Map. Useful for composition to
* avoid mutating a map reference.
*
* @param the key type
* @param the value type
* @return a lens that focuses on copies of maps
*/
public static Lens.Simple
© 2015 - 2025 Weber Informatics LLC | Privacy Policy