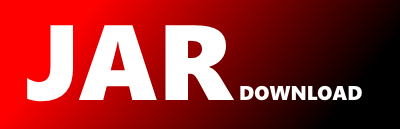
com.jnape.palatable.lambda.adt.hmap.HMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.adt.hmap;
import com.jnape.palatable.lambda.adt.hlist.Tuple2;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import static com.jnape.palatable.lambda.functions.builtin.fn2.Map.map;
import static java.util.Collections.emptyMap;
import static java.util.Collections.singletonMap;
/**
* An immutable heterogeneous mapping from a parametrized type-safe key to any value, supporting a minimal mapping
* interface.
*
* @see TypeSafeKey
* @see com.jnape.palatable.lambda.adt.hlist.HList
*/
public class HMap implements Iterable> {
private static final HMap EMPTY = new HMap(emptyMap());
private final Map table;
private HMap(Map table) {
this.table = table;
}
/**
* Retrieve the value at this key.
*
* @param key the key
* @param the value type
* @return the value at this key wrapped in an {@link Optional}, or {@link Optional#empty}.
*/
@SuppressWarnings("unchecked")
public Optional get(TypeSafeKey key) {
return Optional.ofNullable((T) table.get(key));
}
/**
* Retrieve the value at this key, throwing a {@link NoSuchElementException} if this key is unmapped.
*
* @param key the key
* @param the value type
* @return the value at this key
* @throws NoSuchElementException if the key is unmapped
*/
public V demand(TypeSafeKey key) throws NoSuchElementException {
return get(key).orElseThrow(() -> new NoSuchElementException("Demanded value for key " + key + ", but couldn't find one."));
}
/**
* Store a value for the given key.
*
* @param key the key
* @param value the value
* @param the value type
* @return the updated HMap
*/
public HMap put(TypeSafeKey key, V value) {
return alter(t -> t.put(key, value));
}
/**
* Store all the key/value mappings in hMap
in this HMap.
*
* @param hMap the other HMap
* @return the updated HMap
*/
public HMap putAll(HMap hMap) {
return alter(t -> t.putAll(hMap.table));
}
/**
* Determine if a key is mapped.
*
* @param key the key
* @return true if the key is mapped; false otherwise
*/
public boolean containsKey(TypeSafeKey key) {
return table.containsKey(key);
}
/**
* Remove a mapping from this HMap.
*
* @param key the key
* @return the updated HMap
*/
public HMap remove(TypeSafeKey key) {
return alter(t -> t.remove(key));
}
/**
* Remove all the key/value mappings in hMap
from this HMap.
*
* @param hMap the other HMap
* @return the updated HMap
*/
public HMap removeAll(HMap hMap) {
return alter(t -> t.keySet().removeAll(hMap.table.keySet()));
}
/**
* Retrieve all the mapped keys.
*
* @return an Iterable of all the mapped keys
*/
public Iterable keys() {
return map(Tuple2::_1, this);
}
/**
* Retrieve all the mapped values.
*
* @return an Iterable of all the mapped values
*/
public Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy