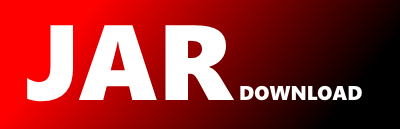
com.jnape.palatable.lambda.functions.builtin.fn2.ReduceRight Maven / Gradle / Ivy
Show all versions of lambda Show documentation
package com.jnape.palatable.lambda.functions.builtin.fn2;
import com.jnape.palatable.lambda.functions.Fn1;
import com.jnape.palatable.lambda.functions.Fn2;
import java.util.Optional;
import java.util.function.BiFunction;
import static com.jnape.palatable.lambda.functions.builtin.fn1.Reverse.reverse;
import static com.jnape.palatable.lambda.functions.builtin.fn2.ReduceLeft.reduceLeft;
/**
* Given an Iterable
of A
s and a {@link BiFunction}<A, A, A>
, iteratively
* accumulate over the Iterable
, returning an Optional<A>
(if the Iterable
* is empty, the result is Optional.empty()
; otherwise, the result is wrapped in
* Optional.of()
. For this reason, null
accumulation results are considered erroneous and will
* throw.
*
* This function is isomorphic to a right fold over the Iterable
where the tail element is the starting
* accumulation value and the result is lifted into an Optional
.
*
* @param The input Iterable element type, as well as the accumulation type
* @see ReduceLeft
* @see com.jnape.palatable.lambda.functions.builtin.fn3.FoldRight
*/
public final class ReduceRight implements Fn2, Iterable, Optional> {
private ReduceRight() {
}
@Override
public final Optional apply(BiFunction super A, ? super A, ? extends A> fn, Iterable as) {
return reduceLeft((b, a) -> fn.apply(a, b), reverse(as));
}
public static ReduceRight reduceRight() {
return new ReduceRight<>();
}
public static Fn1, Optional> reduceRight(BiFunction super A, ? super A, ? extends A> fn) {
return ReduceRight.reduceRight().apply(fn);
}
public static Optional reduceRight(BiFunction super A, ? super A, ? extends A> fn, Iterable as) {
return ReduceRight.reduceRight(fn).apply(as);
}
}