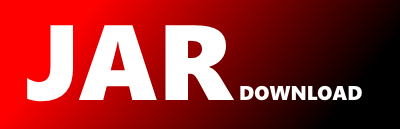
com.jnape.palatable.lambda.functions.Effect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.functions;
import com.jnape.palatable.lambda.adt.Unit;
import com.jnape.palatable.lambda.functions.specialized.SideEffect;
import com.jnape.palatable.lambda.functor.Applicative;
import com.jnape.palatable.lambda.internal.Runtime;
import com.jnape.palatable.lambda.io.IO;
import java.util.function.Consumer;
import static com.jnape.palatable.lambda.adt.Unit.UNIT;
import static com.jnape.palatable.lambda.functions.builtin.fn1.Constantly.constantly;
import static com.jnape.palatable.lambda.functions.specialized.SideEffect.NOOP;
import static com.jnape.palatable.lambda.io.IO.io;
/**
* A function returning "no result", and therefore only useful as a side-effect.
*
* @param the argument type
* @see Fn0
*/
@FunctionalInterface
public interface Effect extends Fn1> {
@Override
IO checkedApply(A a) throws Throwable;
/**
* Convert this {@link Effect} to a java {@link Consumer}
*
* @return the {@link Consumer}
*/
default Consumer toConsumer() {
return a -> apply(a).unsafePerformIO();
}
/**
* {@inheritDoc}
*/
@Override
default IO apply(A a) {
try {
return checkedApply(a);
} catch (Throwable t) {
throw Runtime.throwChecked(t);
}
}
/**
* Left-to-right composition of {@link Effect Effects}.
*
* @param effect the other {@link Effect}
* @return the composed {@link Effect}
*/
default Effect andThen(Effect effect) {
return a -> apply(a).flatMap(constantly(effect.apply(a)));
}
/**
* {@inheritDoc}
*/
@Override
default Effect diMapL(Fn1 super Z, ? extends A> fn) {
return effect(Fn1.super.diMapL(fn));
}
/**
* {@inheritDoc}
*/
@Override
default Effect contraMap(Fn1 super Z, ? extends A> fn) {
return effect(Fn1.super.contraMap(fn));
}
/**
* {@inheritDoc}
*/
@Override
default Effect discardR(Applicative> appB) {
return effect(Fn1.super.discardR(appB));
}
/**
* Static factory method to create an {@link Effect} from a java {@link Consumer}.
*
* @param consumer the {@link Consumer}
* @param the input type
* @return the {@link Effect}
*/
static Effect fromConsumer(Consumer consumer) {
return a -> io(() -> consumer.accept(a));
}
/**
* Create an {@link Effect} from a {@link SideEffect};
*
* @param sideEffect the {@link SideEffect}
* @param any desired input type
* @return the {@link Effect}
*/
static Effect effect(SideEffect sideEffect) {
return effect(constantly(io(sideEffect)));
}
/**
* Create an {@link Effect} that accepts an input and does nothing;
*
* @param any desired input type
* @return the noop {@link Effect}
*/
@SuppressWarnings("unused")
static Effect noop() {
return effect(NOOP);
}
/**
* Create an {@link Effect} from an {@link Fn1} that yields an {@link IO}.
*
* @param fn the function
* @param the effect argument type
* @return the effect
*/
static Effect effect(Fn1 super A, ? extends IO>> fn) {
return fn.fmap(io -> io.fmap(constantly(UNIT)))::apply;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy