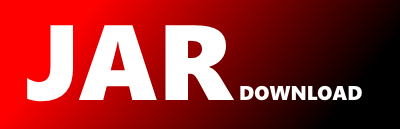
com.jnape.palatable.lambda.functor.builtin.Exchange Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.functor.builtin;
import com.jnape.palatable.lambda.functions.Fn1;
import com.jnape.palatable.lambda.functor.Profunctor;
import com.jnape.palatable.lambda.optics.Iso;
/**
* A profunctor used to extract the isomorphic functions an {@link Iso} is composed of.
*
* @param the smaller viewed value of an {@link Iso}
* @param the smaller viewing value of an {@link Iso}
* @param the larger viewing value of an {@link Iso}
* @param the larger viewed value of an {@link Iso}
*/
public final class Exchange implements Profunctor> {
private final Fn1 super S, ? extends A> sa;
private final Fn1 super B, ? extends T> bt;
public Exchange(Fn1 super S, ? extends A> sa, Fn1 super B, ? extends T> bt) {
this.sa = sa;
this.bt = bt;
}
/**
* Extract the mapping S -> A
.
*
* @return an {@link Fn1}<S, A>
*/
public Fn1 super S, ? extends A> sa() {
return sa;
}
/**
* Extract the mapping B -> T
.
*
* @return an {@link Fn1}<B, T>
*/
public Fn1 super B, ? extends T> bt() {
return bt;
}
/**
* {@inheritDoc}
*/
@Override
public Exchange diMap(Fn1 super Z, ? extends S> lFn,
Fn1 super T, ? extends C> rFn) {
return new Exchange<>(lFn.fmap(sa), bt.fmap(rFn));
}
/**
* {@inheritDoc}
*/
@Override
public Exchange diMapL(Fn1 super Z, ? extends S> fn) {
return (Exchange) Profunctor.super.diMapL(fn);
}
/**
* {@inheritDoc}
*/
@Override
public Exchange diMapR(Fn1 super T, ? extends C> fn) {
return (Exchange) Profunctor.super.diMapR(fn);
}
/**
* {@inheritDoc}
*/
@Override
public Exchange contraMap(Fn1 super Z, ? extends S> fn) {
return (Exchange) Profunctor.super.contraMap(fn);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy