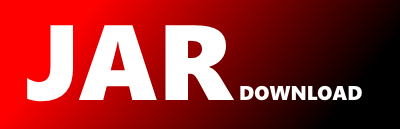
com.jnape.palatable.lambda.monoid.builtin.First Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lambda Show documentation
Show all versions of lambda Show documentation
Functional patterns for Java
package com.jnape.palatable.lambda.monoid.builtin;
import com.jnape.palatable.lambda.adt.Maybe;
import com.jnape.palatable.lambda.functions.Fn1;
import com.jnape.palatable.lambda.monoid.Monoid;
import static com.jnape.palatable.lambda.adt.Maybe.nothing;
import static com.jnape.palatable.lambda.functions.builtin.fn1.CatMaybes.catMaybes;
import static com.jnape.palatable.lambda.functions.builtin.fn1.Head.head;
import static com.jnape.palatable.lambda.functions.builtin.fn2.Map.map;
/**
* A {@link Monoid} instance formed by {@link Maybe}<A>
. The application to two {@link Maybe} values
* produces the first non-empty value, or {@link Maybe#nothing()} if all values are empty.
*
* @param the Maybe value parameter type
* @see Last
* @see Present
* @see Monoid
* @see Maybe
*/
public final class First implements Monoid> {
private static final First> INSTANCE = new First<>();
private First() {
}
@Override
public Maybe identity() {
return nothing();
}
@Override
public Maybe checkedApply(Maybe x, Maybe y) {
return x.fmap(Maybe::just).orElse(y);
}
@Override
public Maybe foldMap(Fn1 super B, ? extends Maybe> fn, Iterable bs) {
return head(catMaybes(map(fn, bs)));
}
@SuppressWarnings("unchecked")
public static First first() {
return (First) INSTANCE;
}
public static Fn1, Maybe> first(Maybe x) {
return First.first().apply(x);
}
public static Maybe first(Maybe x, Maybe y) {
return first(x).apply(y);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy