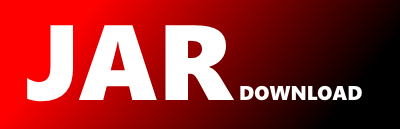
com.jnape.palatable.lambda.functions.builtin.fn3.Times Maven / Gradle / Ivy
Show all versions of lambda Show documentation
package com.jnape.palatable.lambda.functions.builtin.fn3;
import com.jnape.palatable.lambda.functions.Fn1;
import com.jnape.palatable.lambda.functions.Fn2;
import com.jnape.palatable.lambda.functions.Fn3;
import static com.jnape.palatable.lambda.functions.builtin.fn2.Replicate.replicate;
import static com.jnape.palatable.lambda.functions.builtin.fn3.FoldLeft.foldLeft;
/**
* Given some number of times n
to invoke a function A -> A
, and given an input
* A
, iteratively apply the function to the input, and then to the result of the invocation, a total of
* n
times, returning the result.
*
* Example:
*
* times(3, x -> x + 1, 0); // 3
*
* @param the input and output type
*/
public final class Times implements Fn3, A, A> {
private static final Times> INSTANCE = new Times<>();
private Times() {
}
@Override
public A checkedApply(Integer n, Fn1 super A, ? extends A> fn, A a) {
if (n < 0)
throw new IllegalStateException("n must not be less than 0");
return foldLeft((acc, f) -> f.apply(acc), a, replicate(n, fn));
}
@SuppressWarnings("unchecked")
public static Times times() {
return (Times) INSTANCE;
}
public static Fn2, A, A> times(Integer n) {
return Times.times().apply(n);
}
public static Fn1 times(Integer n, Fn1 super A, ? extends A> fn) {
return Times.times(n).apply(fn);
}
public static A times(Integer n, Fn1 super A, ? extends A> fn, A a) {
return Times.times(n, fn).apply(a);
}
}