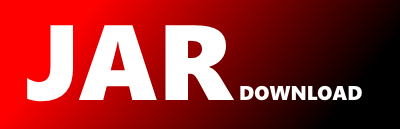
com.joliciel.talismane.machineLearning.features.EqualsOperator Maven / Gradle / Ivy
///////////////////////////////////////////////////////////////////////////////
//Copyright (C) 2014 Joliciel Informatique
//
//This file is part of Talismane.
//
//Talismane is free software: you can redistribute it and/or modify
//it under the terms of the GNU Affero General Public License as published by
//the Free Software Foundation, either version 3 of the License, or
//(at your option) any later version.
//
//Talismane is distributed in the hope that it will be useful,
//but WITHOUT ANY WARRANTY; without even the implied warranty of
//MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
//GNU Affero General Public License for more details.
//
//You should have received a copy of the GNU Affero General Public License
//along with Talismane. If not, see .
//////////////////////////////////////////////////////////////////////////////
package com.joliciel.talismane.machineLearning.features;
import com.joliciel.talismane.TalismaneException;
/**
* Returns operand1 == operand2. For double values, an error margin of 0.0001 is
* allowed.
*
* Note: because of enormous usage of this features, it has been split into four
* separate features, to gain an infinitesimal amount of performance.
*
* @author Assaf Urieli
*
*/
public class EqualsOperator extends AbstractCachableFeatureimplements BooleanFeature {
private Feature operand1;
private Feature operand2;
private double sigma = 0.0001;
private Class> operandType;
public EqualsOperator(DoubleFeature operand1, DoubleFeature operand2) {
super();
this.operand1 = operand1;
this.operand2 = operand2;
this.operandType = Double.class;
this.setName(operand1.getName() + "==" + operand2.getName());
}
public EqualsOperator(IntegerFeature operand1, IntegerFeature operand2) {
super();
this.operand1 = operand1;
this.operand2 = operand2;
this.operandType = Integer.class;
this.setName(operand1.getName() + "==" + operand2.getName());
}
public EqualsOperator(StringFeature operand1, StringFeature operand2) {
super();
this.operand1 = operand1;
this.operand2 = operand2;
this.operandType = String.class;
this.setName(operand1.getName() + "==" + operand2.getName());
}
public EqualsOperator(BooleanFeature operand1, BooleanFeature operand2) {
super();
this.operand1 = operand1;
this.operand2 = operand2;
this.operandType = Boolean.class;
this.setName(operand1.getName() + "==" + operand2.getName());
}
@SuppressWarnings("unchecked")
@Override
protected FeatureResult checkInternal(T context, RuntimeEnvironment env) throws TalismaneException {
FeatureResult featureResult = null;
if (operandType.equals(Double.class)) {
FeatureResult operand1Result = (FeatureResult) operand1.check(context, env);
FeatureResult operand2Result = (FeatureResult) operand2.check(context, env);
if (operand1Result != null && operand2Result != null) {
double diff = Math.abs(operand1Result.getOutcome() - operand2Result.getOutcome());
boolean result = diff <= sigma;
featureResult = this.generateResult(result);
}
} else if (operandType.equals(Integer.class)) {
FeatureResult operand1Result = (FeatureResult) operand1.check(context, env);
FeatureResult operand2Result = (FeatureResult) operand2.check(context, env);
if (operand1Result != null && operand2Result != null) {
boolean result = operand1Result.getOutcome() == operand2Result.getOutcome();
featureResult = this.generateResult(result);
}
} else if (operandType.equals(String.class)) {
FeatureResult operand1Result = (FeatureResult) operand1.check(context, env);
FeatureResult operand2Result = (FeatureResult) operand2.check(context, env);
if (operand1Result != null && operand2Result != null) {
boolean result = operand1Result.getOutcome().equals(operand2Result.getOutcome());
featureResult = this.generateResult(result);
}
} else if (operandType.equals(Boolean.class)) {
FeatureResult operand1Result = (FeatureResult) operand1.check(context, env);
FeatureResult operand2Result = (FeatureResult) operand2.check(context, env);
if (operand1Result != null && operand2Result != null) {
boolean result = operand1Result.getOutcome().equals(operand2Result.getOutcome());
featureResult = this.generateResult(result);
}
}
return featureResult;
}
public Feature getOperand1() {
return operand1;
}
public Feature getOperand2() {
return operand2;
}
public void setOperand1(Feature operand1) {
this.operand1 = operand1;
}
public void setOperand2(Feature operand2) {
this.operand2 = operand2;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy