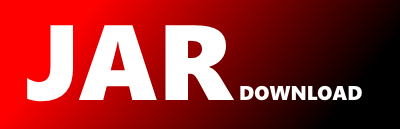
com.google.code.joliratools.logdb.parser.LogParserFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parser Show documentation
Show all versions of parser Show documentation
An efficient parser for Java-style log records.
/**
* Copyright (c) 2010 jolira. All rights reserved. This program and the accompanying materials are made available under
* the terms of the GNU Public License 2.0 which is available at http://www.gnu.org/licenses/old-licenses/gpl-2.0.html
*/
package com.google.code.joliratools.logdb.parser;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.regex.Pattern;
/**
* This is an immutable class for efficiently parsing log files given a set of regular expressions. This class is
* thread-safe.
*
* @author ikrimer
* @since 1.0
*/
public class LogParserFactory implements ParserFactory {
// if different instances of this class can have different patterns and formats remove static
private static Pattern headerPattern;
private static Pattern lineDelimiter;
private static Collection patternsWithFlags = new LinkedHashSet();
// TODO move following into a config file
private static final int capacity = 1024 << 2; // should be multiple of the page size
private static final int bufferSize = 1024 << 2; // should be multiple of the page size
private static final SimpleDateFormat formatter = new SimpleDateFormat("MMM dd, yyyy hh:mm:ss aa");
private static final String[] empty = {};
// private static final int avgLineLength = 80;
/**
* Create a new parser from a set of regular expression describing the patterns to be evaluated in a log file.
*
* @param regex
* the map of regular expression to be used for parsing to regex flags CASE_INSENSITIVE, MULTILINE,
* DOTALL, UNICODE_CASE, and CANON_EQ The map is linked hash map to emphasize relevance of order and
* @param lineSeparatorRegex
* regular expression describing what to use as a line separator
*/
public LogParserFactory(final Map regex, final String lineSeparatorRegex) {
final Iterator patternIterator = regex.keySet().iterator();
String headerPatternString = patternIterator.next();
final int headerPatternFlags = regex.get(headerPatternString).intValue();
// lazy initialize patterns
if (null == headerPattern || null == patternsWithFlags || null == lineDelimiter) {
lineDelimiter = Pattern.compile(lineSeparatorRegex);
headerPattern = Pattern.compile(headerPatternString, headerPatternFlags);
while (patternIterator.hasNext()) {
headerPatternString = patternIterator.next();
patternsWithFlags.add(Pattern.compile(headerPatternString, headerPatternFlags));
}
}
}
@Override
public Parser parse(final InputStream in) {
return null; // TODO: LogParser(in);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy