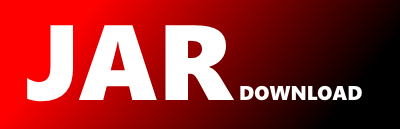
com.google.code.joliratools.logdb.reader.LogDigester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parser Show documentation
Show all versions of parser Show documentation
An efficient parser for Java-style log records.
/**
* Copyright (c) 2010 jolira. All rights reserved. This program and the accompanying materials are made available under
* the terms of the GNU Public License 2.0 which is available at http://www.gnu.org/licenses/old-licenses/gpl-2.0.html
*/
package com.google.code.joliratools.logdb.reader;
import java.io.IOException;
import java.util.Map;
import com.google.code.joliratools.logdb.parser.ParseException;
import com.google.code.joliratools.logdb.parser.Parser;
import com.google.code.joliratools.logdb.parser.Record;
import com.google.code.joliratools.logdb.parser.Record.Type;
/**
* @author jfk
* @date Sep 16, 2010 7:41:31 AM
* @since 1.0
*/
public abstract class LogDigester implements Reader {
/**
* @see Reader#getNext(Parser)
*/
@Override
public Entry getNext(final Parser parser) throws IOException, ParseException {
final Record record = parser.getNext();
if (record == null) {
return null;
}
return new Entry() {
private Map cachedVars = null;
@Override
public String getClazz() {
return record.getClazz();
}
@Override
public String getId() {
return record.getId();
}
@Override
public String getLevel() {
return record.getLevel();
}
@Override
public int getLineNumber() {
return record.getLineNumber();
}
@Override
public String getMethod() {
return record.getMethod();
}
@Override
public String getRaw() {
return record.getRaw();
}
@Override
public long getTimestamp() {
return record.getTimestamp();
}
@Override
public Type getType() {
return record.getType();
}
@Override
public synchronized Map getVariables() throws IOException, ParseException {
if (cachedVars != null) {
return cachedVars;
}
return cachedVars = LogDigester.this.getVariables(record);
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
builder.append("LogDigester#Entry [record=");
builder.append(record);
if (cachedVars != null) {
builder.append(", ");
builder.append("cachedVars=");
builder.append(cachedVars);
}
return builder.toString();
}
};
}
/**
* @param record
* the record
* @return the process variables, or {@literal null} if the record is junk or unrecognized.
* @throws ParseException
* parsing failed
* @throws IOException
* reading failed
*/
protected final Map getVariables(final Record record) throws IOException, ParseException {
final Type type = record.getType();
if (!Type.RECOGNIZED.equals(type)) {
return null;
}
return processVariables(record);
}
/**
* @param record
* the record to be processed
* @return the variables by name.
* @throws ParseException
* parsing failed
* @throws IOException
* reading failed
*/
protected abstract Map processVariables(Record record) throws IOException, ParseException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy