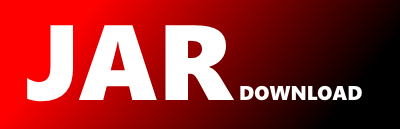
com.jolira.st4j.impl.MetricScope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of server-tracker-4j Show documentation
Show all versions of server-tracker-4j Show documentation
A client library for the Server Tracker. (http://github.com/jolira/server-tracker)
package com.jolira.st4j.impl;
import java.lang.annotation.Annotation;
import javax.inject.Named;
import com.google.inject.Key;
import com.google.inject.Provider;
import com.google.inject.Scope;
import com.google.inject.TypeLiteral;
import com.jolira.st4j.Metric;
import com.jolira.st4j.MetricStore;
/**
* Create metrics for Guice.
*
* @author jfk
* @date Aug 9, 2011 7:58:15 AM
* @since 1.0
*
*/
public class MetricScope implements Scope {
private static Annotation getAnnotation(final Key key) {
final Annotation annotation = key.getAnnotation();
if (annotation != null) {
return annotation;
}
final TypeLiteral literal = key.getTypeLiteral();
final Class super T> raw = literal.getRawType();
return raw.getAnnotation(Metric.class);
}
private static String getName(final Annotation annotation) {
if (annotation == null) {
return null;
}
if (annotation instanceof Metric) {
final Metric metric = (Metric) annotation;
return metric.value();
}
if (annotation instanceof Named) {
final Named named = (Named) annotation;
return named.value();
}
throw new IllegalArgumentException("only @" + Metric.class.getName() + " and @" + Named.class.getName()
+ " can be used to specify metrics.");
}
private static boolean isUnique(final Annotation annotation) {
if (annotation == null || !(annotation instanceof Metric)) {
return true; // default
}
final Metric metric = (Metric) annotation;
return metric.unique();
}
private final MetricStore store;
/**
* Create a new scope.
*
* @param store
* the store to use
*/
public MetricScope(final MetricStore store) {
this.store = store;
}
T getScoped(final Key key, final Provider unscoped) {
final TypeLiteral literal = key.getTypeLiteral();
final Class super T> type = literal.getRawType();
final Annotation annotation = getAnnotation(key);
final String mname = getName(annotation);
final boolean unique = isUnique(annotation);
if (unique) {
@SuppressWarnings("unchecked")
final T metric = (T) store.getThreadLocalMeasurement(mname, type);
if (metric != null) {
return metric;
}
}
final T _metric = unscoped.get();
store.postThreadLocalMeasurement(mname, _metric, unique);
return _metric;
}
@Override
public Provider scope(final Key key, final Provider unscoped) {
return new Provider() {
@Override
public T get() {
return getScoped(key, unscoped);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy