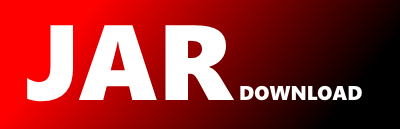
com.jongsoft.Startable Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft;
public interface Startable {
/**
* The status enum provides information into the current state of a {@link Startable} instance.
*/
public static enum Status {
IDLE, // The instance is not running at the moment
STARTING, // The instance is starting and not yet ready
RUNNING, // The instance is ready for further instructions
RESTARTING, // The instance is performing a restart and not yet ready
KILLED, // An underlying resource has been killed, the instance needs to be restarted
FAILED, // The startup has failed
STOPPING // The instance is performing a shutdown operation
}
/**
* Start the entity, this should allocate any needed resources and will allow the instance to accept further instructions
* when completed.
*
* @return true
if the start command succeeded
*/
boolean start();
/**
* Stop the instance. This call will release any allocated resources and result into a {@link #getStatus()} call returning
* {@link Status#IDLE}.
*/
void stop();
/**
* Get the current state of the instance.
*
* @return the status of the instance
* @see Status
*/
Status getStatus();
/**
* Attempt to perform a restart if the status is FAILED, RUNNING or RESTARTING.
*
* @return true if the restart succeeded, false the instance is in incorrect state or restart failed.
*/
default boolean restart() {
if (getStatus() == Status.FAILED || getStatus() == Status.RUNNING || getStatus() == Status.RESTARTING) {
stop();
return start();
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy