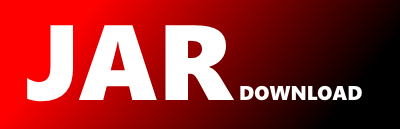
com.jongsoft.highchart.axis.AxisLabels Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.axis;
import com.jongsoft.Builder;
import com.jongsoft.highchart.common.Alignment;
import com.jongsoft.highchart.common.CSSObject;
import com.jongsoft.highchart.common.Function;
import java.util.ArrayList;
import java.util.List;
public class AxisLabels implements Builder {
private final T parent;
private Alignment align;
private final List autoRotation;
private Number autoRotationLimit;
private Number distance;
private Boolean enabled;
private String format;
private Function.Formatter formatter;
private Number padding;
private Boolean reserveSpace;
private Number rotation;
private Number staggerLines;
private Number step;
private final CSSObject> style;
private Boolean useHTML;
private Number x;
private Number y;
private Number zIndex;
public AxisLabels(T parent) {
this.parent = parent;
autoRotation = new ArrayList<>();
this.style = new CSSObject<>(this);
}
/**
* What part of the string the given position is anchored to. Can be one of "left", "center" or "right". Defaults to
* an intelligent guess based on which side of the chart the axis is on and the rotation of the label.
*
* Default: {@link Alignment#CENTER}.
*/
public AxisLabels setAlign(Alignment align) {
this.align = align;
return this;
}
/**
* For horizontal axes, the allowed degrees of label rotation to prevent overlapping labels. If there is enough
* space, labels are not rotated. As the chart gets narrower, it will start rotating the labels -45 degrees,
* then remove every second label and try again with rotations 0 and -45 etc. Set it to false to disable rotation,
* which will cause the labels to word-wrap if possible.
*
* Default: [-45].
*/
public AxisLabels addAutoRotation(Number rotation) {
autoRotation.add(rotation);
return this;
}
/**
* When each category width is more than this many pixels, we don't apply auto rotation. Instead, we lay out the
* axis label with word wrap. A lower limit makes sense when the label contains multiple short words that don't
* extend the available horizontal space for each label.
*
* Default: 80.
*/
public AxisLabels setAutoRotationLimit(Number autoRotationLimit) {
this.autoRotationLimit = autoRotationLimit;
return this;
}
/**
* Polar charts only. The label's pixel distance from the perimeter of the plot area.
*
* Default: 15.
*/
public AxisLabels setDistance(Number distance) {
this.distance = distance;
return this;
}
/**
* Enable or disable the axis labels.
*
* Default: true.
*/
public AxisLabels setEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* A format string
* for the axis label.
*
* Default: {value}.
*/
public AxisLabels setFormat(String format) {
this.format = format;
return this;
}
/**
* Callback JavaScript function to format the label. The value is given by this.value. Additional properties for this are axis, chart, isFirst and isLast
*/
public AxisLabels setFormatter(Function.Formatter formatter) {
this.formatter = formatter;
return this;
}
/**
* The pixel padding for axis labels, to ensure white space between them.
*
* Default: 5.
*/
public AxisLabels setPadding(Number padding) {
this.padding = padding;
return this;
}
/**
* Whether to reserve space for the labels. This can be turned off when for example the labels are rendered inside the plot area instead of outside.
*
* Default: true
*/
public AxisLabels setReserveSpace(Boolean reserveSpace) {
this.reserveSpace = reserveSpace;
return this;
}
/**
* Rotation of the labels in degrees.
*
* Default: 0
*/
public AxisLabels setRotation(Number rotation) {
this.rotation = rotation;
return this;
}
/**
* Horizontal axes only. The number of lines to spread the labels over to make room or tighter labels.
*/
public AxisLabels setStaggerLines(Number staggerLines) {
this.staggerLines = staggerLines;
return this;
}
/**
* To show only every n'th label on the axis, set the step to n. Setting the step to 2 shows every other label.
*
* By default, the step is calculated automatically to avoid overlap. To prevent this, set it to 1. This usually
* only happens on a category axis, and is often a sign that you have chosen the wrong axis type.
* @see Read more at Axis docs: What axis should I use?
*/
public AxisLabels setStep(Number step) {
this.step = step;
return this;
}
/**
* Whether to use HTML to render the labels.
*
* Default: false
*/
public AxisLabels setUseHTML(Boolean useHTML) {
this.useHTML = useHTML;
return this;
}
/**
* The x position offset of the label relative to the tick position on the axis
*
* Default: 0
*/
public AxisLabels setX(Number x) {
this.x = x;
return this;
}
/**
* The y position offset of the label relative to the tick position on the axis. The default makes it
* adapt to the font size on bottom axis.
*
* Default: null
*/
public AxisLabels setY(Number y) {
this.y = y;
return this;
}
/**
* The Z index for the axis labels.
*
* Default: 7
*/
public AxisLabels setzIndex(Number zIndex) {
this.zIndex = zIndex;
return this;
}
@Override
public T build() {
return parent;
}
public Alignment getAlign() {
return align;
}
public List getAutoRotation() {
return autoRotation;
}
public Number getAutoRotationLimit() {
return autoRotationLimit;
}
public Number getDistance() {
return distance;
}
public Boolean getEnabled() {
return enabled;
}
public String getFormat() {
return format;
}
public Function.Formatter getFormatter() {
return formatter;
}
public Boolean getReserveSpace() {
return reserveSpace;
}
public Number getPadding() {
return padding;
}
public Number getRotation() {
return rotation;
}
public Number getStaggerLines() {
return staggerLines;
}
/**
* CSS styles for the label. Use whiteSpace: 'nowrap' to prevent wrapping of category labels.
* Use textOverflow: 'none' to prevent ellipsis (dots).
*/
public CSSObject> getStyle() {
return style;
}
public Boolean getUseHTML() {
return useHTML;
}
public Number getStep() {
return step;
}
public Number getX() {
return x;
}
public Number getY() {
return y;
}
public Number getzIndex() {
return zIndex;
}
}