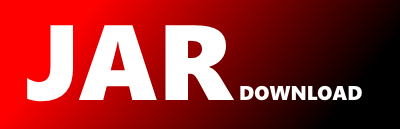
com.jongsoft.highchart.axis.PlotLine Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.axis;
import com.jongsoft.highchart.common.*;
public class PlotLine {
public class PlotLineLabel {
private Alignment align;
private Number rotation;
private CSSObject style;
private String text;
private Alignment textAlign;
private Boolean useHTML;
private VerticalAlignment verticalAlign;
private Number x;
private Number y;
/**
* Horizontal alignment of the label. Can be one of "left", "center" or "right".
*
* Default: {@link Alignment#LEFT}
*/
public PlotLineLabel setAlign(Alignment align) {
this.align = align;
return this;
}
/**
* Rotation of the text label in degrees. Defaults to 0 for horizontal plot lines and 90 for vertical lines.
*/
public PlotLineLabel setRotation(Number rotation) {
this.rotation = rotation;
return this;
}
/**
* The text itself. A subset of HTML is supported.
*/
public PlotLineLabel setText(String text) {
this.text = text;
return this;
}
/**
* The text alignment for the label. While align determines where the texts anchor point is placed within the plot band, textAlign determines how the
* text is aligned against its anchor point. Possible values are "left", "center" and "right".
*
* Default: to the same as {@link #getAlign()}
*/
public PlotLineLabel setTextAlign(Alignment textAlign) {
this.textAlign = textAlign;
return this;
}
/**
* Whether to use HTML to render the labels.
*
* Default: false
*/
public PlotLineLabel setUseHTML(Boolean useHTML) {
this.useHTML = useHTML;
return this;
}
/**
* Vertical alignment of the label relative to the plot band.
*
* Default: {@link VerticalAlignment#TOP}
*/
public PlotLineLabel setVerticalAlign(VerticalAlignment verticalAlign) {
this.verticalAlign = verticalAlign;
return this;
}
/**
* Horizontal position relative the alignment. Default varies by orientation.
*/
public PlotLineLabel setX(Number x) {
this.x = x;
return this;
}
/**
* Vertical position of the text baseline relative to the alignment. Default varies by orientation.
*/
public PlotLineLabel setY(Number y) {
this.y = y;
return this;
}
public CSSObject getStyle() {
if (style == null) {
style = new CSSObject<>(this);
}
return style;
}
public Alignment getAlign() {
return align;
}
public Number getRotation() {
return rotation;
}
public String getText() {
return text;
}
public Alignment getTextAlign() {
return textAlign;
}
public Boolean getUseHTML() {
return useHTML;
}
public VerticalAlignment getVerticalAlign() {
return verticalAlign;
}
public Number getX() {
return x;
}
public Number getY() {
return y;
}
}
private GraphColor color;
private DashStyle dashStyle;
private String id;
private PlotLineLabel label;
private Number value;
private Number width;
private Number zIndex;
/**
* The color of the line.
*/
public PlotLine setColor(GraphColor color) {
this.color = color;
return this;
}
/**
* The dashing or dot style for the plot line
*/
public PlotLine setDashStyle(DashStyle dashStyle) {
this.dashStyle = dashStyle;
return this;
}
/**
* An id used for identifying the plot line in Axis.removePlotLine.
*/
public PlotLine setId(String id) {
this.id = id;
return this;
}
/**
* The position of the line in axis units
*/
public PlotLine setValue(Number value) {
this.value = value;
return this;
}
/**
* The width or thickness of the plot line.
*/
public PlotLine setWidth(Number width) {
this.width = width;
return this;
}
/**
* The z index of the plot line within the chart.
*/
public PlotLine setZIndex(Number zIndex) {
this.zIndex = zIndex;
return this;
}
/**
* Text labels for the plot bands
*/
public PlotLineLabel getLabel() {
if (label == null) {
label = new PlotLineLabel();
}
return label;
}
public GraphColor getColor() {
return color;
}
public DashStyle getDashStyle() {
return dashStyle;
}
public String getId() {
return id;
}
public Number getValue() {
return value;
}
public Number getWidth() {
return width;
}
public Number getzIndex() {
return zIndex;
}
}