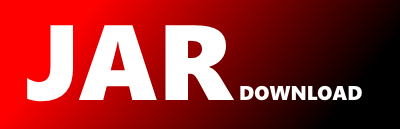
com.jongsoft.highchart.chart.ChartEvents Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.chart;
import com.jongsoft.highchart.common.Function;
public class ChartEvents {
private final T parent;
private Function addSeries;
private Function afterPrint;
private Function beforePrint;
private Function click;
private Function drilldown;
private Function drillup;
private Function drillupall;
private Function load;
private Function redraw;
private Function selection;
public ChartEvents(T parent) {
this.parent = parent;
}
/**
* Fires when a series is added to the chart after load time, using the addSeries method. One parameter, event, is
* passed to the function. This contains common event information based on jQuery or MooTools depending on which
* library is used as the base for Highcharts. Through event.options you can access the series options that was
* passed to the addSeries method.
*
* Returning false prevents the series from being added.
*
* @param addSeries
*/
public void setAddSeries(Function addSeries) {
this.addSeries = addSeries;
}
/**
* Fires after a chart is printed through the context menu item or the Chart.print method. Requires the exporting
* module.
*
* @param afterPrint
*/
public void setAfterPrint(Function afterPrint) {
this.afterPrint = afterPrint;
}
/**
* Fires before a chart is printed through the context menu item or the Chart.print method. Requires the exporting
* module.
*
* @param beforePrint
*/
public void setBeforePrint(Function beforePrint) {
this.beforePrint = beforePrint;
}
/**
* Fires when clicking on the plot background. One parameter, event, is passed to the function. This contains common
* event information based on jQuery or MooTools depending on which library is used as the base for Highcharts.
*
* Information on the clicked spot can be found through event.xAxis and event.yAxis, which are arrays containing the
* axes of each dimension and each axis' value at the clicked spot. The primary axes are event.xAxis[0] and
* event.yAxis[0]. Remember the unit of a datetime axis is milliseconds since 1970-01-01 00:00:00.
*
*
* click: function(e) {
* console.log(
* Highcharts.dateFormat('%Y-%m-%d %H:%M:%S', e.xAxis[0].value),
* e.yAxis[0].value
* )
* }
*
*
* The this keyword refers to the Chart object.
*
* @param click
*/
public void setClick(Function click) {
this.click = click;
}
public void setDrilldown(Function drilldown) {
this.drilldown = drilldown;
}
public void setDrillup(Function drillup) {
this.drillup = drillup;
}
public void setDrillupall(Function drillupall) {
this.drillupall = drillupall;
}
/**
* Fires when the chart is finished loading. Since v4.2.2, it also waits for images to be loaded, for example from
* point markers. One parameter, event, is passed to the function. This contains common event information based on
* jQuery or MooTools depending on which library is used as the base for Highcharts.
*
* There is also a second parameter to the chart constructor where a callback function can be passed to be executed
* on chart.load.
*
* The this keyword refers to the Chart object.
*
* @param load
*/
public void setLoad(Function load) {
this.load = load;
}
public void setRedraw(Function redraw) {
this.redraw = redraw;
}
public void setSelection(Function selection) {
this.selection = selection;
}
public T build() {
return parent;
}
public Function getAddSeries() {
return addSeries;
}
public Function getAfterPrint() {
return afterPrint;
}
public Function getBeforePrint() {
return beforePrint;
}
public Function getClick() {
return click;
}
public Function getDrilldown() {
return drilldown;
}
public Function getDrillup() {
return drillup;
}
public Function getDrillupall() {
return drillupall;
}
public Function getLoad() {
return load;
}
public Function getRedraw() {
return redraw;
}
public Function getSelection() {
return selection;
}
}