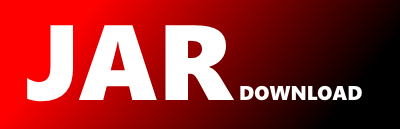
com.jongsoft.highchart.chart.Tooltip Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.chart;
import com.jongsoft.Builder;
import com.jongsoft.highchart.common.CSSObject;
import com.jongsoft.highchart.common.Crosshair;
import com.jongsoft.highchart.common.Function;
import com.jongsoft.highchart.common.Function.Formatter;
import com.jongsoft.highchart.common.Function.Positioner;
import com.jongsoft.highchart.common.Styled;
import com.jongsoft.highchart.series.SeriesPoint;
public class Tooltip extends Styled> implements Builder {
private final T parent;
private Boolean animation;
private Crosshair> crosshair;
private Boolean enabled;
private Boolean followPointer;
private Boolean followTouchMove;
private String footerFormat;
private Formatter formatter;
private String headerFormat;
private Number hideDelay;
private String pointFormat;
private Function pointFormatter;
private Positioner positioner;
private Boolean shadow;
private String shape;
private Boolean shared;
private Number snap;
private CSSObject> style;
private Boolean useHTML;
private Number valueDecimals;
public Tooltip(T parent) {
this.parent = parent;
this.crosshair = new Crosshair<>(self());
this.style = new CSSObject<>(self());
}
public Tooltip setAnimation(Boolean animation) {
this.animation = animation;
return self();
}
/**
* Enable or disable the tooltip.
*
* Default: true.
*/
public Tooltip setEnabled(Boolean enabled) {
this.enabled = enabled;
return self();
}
/**
* Whether the tooltip should follow the mouse as it moves across columns, pie slices and other point types with an extent. By default it behaves this way
* for scatter, bubble and pie series by override in the plotOptions for those series types.
*
* Note: For touch moves to behave the same way, {@link #setFollowTouchMove(java.lang.Boolean) } must be true also.
* Default: false
*/
public Tooltip setFollowPointer(Boolean followPointer) {
this.followPointer = followPointer;
return self();
}
/**
* Whether the tooltip should follow the finger as it moves on a touch device. If chart.zoomType is set, it will override followTouchMove.
*
* Default true
*/
public Tooltip setFollowTouchMove(Boolean followTouchMove) {
this.followTouchMove = followTouchMove;
return self();
}
/**
* A string to append to the tooltip format
*/
public Tooltip setFooterFormat(String footerFormat) {
this.footerFormat = footerFormat;
return self();
}
/**
* Callback function to format the text of the tooltip. Return false to disable tooltip for a specific point on series.
*
* @see Formatter
*/
public Tooltip setFormatter(Formatter formatter) {
this.formatter = formatter;
return self();
}
/**
* The HTML of the tooltip header line. Variables are enclosed by curly brackets. Available variables are point.key, series.name, series.color and other
* members from the point and series objects. The point.key variable contains the category name, x value or datetime string depending on the type of axis.
* For datetime axes, the point.key date format can be set using tooltip.xDateFormat.
*
* Default: <span style="font-size: 10px">{point.key}</span>
*/
public Tooltip setHeaderFormat(String headerFormat) {
this.headerFormat = headerFormat;
return self();
}
/**
* The number of milliseconds to wait until the tooltip is hidden when mouse out from a point or chart.
*
* Default 500
*/
public Tooltip setHideDelay(Number hideDelay) {
this.hideDelay = hideDelay;
return self();
}
/**
* The HTML of the point's line in the tooltip. Variables are enclosed by curly brackets. Available variables are point.x, point.y, series.name and
* series.color and other properties on the same form. Furthermore, point.y can be extended by the tooltip.valuePrefix and tooltip.valueSuffix variables.
* This can also be overridden for each series, which makes it a good hook for displaying units.
*
* Default: <span style="color:{point.color}">\u25CF</span> {series.name}: <b>{point.y}</b><br/>
*/
public Tooltip setPointFormat(String pointFormat) {
this.pointFormat = pointFormat;
return self();
}
/**
* A callback function for formatting the HTML output for a single point in the tooltip. Like the pointFormat string, but with more flexibility.
*
* The this keyword refers to the {@link SeriesPoint} object.
*/
public Tooltip setPointFormatter(Function pointFormatter) {
this.pointFormatter = pointFormatter;
return self();
}
/**
* A callback function to place the tooltip in a default position. The callback receives three parameters: labelWidth, labelHeight and point, where point
* contains values for plotX and plotY telling where the reference point is in the plot area. Add chart.plotLeft and chart.plotTop to get the full
* coordinates.
*
* The return should be an object containing x and y values, for example { x: 100, y: 100 }.
*/
public Tooltip setPositioner(Positioner positioner) {
this.positioner = positioner;
return self();
}
/**
* Whether to apply a drop shadow to the tooltip.
*
* Default: true
*/
public Tooltip setShadow(Boolean shadow) {
this.shadow = shadow;
return self();
}
/**
* The name of a symbol to use for the border around the tooltip.
*
* Default: callout
*/
public Tooltip setShape(String shape) {
this.shape = shape;
return self();
}
/**
* When the tooltip is shared, the entire plot area will capture mouse movement or touch events. Tooltip texts for series types with ordered data (not pie,
* scatter, flags etc) will be shown in a single bubble. This is recommended for single series charts and for tablet/mobile optimized charts.
*
* Default: false.
*/
public Tooltip setShared(Boolean shared) {
this.shared = shared;
return self();
}
/**
* Proximity snap for graphs or single points. Does not apply to bars, columns and pie slices. It defaults to 10 for mouse-powered devices and 25 for touch
* devices.
*/
public Tooltip setSnap(Number snap) {
this.snap = snap;
return self();
}
/**
* Use HTML to render the contents of the tooltip instead of SVG. Using HTML allows advanced formatting like tables and images in the tooltip. It is also
* recommended for rtl languages as it works around rtl bugs in early Firefox.
*
* Default: false.
*/
public Tooltip setUseHTML(Boolean useHTML) {
this.useHTML = useHTML;
return self();
}
/**
* How many decimals to show in each series' y value. This is overridable in each series' tooltip options object. The default is to preserve all decimals.
*/
public Tooltip setValueDecimals(Number valueDecimals) {
this.valueDecimals = valueDecimals;
return self();
}
@Override
public T build() {
return parent;
}
@Override
protected Tooltip self() {
return this;
}
public Boolean getAnimation() {
return animation;
}
public Crosshair> getCrosshair() {
return crosshair;
}
public CSSObject> getStyle() {
return style;
}
public Boolean getEnabled() {
return enabled;
}
public Boolean getFollowPointer() {
return followPointer;
}
public Boolean getFollowTouchMove() {
return followTouchMove;
}
public String getFooterFormat() {
return footerFormat;
}
public Formatter getFormatter() {
return formatter;
}
public String getHeaderFormat() {
return headerFormat;
}
public Number getHideDelay() {
return hideDelay;
}
public String getPointFormat() {
return pointFormat;
}
public Function getPointFormatter() {
return pointFormatter;
}
public Positioner getPositioner() {
return positioner;
}
public Boolean getShadow() {
return shadow;
}
public String getShape() {
return shape;
}
public Boolean getShared() {
return shared;
}
public Number getSnap() {
return snap;
}
public Boolean getUseHTML() {
return useHTML;
}
public Number getValueDecimals() {
return valueDecimals;
}
}