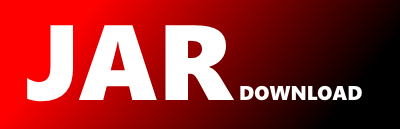
com.jongsoft.highchart.phantomjs.DriverPool Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.phantomjs;
import com.jongsoft.Startable;
import com.jongsoft.highchart.monitoring.Monitoring;
import org.apache.commons.pool2.impl.GenericObjectPool;
import org.openqa.selenium.phantomjs.PhantomJSDriver;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The DriverPool enables a method to get object pooling for the {@link PhantomJSDriver}. This allows for pre-allocating
* multiple drivers. Upon start-up the pool will be set with the following defaults:
*
* - 15 instances of {@link PhantomJSDriver} maximum
* - instances get validated before returned from the {@link #borrowObject(long)} call
* - the pool will block execution of any call attempting to obtain an instance when the pool is empty
*
*
* The driver pool utilizes the {@link DriverFactory} to get managed {@link PhantomJSDriver} instances.
* @see GenericObjectPool
*/
public class DriverPool extends GenericObjectPool implements Startable {
private static final Logger LOG = LoggerFactory.getLogger(DriverPool.class);
private Status status;
public DriverPool() {
super(new DriverFactory());
getFactory().start();
setMaxTotal(15);
setTestOnBorrow(true);
setBlockWhenExhausted(true);
}
@Override
public DriverFactory getFactory() {
return (DriverFactory) super.getFactory();
}
/**
* @see GenericObjectPool#borrowObject(long)
*/
@Override
@Monitoring
public TimedPhantomJsDriver borrowObject(long borrowMaxWaitMillis) throws Exception {
return super.borrowObject(borrowMaxWaitMillis);
}
@Override
public Status getStatus() {
return status;
}
@Override
@Monitoring
public boolean start() {
if (getFactory().start()) {
status = Status.RUNNING;
} else {
status = Status.FAILED;
}
LOG.trace("Starting the driver pool with resulting status " + status);
return status == Status.RUNNING;
}
@Override
@Monitoring
public void stop() {
if (status == Status.RUNNING || status == Status.RESTARTING) {
LOG.trace("Stopping the driver pool");
status = Status.STOPPING;
clear();
getFactory().stop();
status = Status.IDLE;
}
}
}