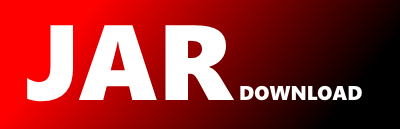
com.jongsoft.highchart.render.ExportApiRenderer Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.render;
import com.jongsoft.Renderer;
import com.jongsoft.highchart.AbstractHighchart;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
/**
* The {@link ExportApiRenderer} will enable you to render Highchart images using the official exporting server. To use a
* own hosted version please provide the system property highchart.export.api
with the full path of your own server.
*
* If the custom system property is not found it will fall back to the official Highchart export server.
*
* @see Export server documentation
*/
public class ExportApiRenderer implements Renderer> {
private static final String FALLBACK_API = "http://export.highcharts.com/";
private static final Logger LOG = LoggerFactory.getLogger(ImageRenderer.class);
private AbstractHighchart, ?> globalOptions;
private AbstractHighchart, ?> chartOptions;
private OutputStream outStream;
@Override
public void render() {
if (outStream == null) {
throw new RenderException("Cannot render highcharts when no output stream is set");
}
LOG.trace("Building HTTP client for request.");
HttpClient client = HttpClientBuilder.create()
.setDefaultRequestConfig(RequestConfig.custom()
.setConnectTimeout(5000)
.build())
.disableAuthCaching()
.build();
try {
LOG.trace("Building the parameter list for a HTTP Post operation.");
List params = new ArrayList<>();
params.add(new BasicNameValuePair("type", "image/png"));
params.add(new BasicNameValuePair("content", "options"));
params.add(new BasicNameValuePair("constr", "Chart"));
params.add(new BasicNameValuePair("scale", "1"));
params.add(new BasicNameValuePair("async", "false"));
params.add(new BasicNameValuePair("options", chartOptions.toJson()));
HttpPost postMessage = new HttpPost(FALLBACK_API);
postMessage.setEntity(new UrlEncodedFormEntity(params));
LOG.trace("Executing the call to the export server: " + postMessage.getURI() + "; data: "
+ String.join(System.lineSeparator(), IOUtils.readLines(postMessage.getEntity().getContent())));
HttpResponse renderResponse = client.execute(postMessage);
if (renderResponse.getStatusLine().getStatusCode() != HttpStatus.SC_OK) {
LOG.error("Unable render on export server due to " + renderResponse.getStatusLine().getReasonPhrase());
throw new RenderException("Could not render, export server returned error: " + renderResponse.getStatusLine().getReasonPhrase());
}
LOG.trace("Cloning the response stream to the output stream.");
IOUtils.copy(renderResponse.getEntity().getContent(), outStream);
postMessage.completed();
} catch (IOException ioException) {
LOG.error("Unable to save image to provided output stream", ioException);
throw new RenderException("Unable to save image to output stream");
}
}
@Override
public Renderer> setRenderObject(AbstractHighchart, ?> chartOptions) {
this.chartOptions = chartOptions;
return this;
}
@Override
public Renderer> setGlobalOptions(AbstractHighchart, ?> chartOptions) {
this.globalOptions = chartOptions;
return this;
}
@Override
public Renderer> setOutputStream(OutputStream outputStream) {
this.outStream = outputStream;
return this;
}
}