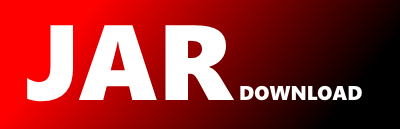
com.jongsoft.highchart.series.DataLabels Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.series;
import com.jongsoft.Builder;
import com.jongsoft.highchart.common.Alignment;
import com.jongsoft.highchart.common.Function;
import com.jongsoft.highchart.common.Styled;
public class DataLabels extends Styled> implements Builder {
private Alignment align;
private Boolean allowOverlap;
private String color;
private Boolean crop;
private Boolean defer;
private Boolean enabled;
private String format;
private Function.Formatter formatter;
private Boolean inside;
private String overflow;
private Number padding;
private final T parent;
private Boolean reserveSpace;
private Number rotation;
public DataLabels(T parent) {
this.parent = parent;
}
/**
* The alignment of the data label compared to the point. If right, the right side of the label should be
* touching the point. For points with an extent, like columns, the alignments also dictates how to align
* it inside the box, as given with the inside option. Can be one of "left", "center" or "right".
*
* Default: center.
*/
public DataLabels setAlign(Alignment align) {
this.align = align;
return self();
}
/**
* Whether to allow data labels to overlap. To make the labels less sensitive for overlapping,
* the {@link #setPadding(Number)} can be set to 0.
*
* Default: false.
*/
public DataLabels setAllowOverlap(Boolean allowOverlap) {
this.allowOverlap = allowOverlap;
return self();
}
/**
* The text color for the data labels.
*
* Default: null.
*/
public DataLabels setColor(String color) {
this.color = color;
return self();
}
/**
* Whether to hide data labels that are outside the plot area. By default, the data label is moved inside the
* plot area according to the {@link #setOverflow(String)} option.
*
* Default: true.
*
* @since 2.3.3
*/
public DataLabels setCrop(Boolean crop) {
this.crop = crop;
return self();
}
/**
* Whether to defer displaying the data labels until the initial series animation has finished.
*
* Default: true.
*
* @since 4.0
*/
public DataLabels setDefer(Boolean defer) {
this.defer = defer;
return self();
}
/**
* Enable or disable the data labels.
*
* Default: false.
*/
public DataLabels setEnabled(Boolean enabled) {
this.enabled = enabled;
return self();
}
/**
* For points with an extent, like columns, whether to align the data label inside the box or to the actual value point.
*
* Default: false in most cases, true in stacked columns.
*
* @since 3.0
*/
public DataLabels setInside(Boolean inside) {
this.inside = inside;
return self();
}
/**
* A format string for the data label. Available variables are the same as for formatter. Default: to {y}.
*
* @see Online documentation format rules
*/
public DataLabels setFormat(String format) {
this.format = format;
return self();
}
/**
* Callback JavaScript function to format the data label. Note that if a format is defined, the format takes precedence
* and the formatter is ignored.
*/
public DataLabels setFormatter(Function.Formatter formatter) {
this.formatter = formatter;
return self();
}
/**
* How to handle data labels that flow outside the plot area. The default is justify, which aligns them inside the plot area.
* For columns and bars, this means it will be moved inside the bar. To display data labels outside the plot area,
* set {@link #setCrop(Boolean)} to false and overflow to "none".
*
* Default: justify.
*
* @since 3.0.6
*/
public DataLabels setOverflow(String overflow) {
this.overflow = overflow;
return self();
}
/**
* When either the borderWidth or the backgroundColor is set, this is the padding within the box.
*
* Default: 5.
*
* @since 2.2.1
*/
public DataLabels setPadding(Number padding) {
this.padding = padding;
return self();
}
/**
* Whether to reserve space for the labels. This can be turned off when for example the labels are rendered inside
* the plot area instead of outside.
*
* Default: true
*
* @since 4.1.10
*/
public DataLabels setReserveSpace(Boolean reserveSpace) {
this.reserveSpace = reserveSpace;
return self();
}
/**
* Text rotation in degrees. Note that due to a more complex structure, backgrounds, borders and padding will
* be lost on a rotated data label.
*
* Default: 0
*/
public DataLabels setRotation(Number rotation) {
this.rotation = rotation;
return self();
}
@Override
public T build() {
return parent;
}
@Override
protected DataLabels self() {
return this;
}
public Alignment getAlign() {
return align;
}
public Boolean getAllowOverlap() {
return allowOverlap;
}
public Boolean getCrop() {
return crop;
}
public String getColor() {
return color;
}
public Boolean getDefer() {
return defer;
}
public Boolean getEnabled() {
return enabled;
}
public String getFormat() {
return format;
}
public Boolean getInside() {
return inside;
}
public String getOverflow() {
return overflow;
}
public Number getPadding() {
return padding;
}
public Number getRotation() {
return rotation;
}
public Function getFormatter() {
return formatter;
}
public Boolean getReserveSpace() {
return reserveSpace;
}
}